How do I check if a string contains another string in Objective-C?
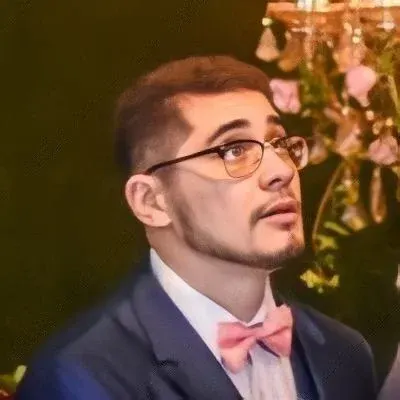
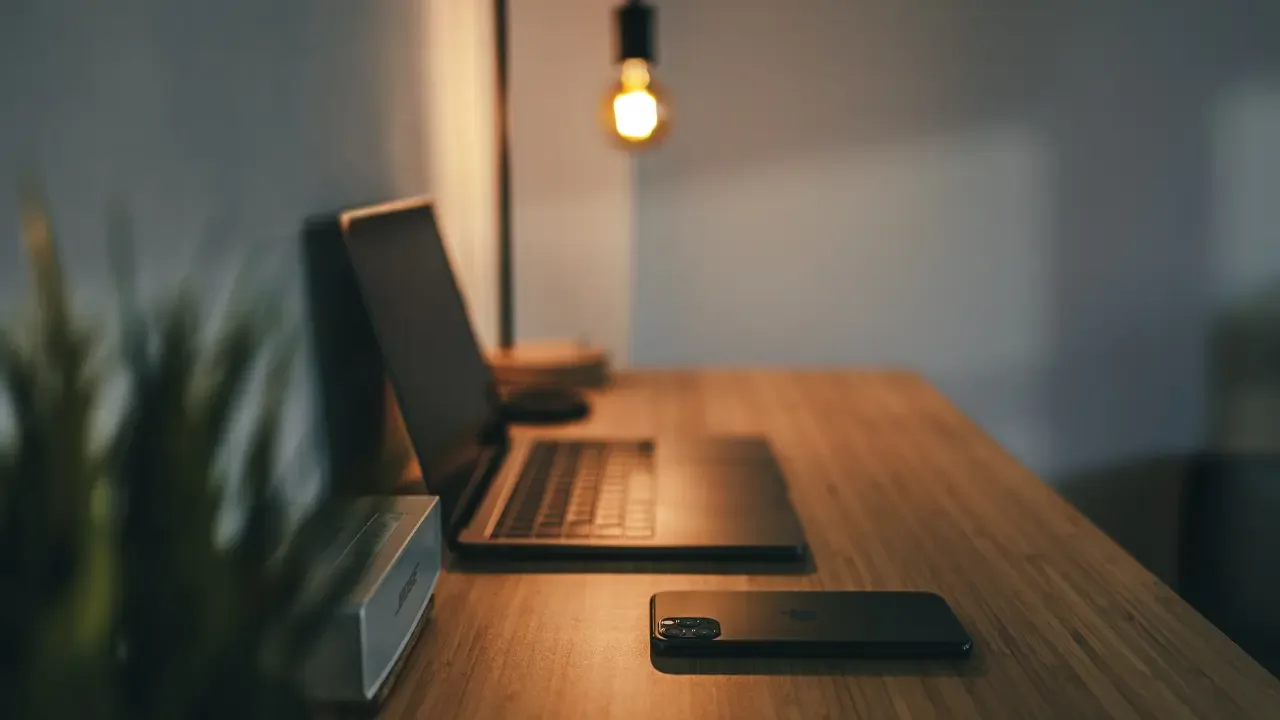
How to Check if a String Contains Another String in Objective-C? 😕💡
Are you looking for an easy way to determine if a string contains another string in Objective-C? You're in luck! In this blog post, I'll show you a simple solution to this common problem. 🙌
The Challenge 💭
Let's begin with the specific problem at hand. You have a string, let's call it string
, and you want to check if it contains another smaller string, let's call it substring
. The ultimate goal is to determine whether substring
exists within string
. 🧐
A Common Approach ⚙️
Based on the context you provided, you discovered the following approach:
if ([string rangeOfString:@"substring"] == 0) {
NSLog(@"Substring doesn't exist");
} else {
NSLog(@"Substring exists");
}
This code snippet utilizes the rangeOfString
method to search for substring
within string
. If the rangeOfString
method returns 0, it means that substring
was found at the beginning of string
. Otherwise, it exists somewhere else within string
. While this approach technically works, we can make it more concise and efficient. 😌
A More Elegant Solution 🌟
To simplify your code and make it more readable, you can use the containsString
method, which was introduced in iOS 8 and works for iOS, macOS, and watchOS. 📱💻⌚️
Here's how you can implement it:
if ([string containsString:@"substring"]) {
NSLog(@"Substring exists");
} else {
NSLog(@"Substring doesn't exist");
}
By using the containsString
method, you can directly check if substring
exists in string
without the need to compare the return value against 0.
