How to add spacing between UITableViewCell
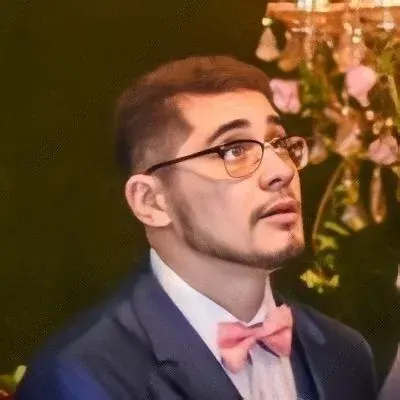
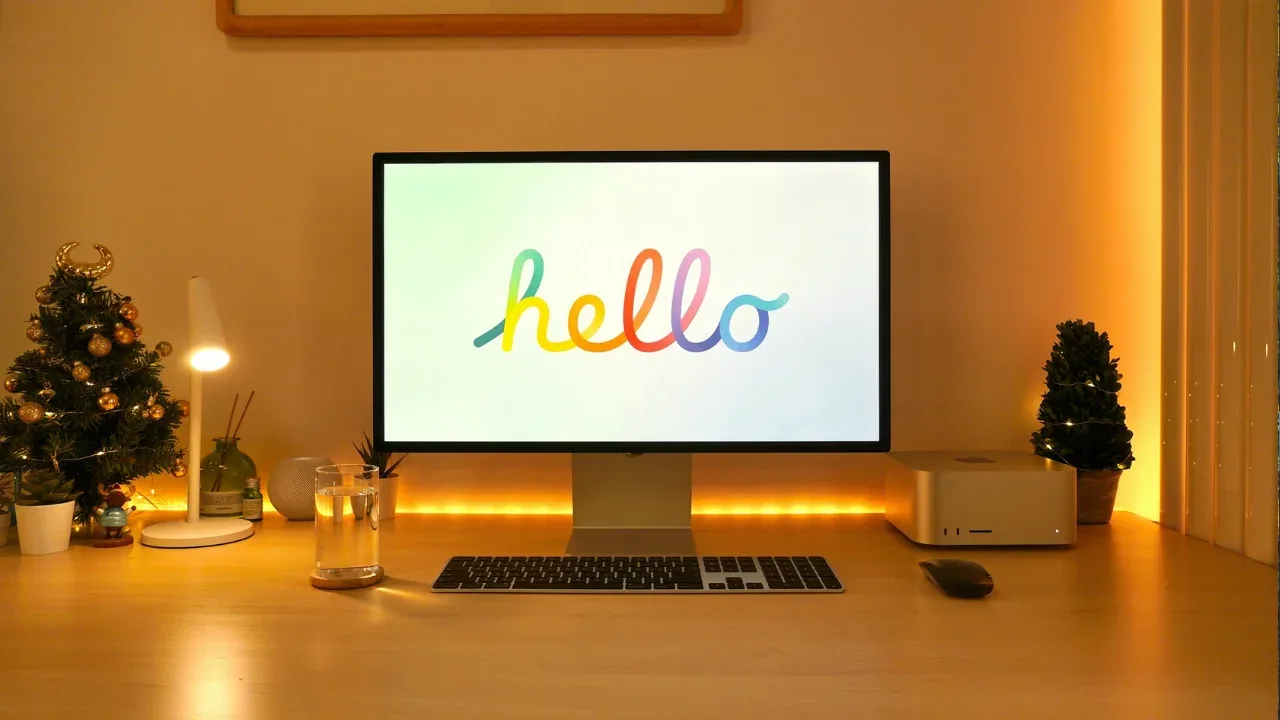
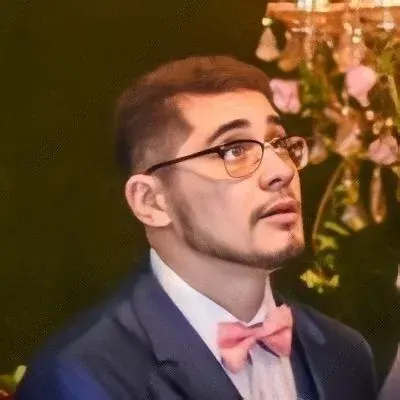
How to Add Spacing Between UITableViewCell
Do you want to add some breathing space between the cells in your UITableView
? Look no further! In this guide, we'll address the common issue of how to add spacing between UITableViewCell
and provide you with easy solutions.
The Problem
Imagine you have a table with cells that each contain an image. You assign the image to the cell using the following code:
cell.imageView.image = [myImages objectAtIndex:indexPath.row];
The problem is that this code causes the image to be enlarged and fit into the whole cell without any spacing between the images.
The Solution
There are a couple of approaches you can take to add spacing between the UITableViewCell
. Let's explore two possible solutions:
Solution 1: Adjusting the Image Size and Adding Padding
One way to achieve the desired spacing is to resize the image and add padding. Here's how you can do it:
// Resize the image
UIImage *resizedImage = [self resizeImage:[myImages objectAtIndex:indexPath.row] withSize:CGSizeMake(cell.frame.size.width, cell.frame.size.height - 20)];
// Add padding
UIImageView *paddedImageView = [[UIImageView alloc] initWithFrame:CGRectMake(0, 10, cell.frame.size.width, cell.frame.size.height - 20)];
paddedImageView.contentMode = UIViewContentModeScaleAspectFit;
paddedImageView.image = resizedImage;
// Add the padded image view to the cell
[cell.contentView addSubview:paddedImageView];
In this solution, we first resize the image to fit the cell's dimensions. Then, we create a new UIImageView
with added padding and set its content mode to UIViewContentModeScaleAspectFit
. Finally, we add the padded image view to the cell's contentView
.
Solution 2: Implementing Custom Spacing Using Auto Layout
Another approach is to use Auto Layout to create custom spacing between the cells. Follow these steps to achieve the desired result:
Add a new
UIImageView
as a subview of the cell'scontentView
.Pin the image view's leading, trailing, top, and bottom constraints to the cell's
contentView
.Set the image view's content mode to
UIViewContentModeScaleAspectFit
.Specify the desired spacing by adding a height constraint to the image view. For example, if you want 20 points of spacing, set the height constraint's constant to
imageHeight + 20
.
Here's an example implementation in code:
let padding: CGFloat = 20
let imageView = UIImageView()
imageView.contentMode = .scaleAspectFit
imageView.translatesAutoresizingMaskIntoConstraints = false
cell.contentView.addSubview(imageView)
// Add constraints
imageView.leadingAnchor.constraint(equalTo: cell.contentView.leadingAnchor).isActive = true
imageView.trailingAnchor.constraint(equalTo: cell.contentView.trailingAnchor).isActive = true
imageView.topAnchor.constraint(equalTo: cell.contentView.topAnchor).isActive = true
imageView.bottomAnchor.constraint(equalTo: cell.contentView.bottomAnchor).isActive = true
imageView.heightAnchor.constraint(equalToConstant: imageHeight + padding).isActive = true
By following these steps, you can customize the spacing between the cells to your liking.
Your Turn
There you have it – two easy solutions to add spacing between UITableViewCell
. Now it's your turn to try them out and see which approach works best for you. Don't hesitate to experiment and tweak the code to match your specific requirements.
Have any questions or other solutions to share? Let us know in the comments below! 🚀