Generate JSON string from NSDictionary in iOS
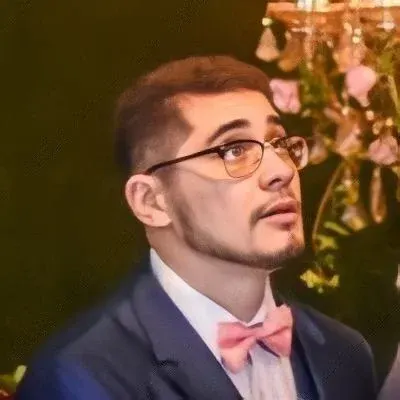
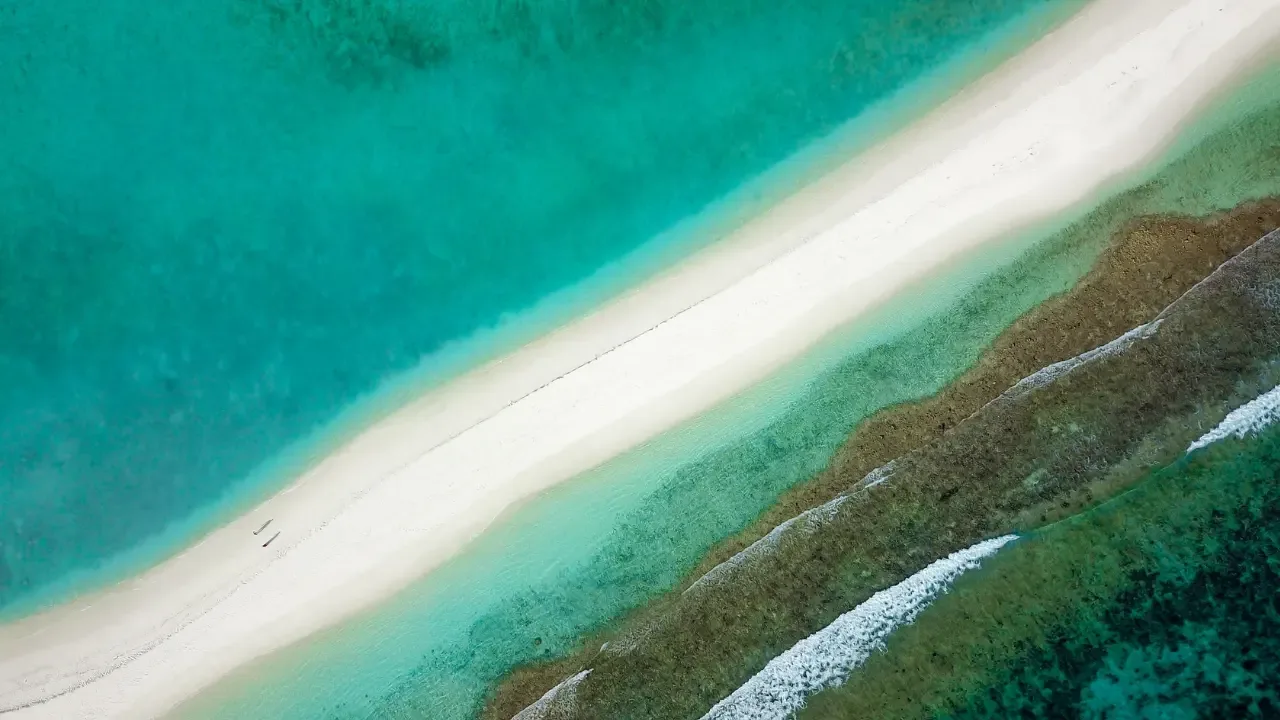
📝 How to Generate a JSON String from NSDictionary in iOS 📱
Hey there, tech enthusiasts! 😎 Have you ever found yourself in a predicament where you need to generate a JSON string from an NSDictionary in iOS? 🤔 Fret not! We've got you covered! In this post, we'll walk you through the process step-by-step, addressing common issues and providing easy solutions. So, let's dive right in! 💪
⚠️ The Challenge: Converting an NSDictionary to a JSON String
The initial question we received was from a user who had a dictionary and needed to convert it into a JSON string. They were seeking assistance on whether this conversion was possible and, of course, our tech-savvy community is always ready to lend a helping hand! 🤝
💡 Easy Solution: Utilizing NSJSONSerialization
So, how can we achieve this conversion in iOS? The answer lies within the NSJSONSerialization class, which provides a straightforward way to convert NSDictionary objects to JSON strings. Here's a handy code snippet to get you started:
let dictionary: NSDictionary = ["key1": "value1", "key2": "value2"] // Your NSDictionary
let jsonData: Data? = try? JSONSerialization.data(withJSONObject: dictionary, options: .prettyPrinted)
if let jsonData = jsonData {
let jsonString = String(data: jsonData, encoding: .utf8)
// Now you have your JSON string
}
📍 Explanation: Breaking Down the Code
1️⃣ First, you'll create an NSDictionary object containing your key-value pairs. Feel free to customize it to suit your needs.
