How do you add an in-app purchase to an iOS application?
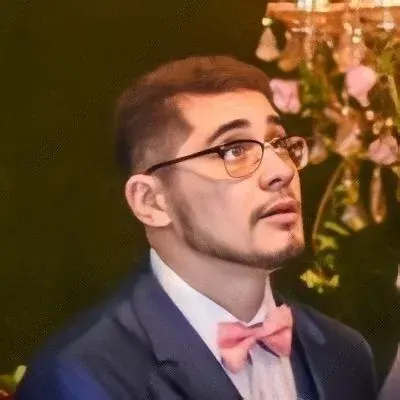
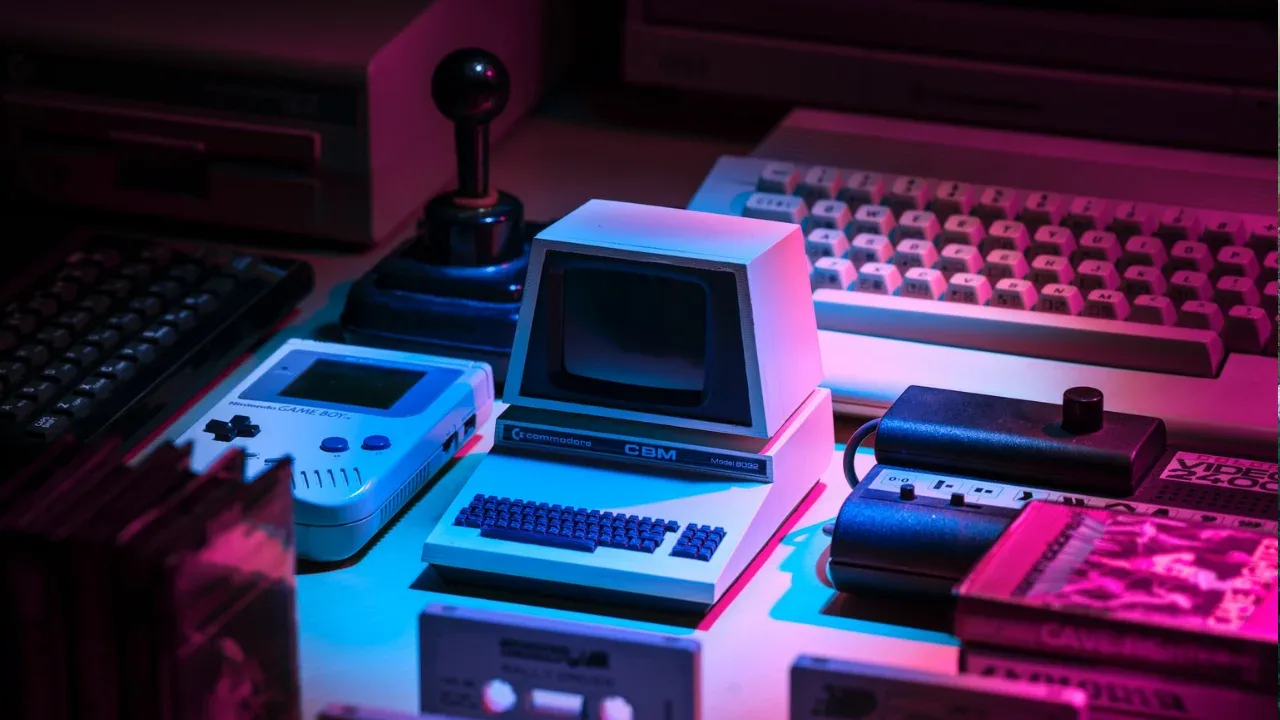
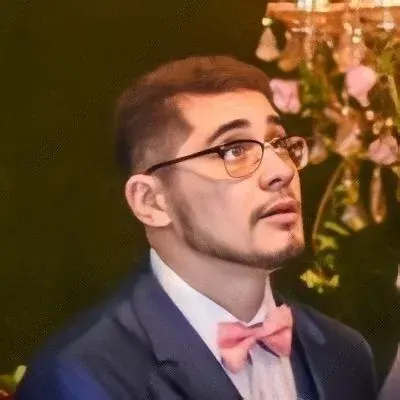
How to Add an In-App Purchase to Your iOS App ๐ฑ๐ฐ
So, you've built an awesome iOS app and now you want to monetize it by adding in-app purchases ๐ค Adding in-app purchases to your app can be a great way to generate revenue and provide additional features or content to your users. In this guide, we'll walk you through the process of adding an in-app purchase to your iOS app step by step.
