How to add constraints programmatically using Swift
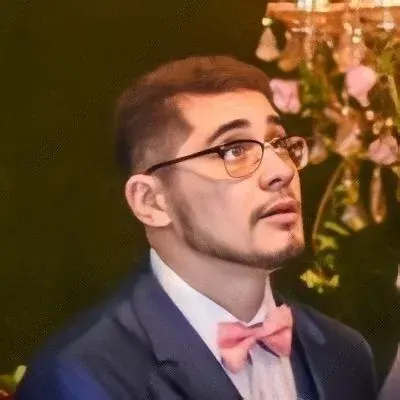
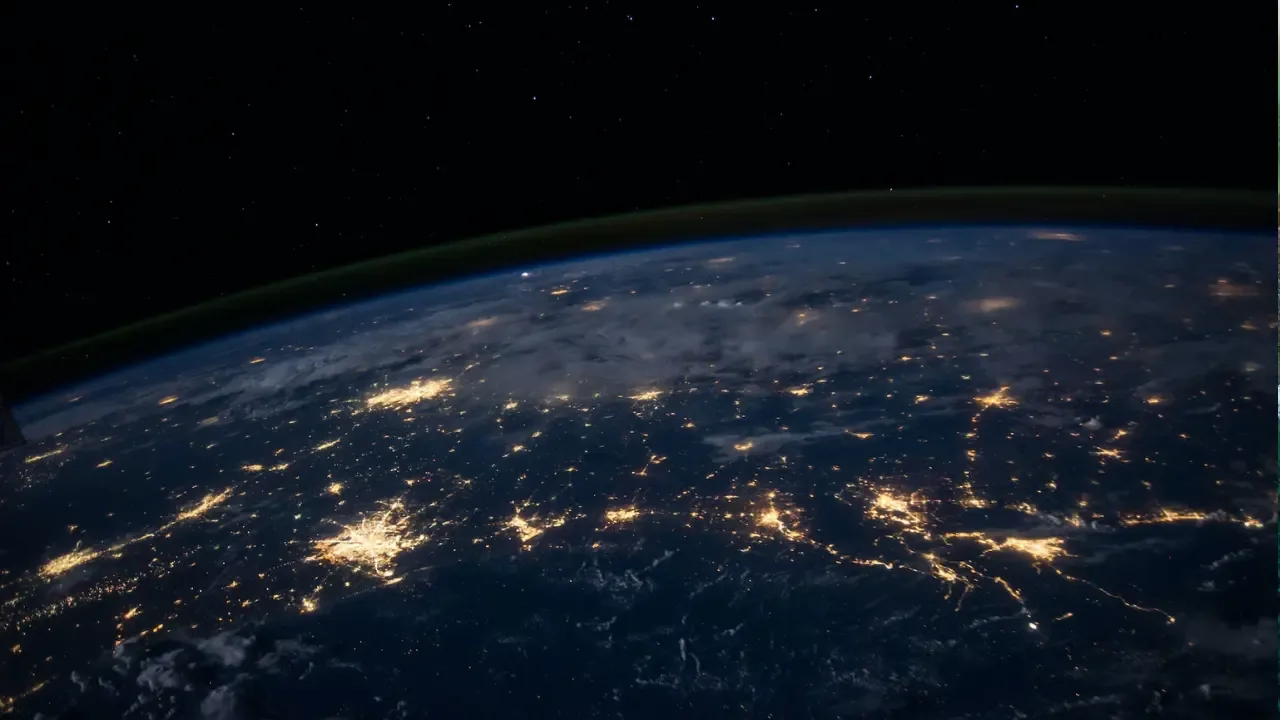
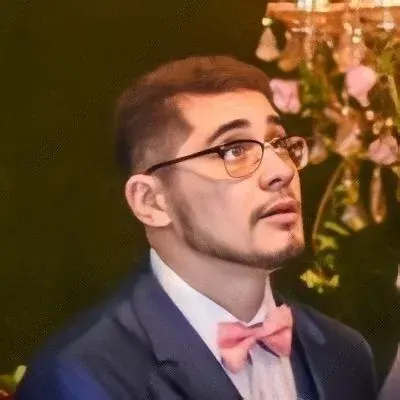
How to Add Constraints Programmatically using Swift
š This week, we're diving into the world of constraints in Swift! š
If you've been scratching your head š¤ trying to figure out how to add constraints programmatically to a UIView using Swift, fear not! We've got you covered. šŖ
Let's take a look at the code snippet you posted:
var new_view:UIView! = UIView(frame: CGRectMake(0, 0, 100, 100))
new_view.backgroundColor = UIColor.redColor()
view.addSubview(new_view)
var constX:NSLayoutConstraint = NSLayoutConstraint(item: new_view, attribute: NSLayoutAttribute.CenterX, relatedBy: NSLayoutRelation.Equal, toItem: self.view, attribute: NSLayoutAttribute.CenterX, multiplier: 1, constant: 0)
self.view.addConstraint(constX)
var constY:NSLayoutConstraint = NSLayoutConstraint(item: new_view, attribute: NSLayoutAttribute.CenterY, relatedBy: NSLayoutRelation.Equal, toItem: self.view, attribute: NSLayoutAttribute.CenterY, multiplier: 1, constant: 0)
self.view.addConstraint(constY)
var constW:NSLayoutConstraint = NSLayoutConstraint(item: new_view, attribute: NSLayoutAttribute.Width, relatedBy: NSLayoutRelation.Equal, toItem: new_view, attribute: NSLayoutAttribute.Width, multiplier: 1, constant: 0)
self.view.addConstraint(constW)
var constH:NSLayoutConstraint = NSLayoutConstraint(item: new_view, attribute: NSLayoutAttribute.Height, relatedBy: NSLayoutRelation.Equal, toItem: new_view, attribute: NSLayoutAttribute.Height, multiplier: 1, constant: 0)
self.view.addConstraint(constH)
š The code you provided looks correct at first glance, but it seems that Xcode is throwing some constraint-related errors. š«
The error message you received from Xcode says:
