How does one declare optional methods in a Swift protocol?
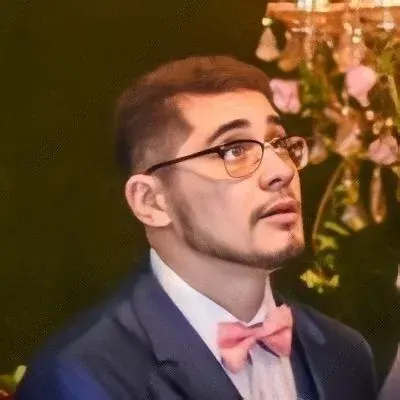
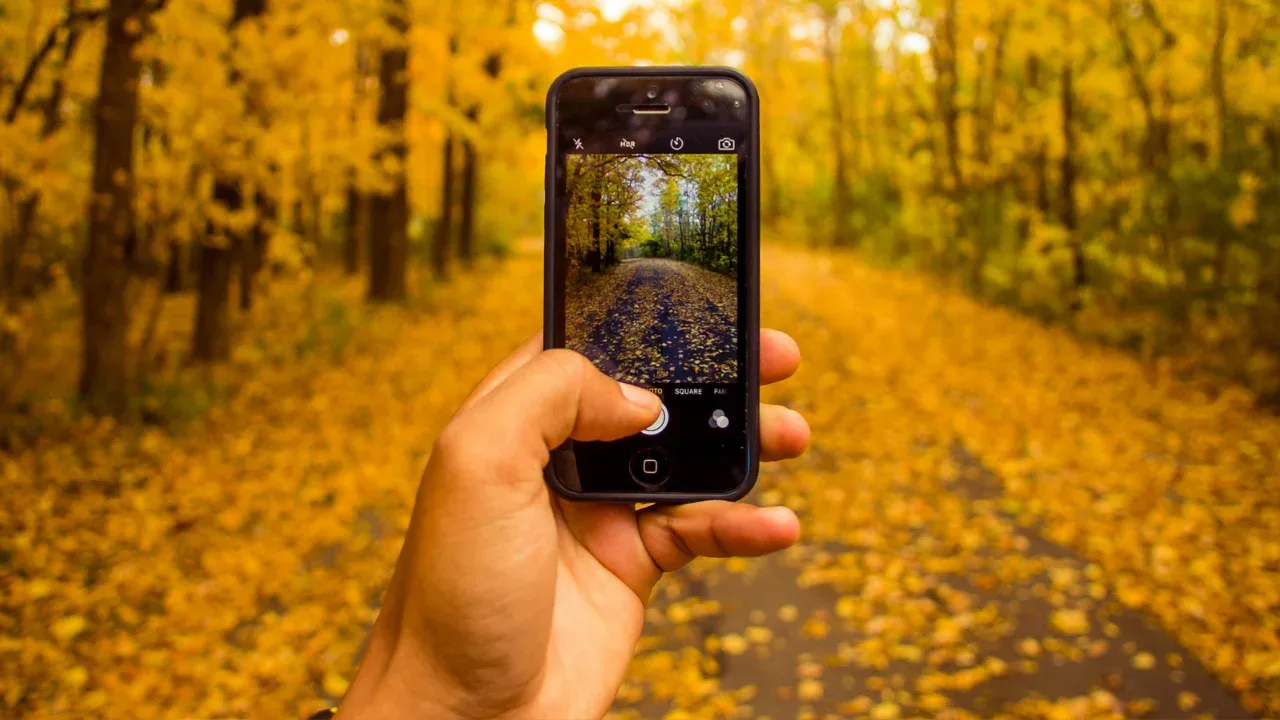
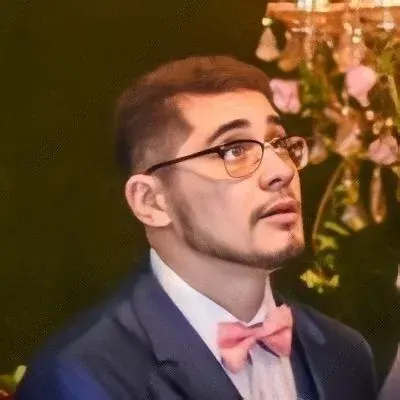
📝 Blog Post: Declaring Optional Methods in Swift Protocols
Hey there, fellow Swift enthusiast! 👋 Are you scratching your head, wondering how to declare optional methods within a Swift protocol? You're not alone! 🤔 Many Swift developers have been pondering this very question. But fret not, my friend, for I have the answers you seek! 🎉
The Scenario: Optional Methods in Swift Protocols
So, you've created a protocol in Swift, and you want some of its methods to be optional. But wait a minute, declaring optional methods is not as straightforward in Swift as it is in some other programming languages. 😓
The Problem: No Built-in Direct Solution
If you're wondering whether Swift has a native way to declare optional methods in a protocol, the straight answer is no, it doesn't. 😕 Unlike Objective-C, which allows you to easily declare optional methods using the @optional
directive, Swift doesn't provide a similar feature out of the box.
The Solution: Optional Method Requirements
Although Swift doesn't offer an explicit "optional" keyword for protocol methods, it provides an elegant workaround called "optional method requirements". These allow you to define methods that conforming types can decide whether or not to implement. 🙌
Here's how you can declare an optional method requirement:
@objc protocol MyProtocol {
@objc optional func optionalMethod()
}
Note the @objc
attribute. Since optional method requirements are a feature inherited from Objective-C, you need to mark your protocol as @objc
for these optional methods to work.
Using Optional Methods
To conform to a protocol with an optional method requirement, you can simply choose whether or not to implement the optional method in your conforming type. If you decide to implement it, you must follow some rules to ensure both the caller and the compiler are happy:
The conforming type must also be marked as
@objc
since it needs to take advantage of Objective-C runtime dispatching.Optional methods defined in protocols are always implicitly optional in the conforming type, so no additional keyword is needed for implementation.
To invoke an optional method, you first have to check if the conforming instance implements it, using the
responds(to:)
method.
Here's an example:
@objc protocol MyProtocol {
@objc optional func optionalMethod()
}
class MyClass: NSObject, MyProtocol {
func callOptionalMethod() {
if self.responds(to: #selector(optionalMethod)) {
optionalMethod?()
}
}
}
let obj = MyClass()
obj.callOptionalMethod() // Calls the optional method if implemented
Easy peasy, right? 😀 By following these steps, you can incorporate optional methods within your Swift protocols.
So, What's the Verdict?
While Swift doesn't have a built-in keyword for declaring optional methods in protocols, the optional method requirements offer a neat workaround. Remember to mark your protocol and conforming types as @objc
, and utilize the responds(to:)
method to safely call the optional methods.
Now, with this newfound knowledge, go ahead and make your Swift protocols even more flexible and powerful! ✨💪
If you found this blog post helpful, let me know in the comments below. Have you encountered any other Swift conundrums? Share your thoughts and questions, and let's tackle them together! 💬🚀