How to change font of UIButton with Swift
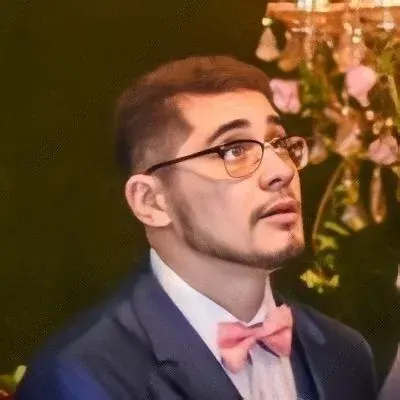
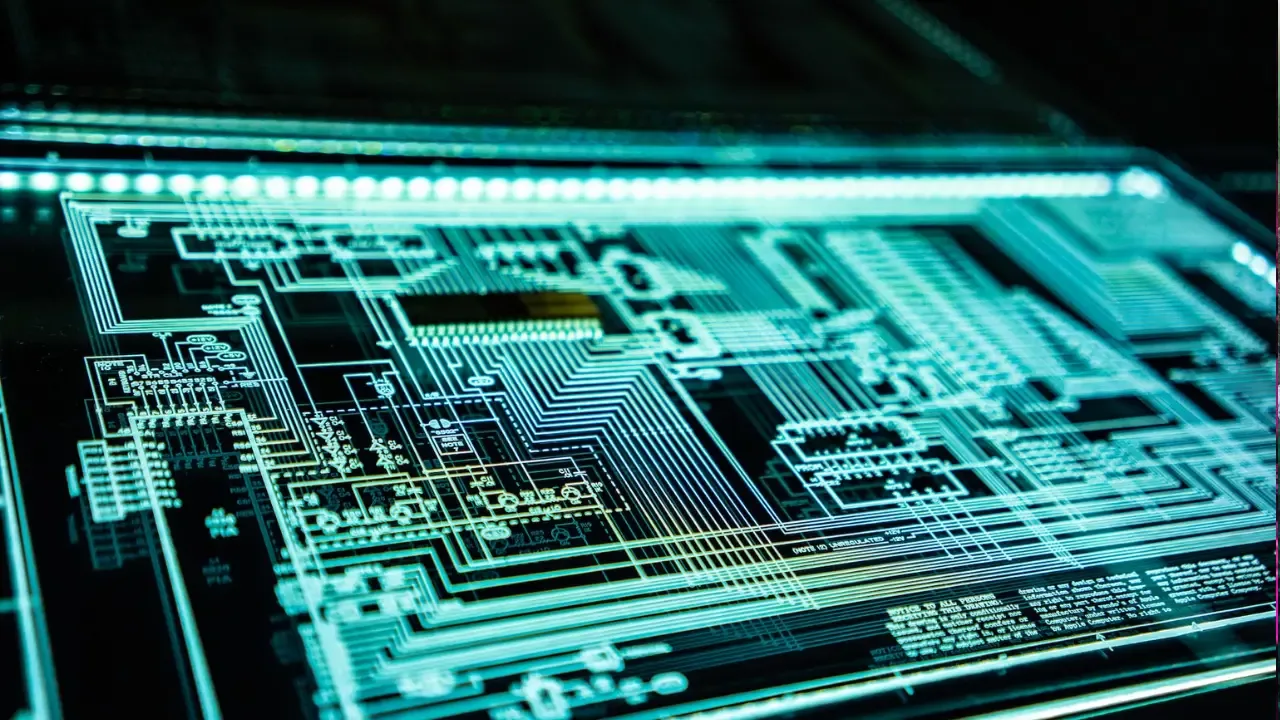
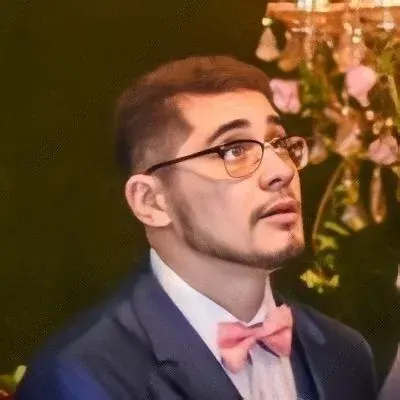
How to Change the Font of a UIButton with Swift 😎
So, you want to change the font of a UIButton using Swift, huh? Well, have no fear because I'm here to help you out! 💪🔥
You might have tried using the .font
property, but unfortunately, it's deprecated now. But don't worry, I've got a couple of easy solutions for you to try out. Let's dive right in! 🏊♂️
Solution 1: Attributed Text 📝
One way to change the font of a UIButton is by using attributed text. It allows you to customize the appearance of specific parts of a text string, including the font. Here's how you can do it:
let attrs: [NSAttributedString.Key: Any] = [
.font: UIFont(name: "YourFontName", size: 10)! // Replace "YourFontName" with the desired font name
]
let attributedString = NSAttributedString(string: "Your Button Text", attributes: attrs)
yourButton.setAttributedTitle(attributedString, for: .normal)
By using the setAttributedTitle(_:for:)
method, you can set an attributed string as the title for your UIButton and apply the desired font. 🖊️
Solution 2: Custom Button Subclass 🎨
Another way to change the font is by subclassing UIButton and creating your custom button class. This approach gives you more flexibility and reusability. Here's how you can do it:
class CustomButton: UIButton {
override func awakeFromNib() {
super.awakeFromNib()
titleLabel?.font = UIFont(name: "YourFontName", size: 10)! // Replace "YourFontName" with the desired font name
}
}
After creating your custom button class, assign it to your UIButton either programmatically or through Interface Builder. Your button will now use the specified font!
📣 Call-to-Action: Share Your Experience!
I hope these solutions help you change the font of your UIButton easily. Try them out and let me know which one worked best for you! Have you faced any other challenges while working with UIButton and fonts? Share your experiences and solutions in the comments below to help fellow developers. Let's learn together! 🤝💡
Stay tuned for more exciting tech tutorials, tips, and tricks on our blog. Until then, happy coding! 💻🎉