Unable to simultaneously satisfy constraints. Probably at least one of the constraints in the following list is one you don't want...
š Let's break this down and address the issue step by step:
1ļøā£ Take a closer look at the constraints: In the error message, Xcode provided a list of constraints with hexadecimal identifiers. These identifiers can be used to identify the conflicting constraints.
2ļøā£ Check for unwanted constraints: From the list of constraints Xcode provided, try to identify any constraints that you don't expect or didn't add explicitly in your code. These unwanted constraints might be causing conflicts.
3ļøā£ Inspect the constraint creation code: Look for the part of your code where the unwanted constraint or constraints are being added. Make sure you identify the specific line where the constraint is being added and fix it accordingly.
4ļøā£ Symbolic breakpoint to catch the issue: Xcode suggests making a symbolic breakpoint at UIViewAlertForUnsatisfiableConstraints
to catch the constraint issue in the debugger. This breakpoint will help you identify the point in your code where the unsatisfiable constraint is being added.
5ļøā£ Review the documentation: If you encounter NSAutoresizingMaskLayoutConstraints that you don't understand, refer to the documentation for the UIView property translatesAutoresizingMaskIntoConstraints
.
š” Remember that adding constraints programmatically can be tricky, and sometimes it involves trial and error. Don't hesitate to experiment with different constraints and tweak their values to achieve the desired layout.
š So, give it a try! Fix those unwanted constraints and see if it resolves the issue in Xcode.
Got any more questions? Feel free to ask in the comments below! š
š Don't forget to share this blog post with your fellow Swift developers who might be struggling with adding constraints programmatically. Sharing is caring! Let's help everyone build beautiful layouts in Swift together! š