How do I convert a date/time string into a different date string?
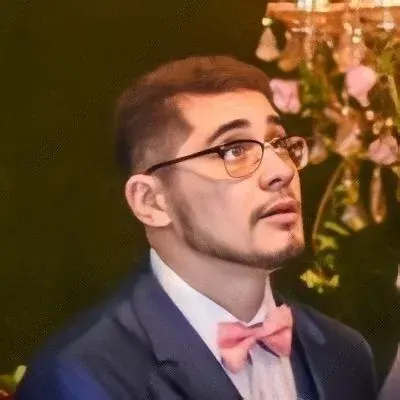
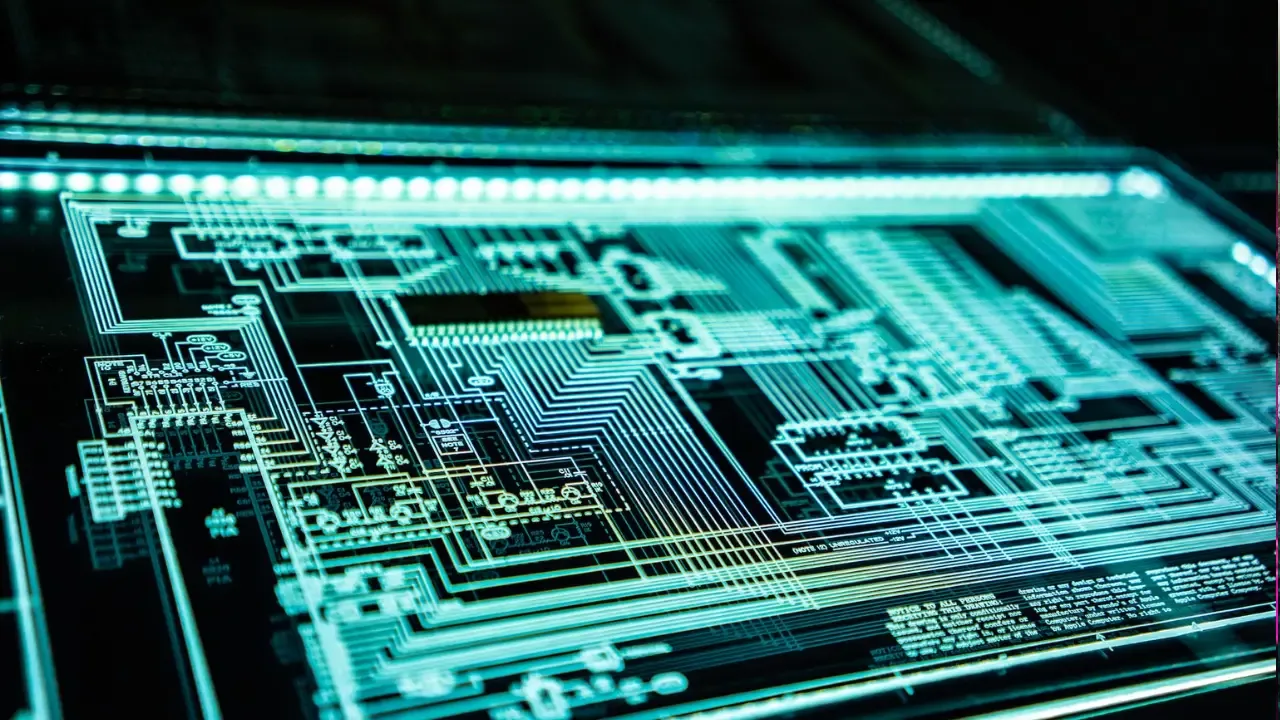
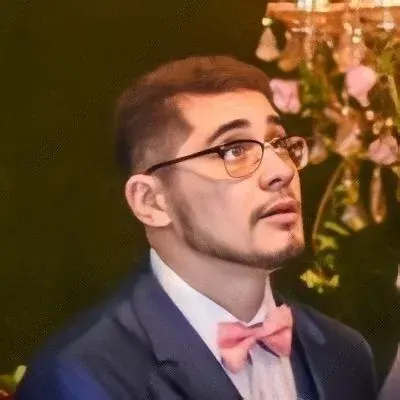
How to Convert a Date/Time String into a Different Date Format
Have you ever found yourself stuck trying to convert a date/time string into a different date format? Don't worry, we've got you covered! In this blog post, we'll address a common issue and provide an easy solution to help you convert that date/time string into the format you desire. 📅💥
The Problem:
Let's consider the following scenario:
From this: 2016-02-29 12:24:26
To this: Feb 29, 2016
You may have tried to use the NSDateFormatter
in your code, just like the example below:
let dateFormatter = NSDateFormatter()
dateFormatter.dateFormat = "MM-dd-yyyy"
dateFormatter.timeZone = NSTimeZone(name: "UTC")
let date: NSDate? = dateFormatter.dateFromString("2016-02-29 12:24:26")
print(date)
However, to your disappointment, the above code snippet might have returned a nil
value. 😕
The Solution:
Fear not, because the issue lies in the mismatched date format in the code. To convert the given date/time string into the desired format, you need to adjust the date format string in the NSDateFormatter
to match the input string format.
In our case, the format of the given date/time string needs to be "yyyy-MM-dd HH:mm:ss". Let's make the necessary adjustments to our code:
let dateFormatter = DateFormatter()
dateFormatter.dateFormat = "yyyy-MM-dd HH:mm:ss"
let date = dateFormatter.date(from: "2016-02-29 12:24:26")
let outputDateFormatter = DateFormatter()
outputDateFormatter.dateFormat = "MMM dd, yyyy"
let outputDateString = outputDateFormatter.string(from: date!)
print(outputDateString)
Using the correct format, you'll now be able to convert the string into the desired format. 🎉
Wrapping Up:
Converting a date/time string into a different format might seem challenging at first, but with the right approach, it becomes a breeze! By following the steps outlined above and using the correct date format, you'll be able to achieve the required result.
So go ahead, give it a try, and embrace the power to convert date/time strings effortlessly! 🕒💪
If you have any further questions or need help with anything else, feel free to leave a comment below. Happy coding! ✨👩💻✨