Try It Yourself! 💪
To help you grasp the concept, let's apply what we've learned so far using a concrete example. Imagine you have a string that contains the word "Objective-C" and you want to determine if it contains the substring "C". The following code will do the trick:
NSString *string = @"Learning Objective-C is fun!";
NSString *substring = @"C";
if ([string containsString:substring]) {
NSLog(@"The string contains the substring!");
} else {
NSLog(@"The string does not contain the substring!");
}
When you run this code snippet, you should see the following output in your console:
The string contains the substring!
Conclusion and Call-to-Action 👏📢
In this blog post, we explored how to check if a string contains another string in Objective-C. We started by reviewing a common approach and then introduced a more elegant solution using the containsString
method. 🎉
Now it's your turn to try it out! Apply this knowledge to your own projects and make your code cleaner and more efficient. Share your experience and any cool tips you discover in the comments below. Let's level up our Objective-C string manipulation skills together! 💪🚀
Remember to subscribe to our blog for more helpful tips and tricks on Objective-C development. Happy coding! 😄👩💻👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
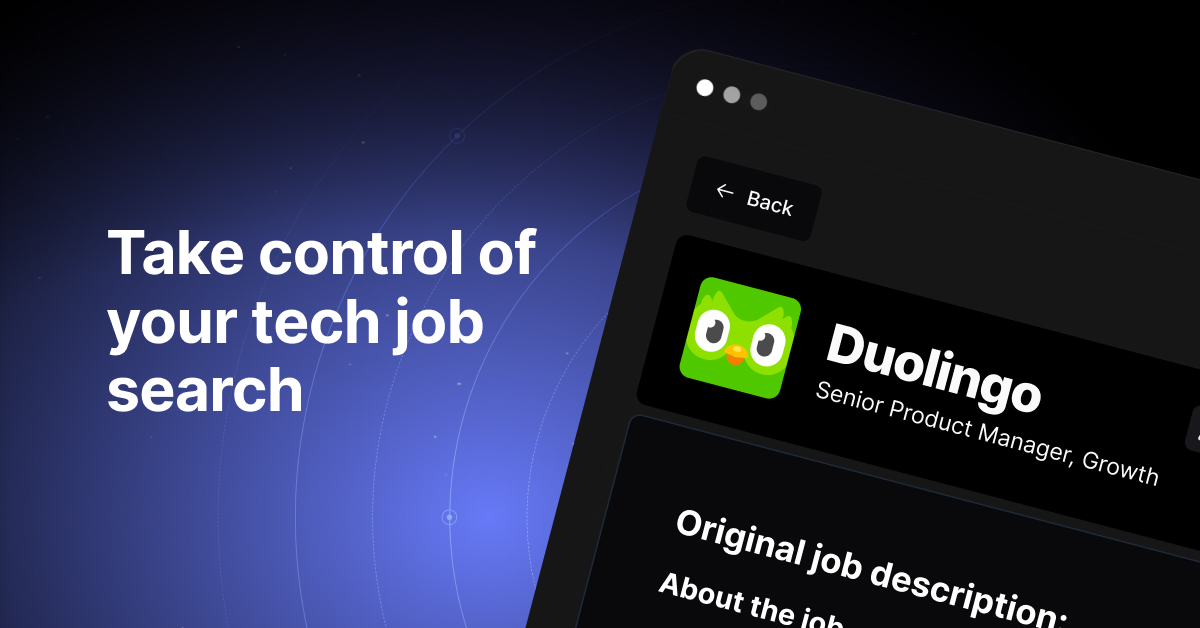