Handling applicationDidBecomeActive - "How can a view controller respond to the app becoming Active?"
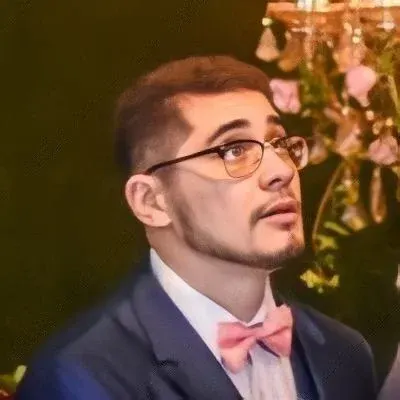

How can a view controller respond to the app becoming Active?
š±š” Handling the applicationDidBecomeActive
method in your AppDelegate can be tricky when you want to call a method within a specific view controller. But worry not! We've got you covered. In this post, we'll address this common issue and provide easy solutions to ensure your view controller responds seamlessly to the app becoming active. So let's dive in and make your app shine! āØ
The Challenge
š§ Here's the challenge: you have the UIApplicationDelegate
protocol in your main AppDelegate.m
class, with the applicationDidBecomeActive
method defined. However, you want to call a method that resides in another view controller. How can you determine which view controller is currently showing in the applicationDidBecomeActive
method and make a call to a method within that controller? Let's break it down step by step!
Step 1: Access the Current View Controller
š In order to determine which view controller is currently active, we need to access the root view controller of the UIWindow
in your AppDelegate. Here's how you can achieve this:
// Swift
func applicationDidBecomeActive(_ application: UIApplication) {
guard let rootViewController = (window?.rootViewController as? UINavigationController)?.visibleViewController else {
return
}
if let viewController = rootViewController as? YourViewController {
// Call your method within the view controller
viewController.yourMethod()
}
}
// Objective-C
- (void)applicationDidBecomeActive:(UIApplication *)application {
UINavigationController *navigationController = (UINavigationController *)self.window.rootViewController;
UIViewController *rootViewController = navigationController.visibleViewController;
if ([rootViewController isKindOfClass:[YourViewController class]]) {
YourViewController *viewController = (YourViewController *)rootViewController;
// Call your method within the view controller
[viewController yourMethod];
}
}
š” In this code snippet, we access the root view controller of the application window, which is assumed to be a UINavigationController
. We then retrieve the currently visible view controller using the visibleViewController
property. Finally, we check if the view controller is of the desired type (YourViewController
) and call the desired method within it.
Step 2: Customize for Your Specific Scenario
š§ The provided code snippet assumes that your root view controller is a UINavigationController
. If that's not the case, you will need to modify the code accordingly. For example, if your root view controller is a UITabBarController
, you can access the current view controller as follows:
// Swift
guard let tabBarController = window?.rootViewController as? UITabBarController,
let selectedViewController = tabBarController.selectedViewController else {
return
}
if let viewController = selectedViewController as? YourViewController {
// Call your method within the view controller
viewController.yourMethod()
}
// Objective-C
UITabBarController *tabBarController = (UITabBarController *)self.window.rootViewController;
UIViewController *selectedViewController = tabBarController.selectedViewController;
if ([selectedViewController isKindOfClass:[YourViewController class]]) {
YourViewController *viewController = (YourViewController *)selectedViewController;
// Call your method within the view controller
[viewController yourMethod];
}
š” Remember, the key is to access the currently active view controller and then cast it to the appropriate type so that you can call the desired method.
Step 3: Engage with Your Users
š¬ Now that you have a clear understanding of how to handle the applicationDidBecomeActive
method and call methods within specific view controllers, you're empowered to create delightful user experiences, tailored to your app's needs. So, go ahead and implement these solutions in your app, and let us know how it goes in the comments section below! We'd love to hear your success stories and even help with any challenges you face along the way.
š Don't forget to share this blog post with your fellow developers who might be struggling with similar issues. Sharing is caring! š
That's a wrap, folks! š Now you're armed with the knowledge to handle the applicationDidBecomeActive
method like a pro. Happy coding! š»āØ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
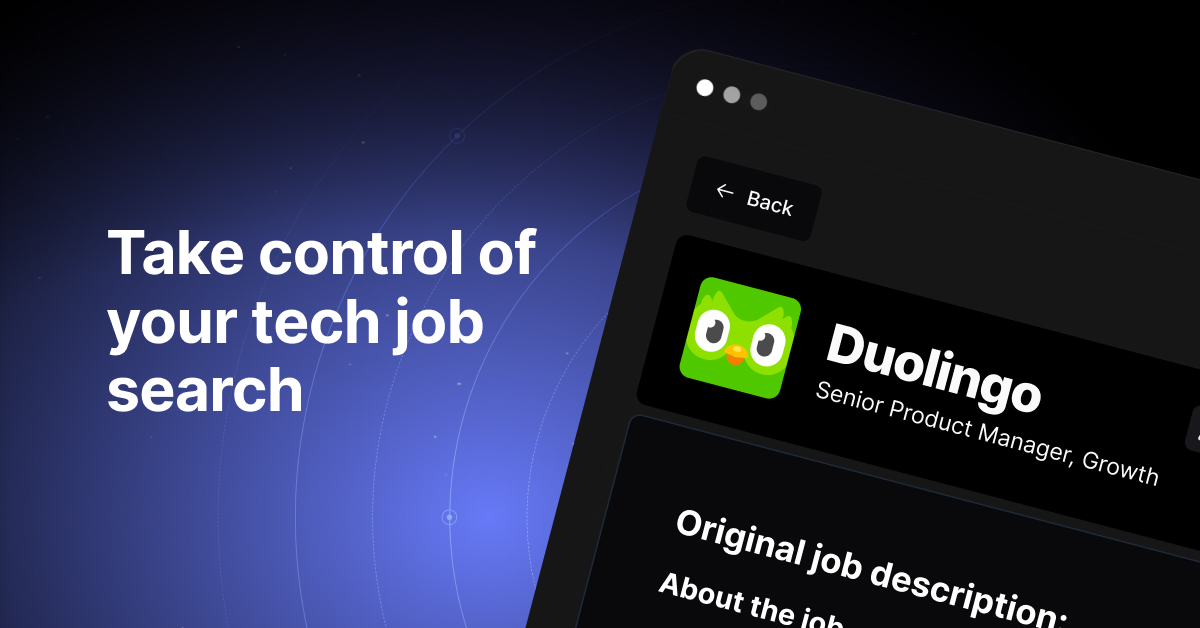