How do you find out the type of an object (in Swift)?
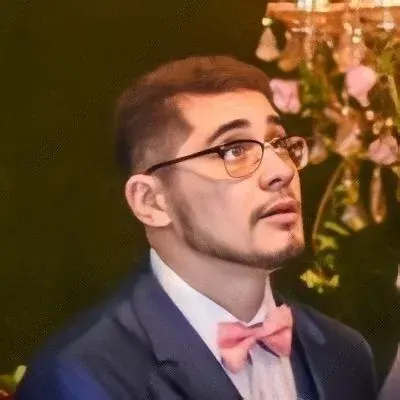
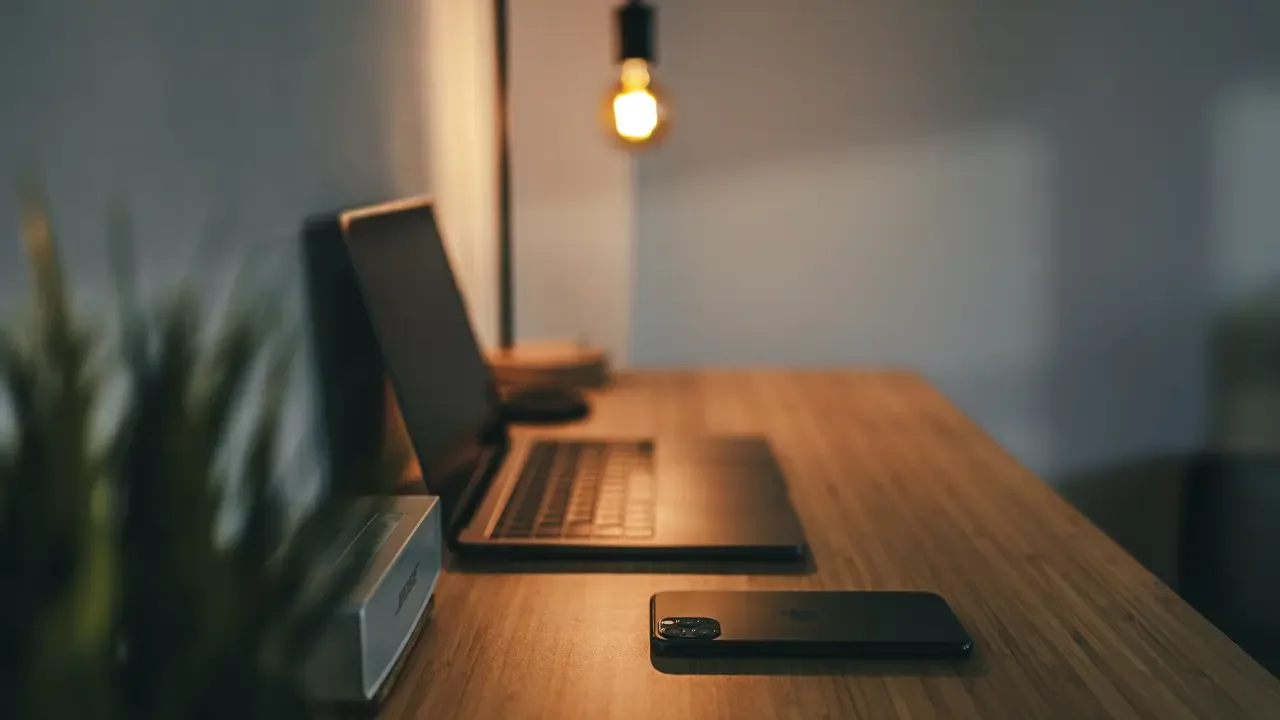
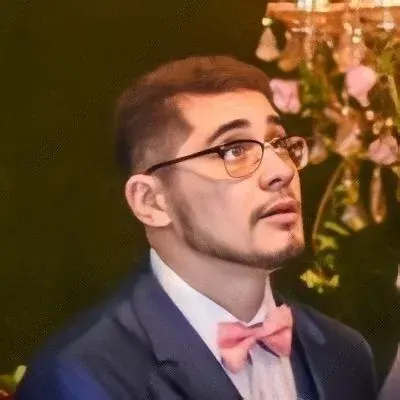
How to Find Out the Type of an Object in Swift 🕵️♂️
Have you ever found yourself scratching your head, wondering how to figure out the type of an object in Swift? 🤔 Don't worry, you're not alone! In this guide, we'll explore different techniques to help you tackle this common issue. So let's dive in and unlock the secrets of object type discovery! 💪
The dynamicType Property 👀
One option that might come to mind is using the dynamicType
property. However, it's important to note that in recent versions of Swift, accessing dynamicType
gives you a reference to the type, not the instance of the dynamic type. This means that it won't provide you with the concrete type information you're looking for. 😕
Here's an example:
class MyClass {
var count = 0
}
let mc = MyClass()
// Update: This now evaluates as true
mc.dynamicType === MyClass.self
Even though mc.dynamicType === MyClass.self
might seem promising, it still doesn't give you the actual type of the object. So let's explore other techniques! 💡
Using Class References 📚
Another approach you can take is using class references to instantiate a new object. This technique might give you the type information you desire. However, you might encounter a hurdle along the way. When you use a class reference to create a new object, you might receive an error message stating that you must add a required
initializer.
Let's take a look at an example that demonstrates this:
class MyClass {
var count = 0
required init() {
}
}
let myClass2 = MyClass.self
let mc2 = MyClass2()
In this case, MyClass2()
is successfully instantiated using MyClass.self
, which indicates that you can obtain the type information. However, it does introduce some additional complexity with required
initializers. 😕
Seeking Further Solutions 🚀
Although the above techniques can get you closer to discovering the type of an object, they might not fully meet your needs. But fear not! Swift provides more powerful solutions to this problem.
Here are a few alternatives worth exploring:
1. The type(of:)
Function 📚
Swift offers the type(of:)
function, which allows you to determine the type of an object without any extra hassle.
Here's an example of how you can use it:
class MyClass {
var count = 0
}
let mc = MyClass()
let type = type(of: mc)
print(type) // Output: MyClass
By utilizing type(of: mc)
, you can easily retrieve the type information you're looking for. 🎉
2. The Mirror
Structure 🔍
Another powerful tool at your disposal is the Mirror
structure. It enables you to inspect the structure and properties of any object, including its type.
Consider the following example:
class MyClass {
var count = 0
}
let mc = MyClass()
let mirror = Mirror(reflecting: mc)
let objectType = mirror.subjectType
print(objectType) // Output: MyClass
Using Mirror
, you can obtain the desired type information effortlessly. 🕵️♀️
Remember, these are just a couple of techniques to get you started on your journey to discover an object's type in Swift. Further exploration and experimentation are encouraged! 🔬
Engage with the Community! 💬
We hope this guide has provided you with essential insights on finding out the type of an object in Swift. Were you aware of these techniques? Do you have any other novel solutions that we haven't mentioned? Share your thoughts and experiences in the comments below! Let's learn from each other and build a vibrant Swift community together! 🌟