How do I convert a Swift Array to a String?
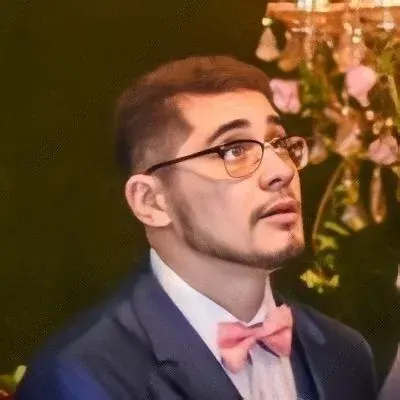
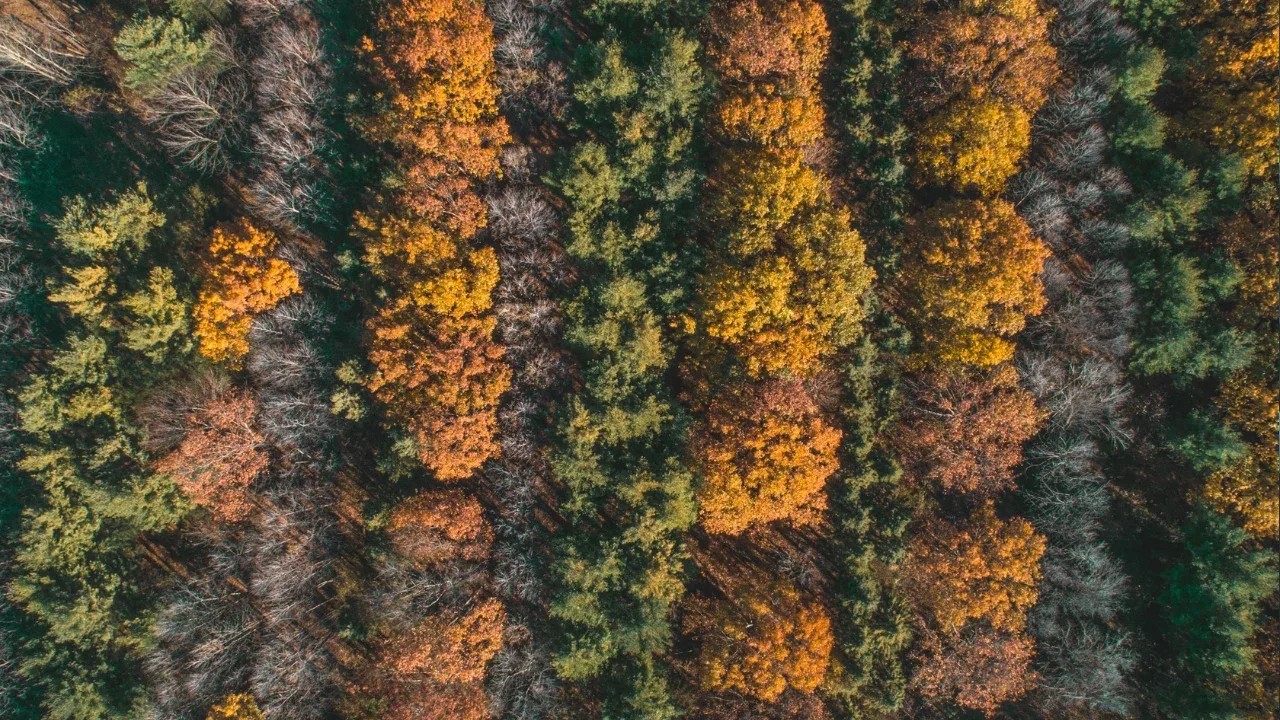
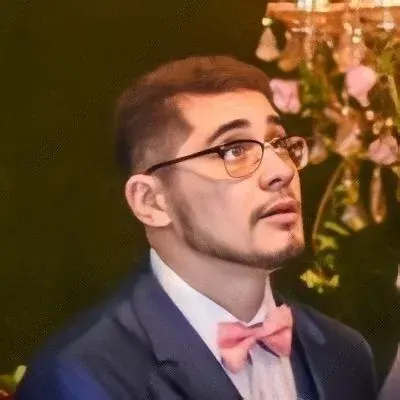
🔤🔄 How to Convert a Swift Array to a String? 💡💻
So, you're wondering if Swift has a handy-dandy built-in method to convert an array into a string? Well, I'm here to tell you that...yes, yes it does! 🎉🎉
Before we dive into the solution, let's take a quick look at the context around this question. Our fellow programmer here knows how to manually convert an array to a string, but they're curious if there's a more convenient way to handle this in Swift. And boy, are they in for a treat! 🍬
When you're working with Swift, you'll be pleased to know that there is a default and straightforward way to convert an array into a string. So, let's buckle up and learn how to do it, shall we? 💪
The Swift Way 🚀
To convert an array to a string in Swift, you can simply use the joined(separator:)
method. This method allows you to specify a separator that will be used to join all the elements of the array into a string. 🪟
Here's a code snippet to illustrate how it works:
let myArray = ["Hello", "World", "!"]
let myString = myArray.joined(separator: ", ")
print(myString) // Output: Hello, World, !
In the example above, we have an array called myArray
containing three elements. By calling the joined(separator:)
method on this array and passing in ", "
as the separator, we obtain a string representation myString
that joins all the elements together with a comma separated by a space. When we print myString
, we get the desired output: "Hello, World, !". 🌍
But wait! There's more! You can use any separator you want. For example, if you prefer to separate the elements of the array with a dash, you can simply change the separator:
let myArray = ["Apple", "Banana", "Cherry"]
let myString = myArray.joined(separator: "-")
print(myString) // Output: Apple-Banana-Cherry
The possibilities are endless! 😄
The Power of Simplicity 🎈
Now that you know how to convert an array to a string in Swift, you can say goodbye to manual concatenation nightmares and embrace the power of simplicity. Swift's joined(separator:)
method is a clean and efficient way to get the job done.
So go ahead, give it a spin, and let your arrays shine in the form of beautifully formatted strings. Your fellow developers will thank you for it! 💁♂️💁♀️
That's all for now, folks! If you have any other questions or want to share your own insights on Swift, feel free to leave a comment below. Happy coding! 💻✨
🌟💬🌟 Don't forget to share this post with your fellow Swift enthusiasts and spread the joy of easy array-to-string conversions! Together, we can make programming a more pleasant experience for everyone. Cheers! 🥂🎉