How do you add multi-line text to a UIButton?
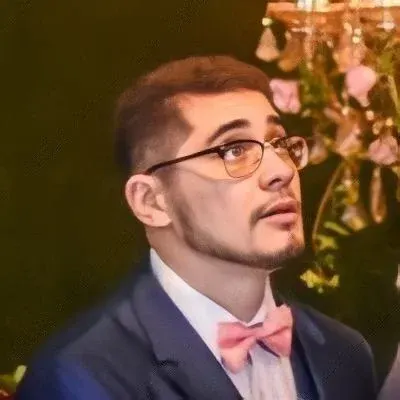
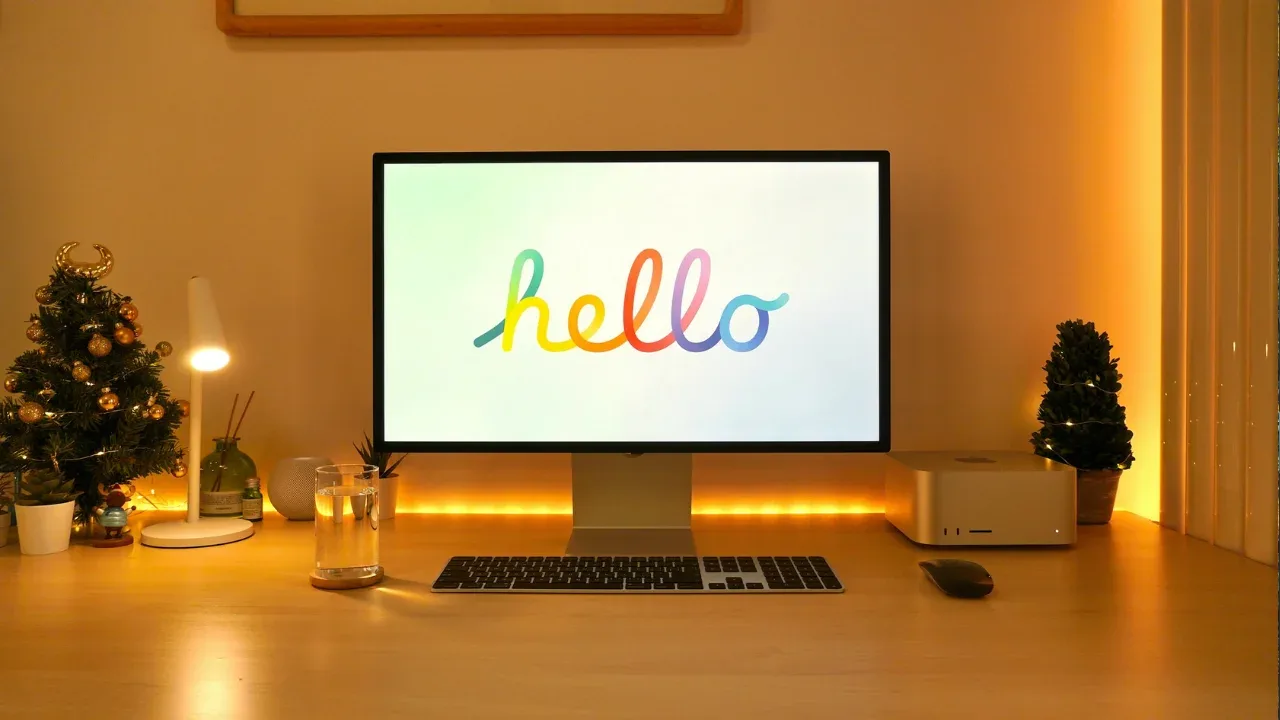
How to Add Multi-Line Text to a UIButton: A Complete Guide
š„ Hey there tech enthusiasts! Are you facing trouble adding multi-line text to a UIButton? š¤ Don't worry, we've got you covered! In this guide, we will walk you through common issues you might encounter and provide easy solutions to spice up those buttons with eye-catching multi-line text. šŖ
The Problem:
One common solution is to add a UILabel as a subview to the UIButton and set the desired text. But, what if the text is always obscured by the backgroundImage of the UIButton? š¤·āāļø
Take a look at the code snippet provided by a fellow developer:
UILabel *buttonLabel = [[UILabel alloc] initWithFrame:targetButton.bounds];
buttonLabel.text = @"Long text string";
[targetButton addSubview:buttonLabel];
[targetButton bringSubviewToFront:buttonLabel];
The developer is adding a UILabel as a subview to the UIButton and assigns it some meaningful text. However, the text itself cannot be seen. š So, is this a bug in UIButton or is the developer doing something wrong? Let's find out!
