How do I put the image on the right side of the text in a UIButton?
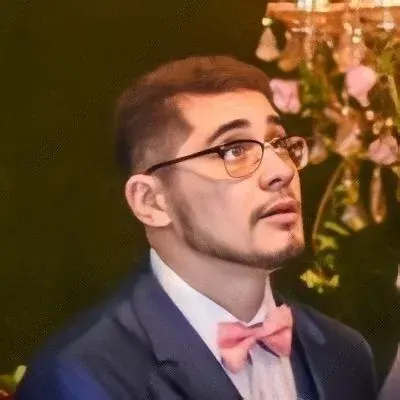
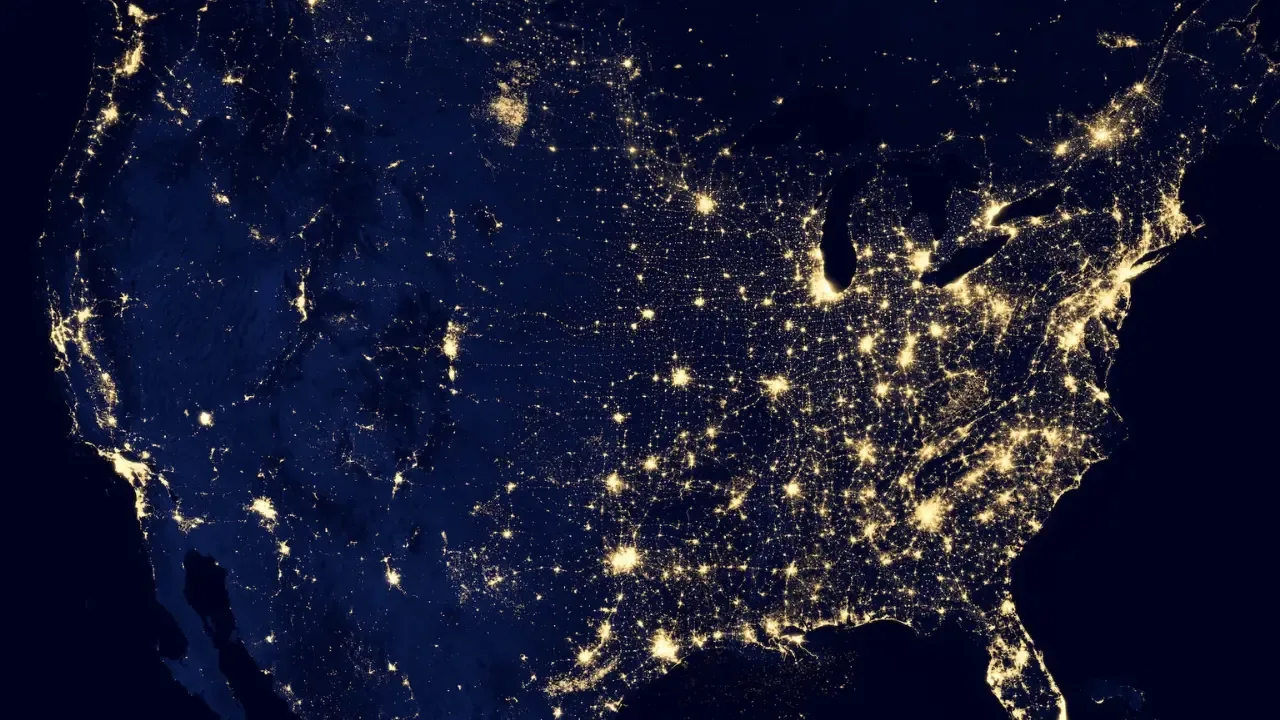
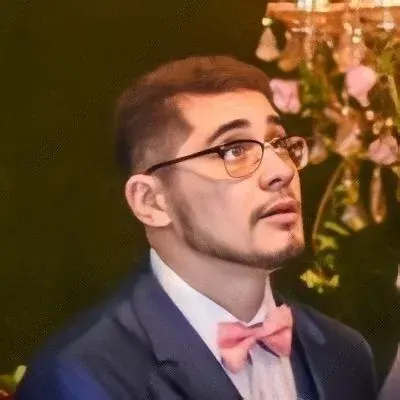
📷 Image on the Right Side of UIButton Text - A Complete Guide 📷
Are you tired of having your image on the left side of the text in your UIButton? Well, fear not! In this guide, we will walk you through the process of putting the image on the right side of the text in a UIButton. 🚀
The Challenge 💪
One of our readers recently asked us how to achieve this without using a subview. They wanted a UIButton with a background image, text, and an image in it. However, by default, the image appears on the left side of the text. 😕
The Solution 🙌
Fortunately, there is a straightforward solution to this problem. Simply follow these steps:
Create a custom UIButton subclass or use the existing UIButton subclass you are using.
Override the
layoutSubviews
method in the subclass.Inside the
layoutSubviews
method, call the superclass's implementation usingsuper.layoutSubviews()
.After calling the superclass, adjust the position of the image and text within the button using the
titleEdgeInsets
andimageEdgeInsets
properties.Specify negative values for the
titleEdgeInsets
to shift the text towards the right side.Specify positive values for the
imageEdgeInsets
to shift the image towards the right side.
Here's an example implementation that puts the image on the right side of the text in a UIButton:
class MyButton: UIButton {
override func layoutSubviews() {
super.layoutSubviews()
// Shift the text towards the right
self.titleEdgeInsets.left = self.bounds.width - self.imageView!.frame.width - self.titleLabel!.frame.width
// Shift the image towards the right
self.imageEdgeInsets.left = self.titleLabel!.frame.width
}
}
By following these steps, you will be able to achieve the desired layout without the need for subviews. 🎉
Common Pitfalls to Avoid 🚧
Keep in mind the following potential issues that you might encounter:
Make sure to set an appropriate background image for your button to avoid any visibility issues.
The
titleEdgeInsets
andimageEdgeInsets
values might need adjustment based on your specific button layout requirements.
That's it! 😎
Now you know how to put the image on the right side of the text in a UIButton without using subviews. We hope this guide has been helpful to you! Feel free to reach out if you have any questions or need further assistance. ✨
Share your Success Story! 🌟
Have you successfully implemented this solution in your project? We would love to hear about it! Share your experience, code snippets, or any other tips and tricks you discovered along the way in the comments below. Let's learn from each other and make our coding journey more exciting! 💡
Happy coding! 👩💻👨💻