2️⃣ Next, you'll use the JSONSerialization.data(withJSONObject:options:) method to convert the NSDictionary into JSON data. The options parameter allows customization of the JSON output format. We're using the .prettyPrinted option here to add indentation and line breaks for readability.
3️⃣ Provided that there are no errors during the conversion, you'll then convert the JSON data into a string representation using the String(data:encoding:) initializer. We're using the .utf8 encoding to ensure compatibility.
4️⃣ Finally, you'll have your JSON string ready to be used further in your iOS app. 🎉
🔧 Common Issues and Troubleshooting
No Pretty Print Option - If you don't require pretty-printed JSON and prefer a more compact format, simply remove the option .prettyPrinted while calling the JSONSerialization.data(withJSONObject:options:) method.
Invalid JSON - Keep in mind that the dictionary's keys and values must be types supported by JSON (e.g., NSString, NSNumber, NSArray, or NSDictionary). Failure to adhere to this while building your dictionary might result in an invalid JSON format.
Error Handling - In the code snippet, we utilized optionals to handle potential errors during the data creation and string conversion. Do make sure to incorporate proper error handling in your production code.
📢 Your Turn: Engage with the Community!
Voila! You now have the power to generate a JSON string from an NSDictionary in iOS! 💪 We hope this guide was helpful and shed light on any confusion you may have had. If you have any suggestions, tips, or unique use cases, please share them in the comments below! Let's keep the discussions flowing and help each other grow as tech wizards! ✨
✨ Remember, sharing is caring! If you found this guide useful, don't forget to hit the share button and spread the knowledge among your friends and colleagues! 📲
Happy coding! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
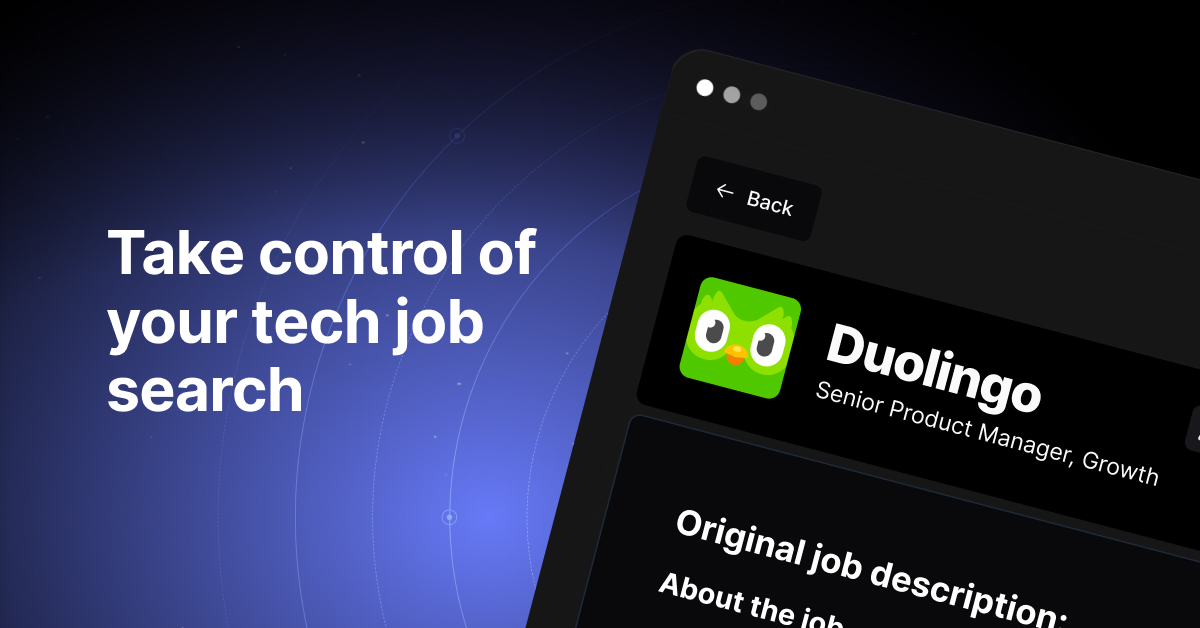