How to Apply Gradient to background view of iOS Swift App
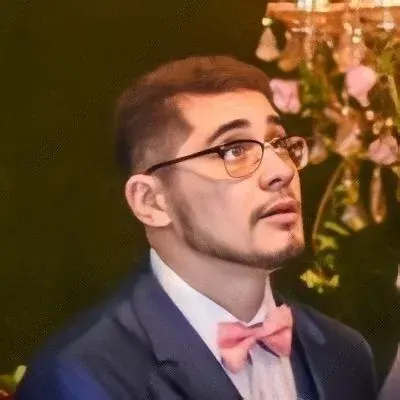
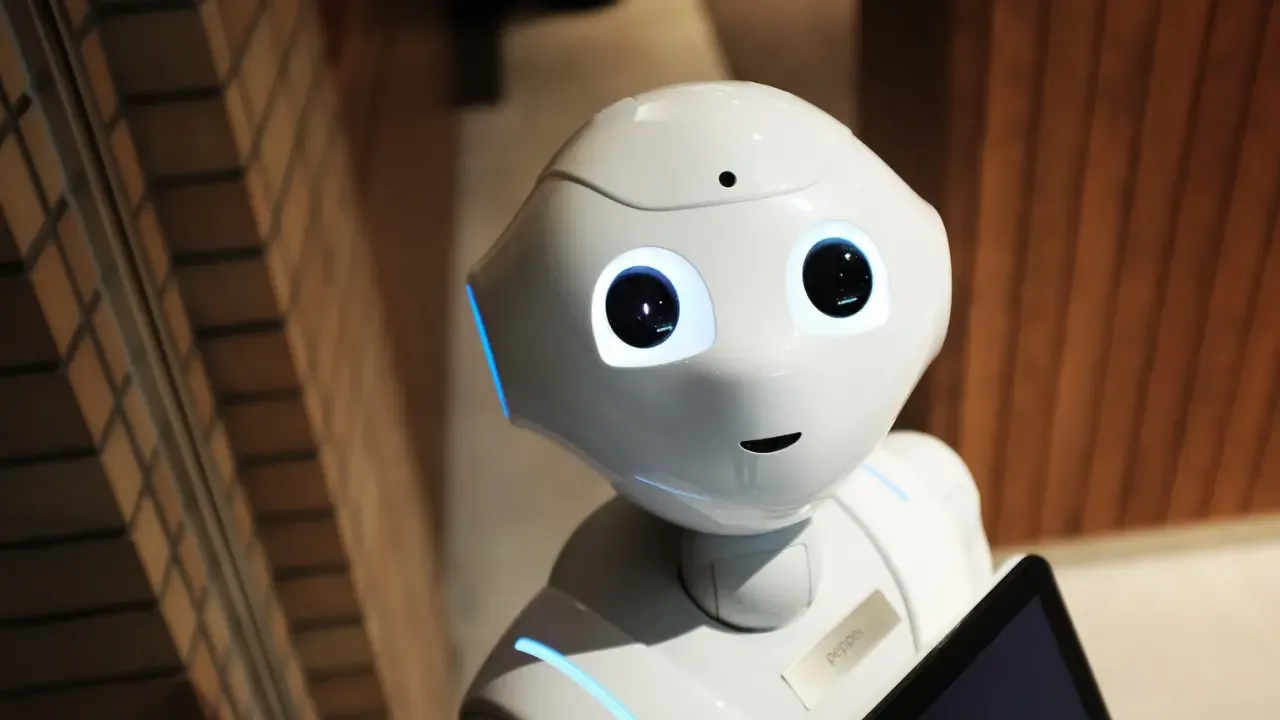
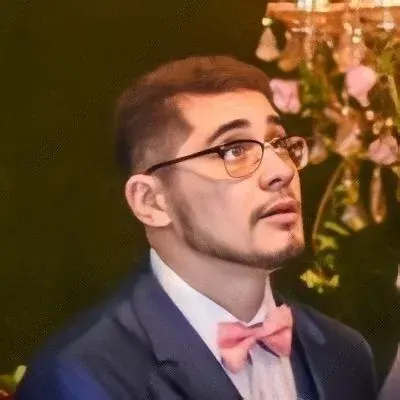
📝📱🌈How to Apply Gradient to Background View of iOS Swift App🌈📱📝
Are you trying to add a gradient as the background color of a view in your iOS Swift app, but nothing seems to change? Don't worry, you're not alone! Many developers face this issue, especially when using newer versions of Xcode and Swift. Luckily, there's an easy solution, and we're here to guide you through it!
Before we dive into the solution, let's take a look at the code provided:
class Colors {
let colorTop = UIColor(red: 192.0/255.0, green: 38.0/255.0, blue: 42.0/255.0, alpha: 1.0)
let colorBottom = UIColor(red: 35.0/255.0, green: 2.0/255.0, blue: 2.0/255.0, alpha: 1.0)
let gl: CAGradientLayer
init() {
gl = CAGradientLayer()
gl.colors = [colorTop, colorBottom]
gl.locations = [0.0, 1.0]
}
}
let colors = Colors()
func refresh() {
view.backgroundColor = UIColor.clearColor()
let backgroundLayer = colors.gl
backgroundLayer.frame = view.frame
view.layer.insertSublayer(backgroundLayer, atIndex: 0)
}
Now, let's break it down and address the issue step by step:
1️⃣ The Colors
class:
The Colors
class is used to define the gradient colors. In the provided code, colorTop
and colorBottom
represent the top and bottom colors of the gradient, respectively. These values are defined using the UIColor
class, with RGB values divided by 255.0.
2️⃣ The gl
property:
The gl
property of type CAGradientLayer
is responsible for creating the gradient layer. It is initialized and configured in the init
method of the Colors
class. The colors
property of the gl
object is set to an array consisting of colorTop
and colorBottom
, representing the gradient colors. The locations
property defines the position of the gradient colors, with 0.0 representing the top and 1.0 representing the bottom.
3️⃣ The refresh()
function:
This function is called to refresh the view and apply the gradient background. It first sets the backgroundColor
of the view to clearColor()
to make it transparent. Then, it creates a new variable backgroundLayer
using the gl
property of the Colors
class. This layer is then sized to match the view's frame and finally inserted as a sublayer at index 0 of the view's layer.
Now that we understand the code, let's get to the solution! 🎉
To make the gradient background appear correctly, we need to ensure that the code is executed at the right time. One common mistake is placing the refresh()
function in the wrong place, resulting in the gradient not being applied.
To solve this issue, follow these steps:
1️⃣ Open your view controller file.
2️⃣ Locate the viewDidLoad()
function.
3️⃣ Inside the viewDidLoad()
function, add a call to the refresh()
function. The updated code should look like this:
override func viewDidLoad() {
super.viewDidLoad()
refresh()
}
By calling the refresh()
function inside viewDidLoad()
, we ensure that the gradient background is applied when the view controller is initially loaded.
That's it! 🎉 Your gradient background should now be applied successfully to the view of your iOS Swift app!
If you're still facing any issues or have any questions, feel free to leave a comment below. We're here to help! 💪
🔗 Subscribe to our newsletter to receive more iOS development tips and tricks delivered straight to your inbox. Stay updated with the latest trends and become a Swift pro! 🚀
Keep coding and keep rocking! 🤘💻