Step 1: Set Up Your Project ๐๏ธ
To get started, open your Xcode project and navigate to the "Capabilities" tab of your target settings. Enable the "In-App Purchase" capability. This will create an App ID with in-app purchase support for your app.
Step 2: Create In-App Purchase Products ๐ฆ
Next, you'll need to create the in-app purchase products in App Store Connect. Go to App Store Connect, select your app, and navigate to the "In-App Purchases" section. Click the "+" button to add a new in-app purchase product.
You can choose from various types of in-app purchases, such as consumable, non-consumable, auto-renewable subscriptions, and non-renewable subscriptions. Select the type that suits your app and provide the necessary details, including a unique product ID, pricing information, and localized descriptions.
Step 3: Handle the Purchase Process ๐
To handle the purchase process in your app, you'll need to use the StoreKit framework provided by Apple. Start by importing the StoreKit framework into your project.
Implement the StoreKit delegate methods to handle the various stages of the purchase process, such as presenting the in-app purchase store, processing transactions, and providing the purchased content to the user.
Here's an example of how you can implement the purchase process in your app:
import StoreKit
// Implement the SKPaymentTransactionObserver protocol
class MyStoreObserver: NSObject, SKPaymentTransactionObserver {
func paymentQueue(_ queue: SKPaymentQueue, updatedTransactions transactions: [SKPaymentTransaction]) {
for transaction in transactions {
switch transaction.transactionState {
case .purchased:
// Unlock the purchased content for the user
unlockContent()
SKPaymentQueue.default().finishTransaction(transaction)
case .failed:
// Handle the failed transaction
handleFailedTransaction()
SKPaymentQueue.default().finishTransaction(transaction)
case .restored:
// Restore the previously purchased content for the user
restorePurchasedContent()
SKPaymentQueue.default().finishTransaction(transaction)
default:
break
}
}
}
// Other delegate methods...
}
// Add store observer to the payment queue
SKPaymentQueue.default().add(MyStoreObserver())
Step 4: Test Your In-App Purchase ๐งช
Before submitting your app to the App Store, it's crucial to thoroughly test your in-app purchase implementation. You can use sandbox testing accounts provided by Apple to simulate the purchase process without actually charging any real money.
Test all the possible scenarios, such as successful purchases, failed purchases, and restoring purchases. Make sure to handle any errors or edge cases gracefully to provide a smooth user experience.
Step 5: Submit Your App to the App Store ๐
Once you've tested your in-app purchase and are confident that it's working correctly, you're ready to submit your app to the App Store. Make sure to follow Apple's App Review Guidelines and provide accurate and clear information about your in-app purchases during the submission process.
Conclusion ๐
Congratulations! ๐ฅณ You've successfully added in-app purchase functionality to your iOS app. Now it's time to sit back and watch the money roll in ๐ธ Don't forget to continuously monitor the performance of your in-app purchases and iterate on your monetization strategy to maximize your app's revenue potential.
If you found this guide helpful, share it with your fellow app developers and let them know how easy it is to add in-app purchases to their iOS apps. Happy coding! ๐ป๐
*[App Store Connect]: Apple's platform for managing iOS apps on the App Store *[SKPaymentTransactionObserver]: A protocol for observing and handling in-app purchases *[SDK]: Software Development Kit