How can I programmatically determine if my app is running in the iphone simulator?
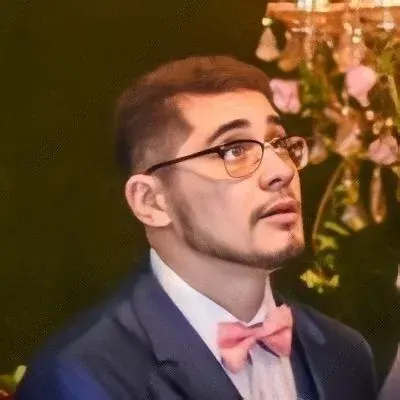
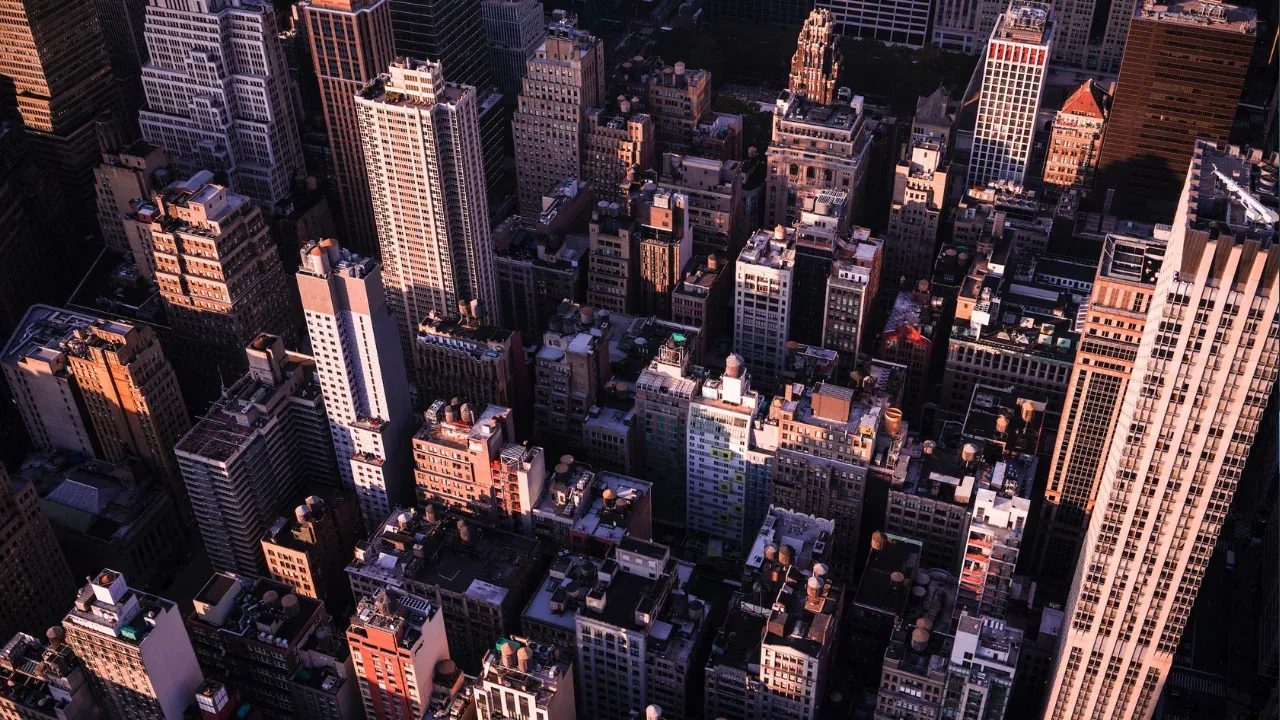
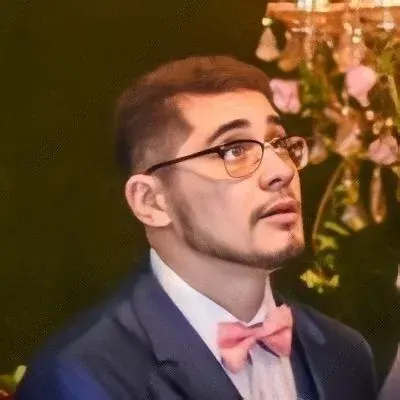
How to Determine if Your App is Running in the iPhone Simulator
Are you a developer looking to determine if your code is running in the iPhone simulator? Do you want to dynamically include or exclude code based on the specific iPhone version or simulator being used? Look no further, as we have got you covered! 📱💻
The Challenge: Determining Simulator vs Actual Device
The first challenge is to determine whether your code is running in the simulator or on an actual iPhone device. This can be crucial for various reasons, such as enabling or disabling specific features or working around simulator-specific limitations.
Solution 1: Detecting the Simulator Environment
One approach is to use the TARGET_OS_SIMULATOR
preprocessor macro to check if your code is running in the simulator. This macro is available in Xcode and is defined when compiling for the simulator. Here's an example of how you can use it:
#if targetEnvironment(simulator)
print("Running in the simulator")
#else
print("Running on a physical device")
#endif
This code snippet checks if the target environment is a simulator and executes the corresponding code block accordingly. Easy, right? 😎
Solution 2: Checking Device Model and Simulator Version
If you want to determine both the simulator and the specific iPhone version, you can use the UIDevice
class from the iOS SDK. Here's an example:
import UIKit
let device = UIDevice.current
if device.model.contains("Simulator") {
print("Running in the simulator")
if let simulatorVersion = device.systemVersion.split(separator: ".").first {
print("Simulator version: \(simulatorVersion)")
}
} else {
print("Running on an actual iPhone device")
print("Device model: \(device.model)")
print("iOS version: \(device.systemVersion)")
}
This code checks if the device model contains the word "Simulator" and prints the corresponding output. It also retrieves the simulator version by splitting the system version string and extracting the first component.
Call-to-Action: Share Your Experience!
We hope these solutions help you determine if your app is running in the iPhone simulator and provide insights into the specific simulator version or device model. Try them out and let us know your experience in the comments below! 👇✍️
Remember, sharing is caring! If you found this article helpful, share it with your fellow developers who might be facing the same challenge. Happy coding! 🚀🔥