How to call gesture tap on UIView programmatically in swift
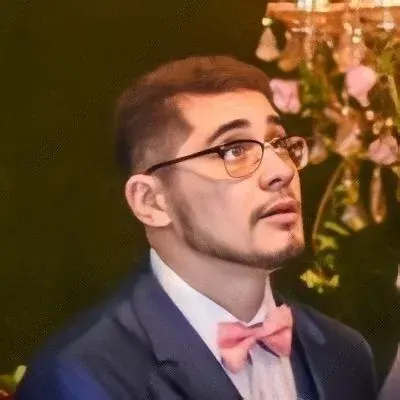
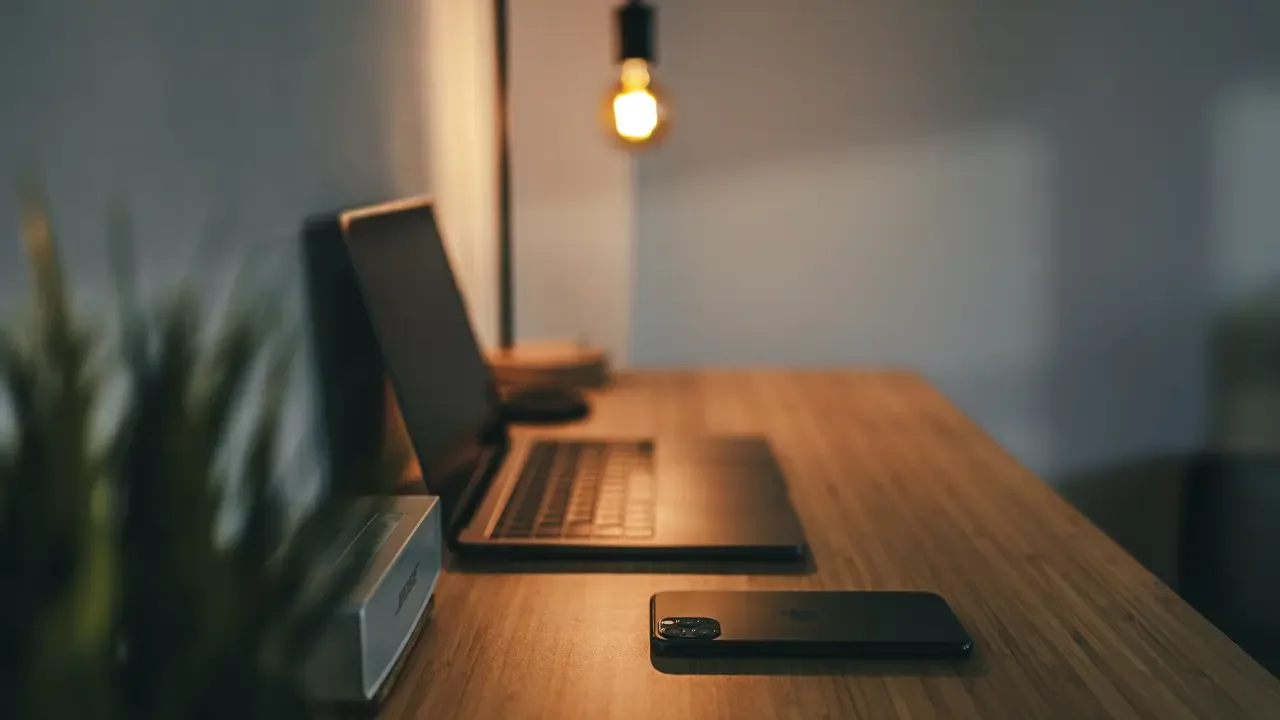
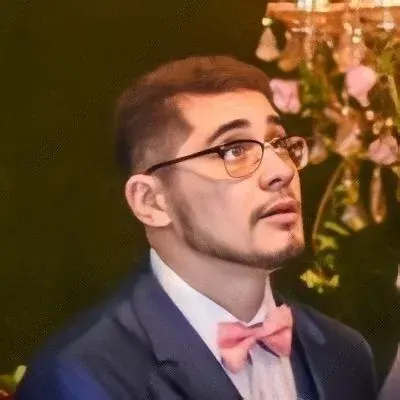
How to Call Gesture Tap on UIView Programmatically in Swift 😎👋
So, you've added a tap gesture to your UIView and now you want to call it programmatically? Awesome! In this post, I'll guide you through the common issues you may face and provide easy solutions. Let's dive in! 🏊♀️
The Initial Setup 🛠️
Let's quickly review the initial setup where you've added a tap gesture to your UIView:
let tap = UITapGestureRecognizer(target: self, action: Selector("handleTap:"))
tap.delegate = self
myView.addGesture(tap)
You're all set! Now, let's see how you can call this gesture programmatically. 📞
The Unsuccessful Attempts 🚫
As mentioned, the following code snippet didn't work for you:
myView.sendActionForEvent(UIEvents.touchUpDown)
And you're seeing an "unrecognized selector sent to instance" error. 😕
The Solution 🎉
The sendActionForEvent
is not suitable for calling a tap gesture. Instead, you can use the perform
method of the gesture recognizer. Here's the updated code:
tap.perform()
That's it! 🎊 You should now be able to call your tap gesture programmatically. Easy, right? 😉
A Word of Caution ⚠️
Keep in mind that calling a tap gesture programmatically is useful in certain scenarios, but it's important to consider the user experience. Users are accustomed to tapping on UI elements, and triggering gestures programmatically might not provide the same intuitive experience. So, use this feature judiciously.
Conclusion 🌟
You've learned how to call a tap gesture on a UIView programmatically in Swift. By using the perform
method of the gesture recognizer, you can easily trigger the tap gesture with just a single line of code. Remember to be mindful of the user experience when using this feature. Now, go ahead and spice up your app with some awesome programmatically triggered tap gestures! 💃
Have you encountered any other issues related to tap gestures in Swift? Share your thoughts, experiences, and questions in the comments below! Let's tap into the conversation! 👇