How do I change the font size of a UILabel in Swift?
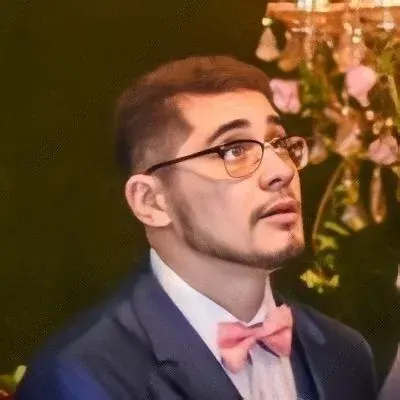
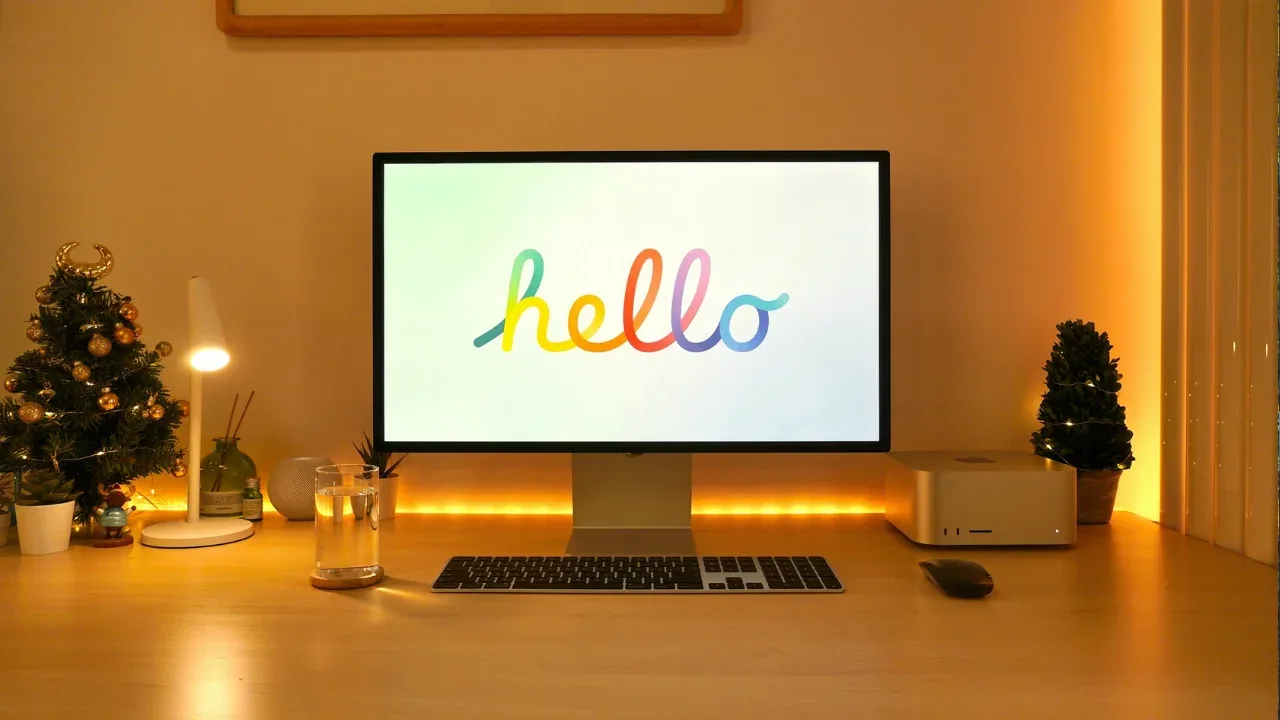
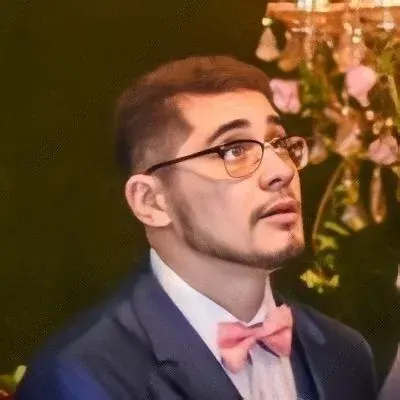
How to Change the Font Size of a UILabel in Swift
Do you want to make your UILabel stand out by adjusting its font size? 🤔 Look no further! In this blog post, we'll explore how you can easily change the font size of a UILabel in Swift. Whether you're a beginner or an experienced Swift developer, we've got you covered. Let's dive in!
The Common Roadblock: Read-Only fontSize Property
You might have noticed that the fontSize
property of a UILabel's font
is read-only, making it a bit tricky to modify. 😬 But don't worry, there's a simple workaround!
Solution: Modifying the Font
To change the font size of a UILabel in Swift, you need to create a new font object based on the existing font. Here's how you can do it:
// Get the current font of the UILabel
let currentFont = label.font
// Create a new font with the desired size
let newFont = UIFont(descriptor: currentFont.fontDescriptor, size: 20)
// Set the new font on the UILabel
label.font = newFont
In this example, we assume that you want to change the font size to 20
. Feel free to adjust it to your desired value. Now let's break down what's happening in the code:
We start by getting the current font of the UILabel using
label.font
.Next, we create a new font object using the
UIFont
initializer. We pass the current font's descriptor and the desired font size as parameters.Finally, we assign the new font to the
font
property of the UILabel usinglabel.font = newFont
.
That's it! You have successfully changed the font size of your UILabel. 🎉
Going the Extra Mile: Dynamic Type Support
If you want to make your app more accessible and user-friendly, consider supporting Dynamic Type. With Dynamic Type, your app's font sizes will automatically adjust according to the user's preferred text size setting.
To enable Dynamic Type support, you can use the UIFontMetrics
class. Here's an example of how you can implement it:
// Create a fontMetrics instance
let fontMetrics = UIFontMetrics(forTextStyle: .body)
// Use the fontMetrics instance to scale your font
let scaledFont = fontMetrics.scaledFont(for: label.font)
// Set the scaled font on the UILabel
label.font = scaledFont
In this example, we create a UIFontMetrics
instance for the .body
text style. Then, we use the scaledFont(for:)
method to scale the label's font based on the user's preferred text size setting. Finally, we assign the scaled font to the font
property of the UILabel.
By implementing Dynamic Type support, you ensure that your app's font sizes adapt to users' preferences, offering a better user experience. 👍
Share Your Success and Get Feedback!
Congratulations on successfully changing the font size of your UILabel in Swift! 🙌 We hope this guide was helpful to you. Feel free to share your success story or ask any questions in the comments below.
If you found this blog post useful, consider sharing it with your fellow developers. Let's spread the knowledge! ✨
Keep coding and stay tuned for more exciting Swift tutorials!
👉 Have you successfully changed the font size of a UILabel in Swift? Share your experience in the comments! 👈