The Solution:
The problem lies in the way a UIButton handles the background image. By default, the background image's content mode is set to "Scale To Fill", which causes it to occupy the entire button's bounds, hiding any subviews, including our UILabel. šµ
But worry not! We have a couple of easy solutions for you:
Solution 1: Adjust the Background Image's Content Mode
The first solution involves modifying the background image's content mode to allow the subviews to be visible. By setting the content mode of the background image to "Center", we can ensure that it doesn't overlap our UILabel.
[targetButton setBackgroundImage:[UIImage imageNamed:@"your_image_name"] forState:UIControlStateNormal];
[targetButton.imageView setContentMode:UIViewContentModeCenter];
With this change, the subviews, including the UILabel, will now be visible on the button.
Solution 2: Customize the UIButton Using NSAttributedString
Another way to achieve multi-line text on a UIButton is by leveraging NSAttributedString
. This approach gives us more flexibility in customizing the appearance of the button's text.
NSString *text = @"Long text string";
NSMutableParagraphStyle *paragraphStyle = [[NSMutableParagraphStyle alloc] init];
paragraphStyle.alignment = NSTextAlignmentCenter;
NSAttributedString *attributedText = [[NSAttributedString alloc] initWithString:text attributes:@{NSParagraphStyleAttributeName: paragraphStyle}];
[targetButton setAttributedTitle:attributedText forState:UIControlStateNormal];
By using NSMutableParagraphStyle
, we can adjust the alignment of the text and create a multi-line effect. Feel free to modify attributes like font, color, and spacing to achieve your desired look!
Time to Rock Your Multi-Line Text Buttons! š
Adding multi-line text to a UIButton is now a piece of cake! š° Whether you choose to adjust the background image's content mode or customize the button using NSAttributedString
, it's time to let your creativity shine and create captivating buttons.
We hope this guide was helpful in solving your problem. Let us know in the comments below if you encountered any other issues or if there are specific topics you'd like us to cover next.
Now go out there, spruce up those buttons, and elevate your user experience! š„š»
Keep calm and keep coding! šš»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
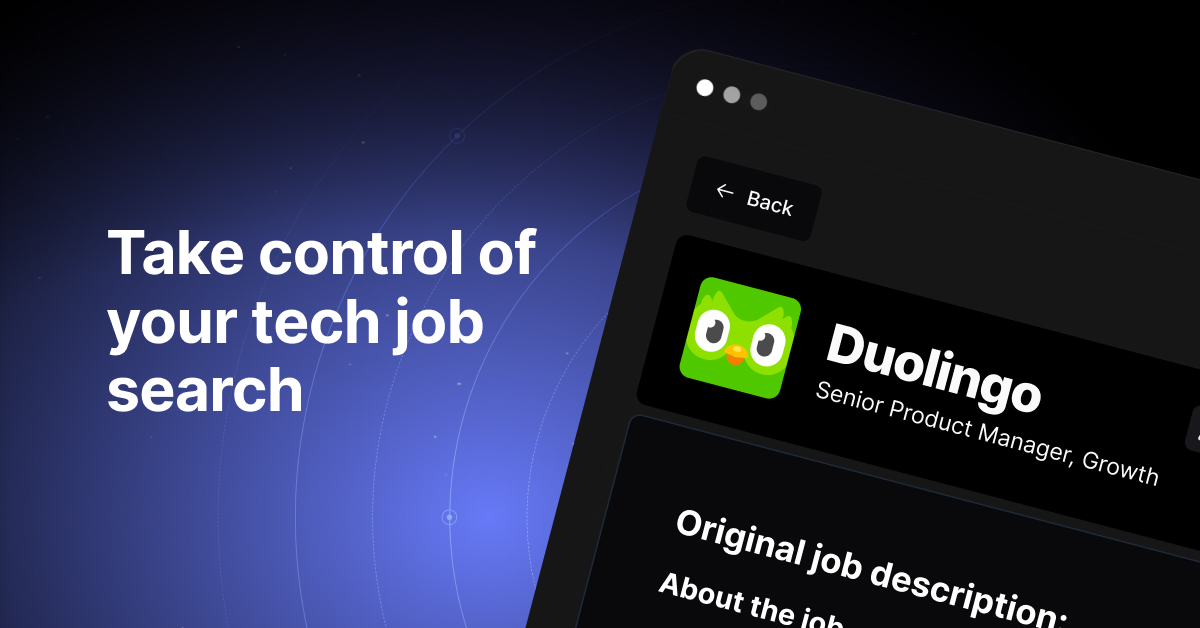