Generate a UUID on iOS from Swift
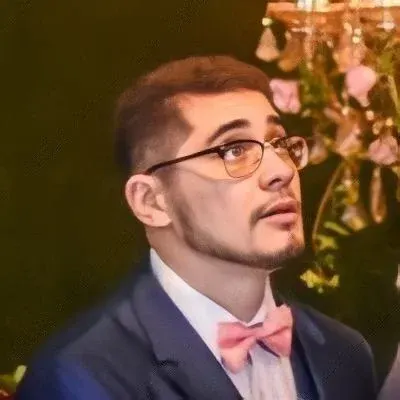
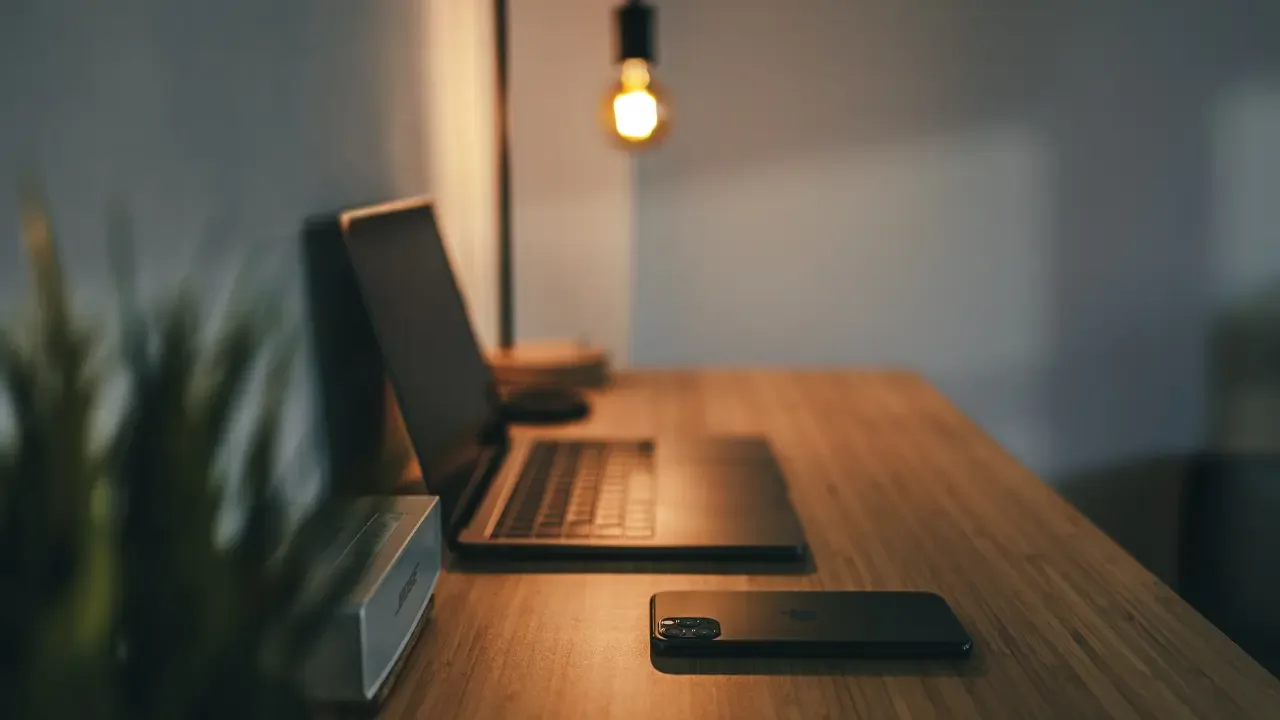
πTitle: Generating a Unique Identifier (UUID) in iOS with Swift: A Safer and More Efficient Approach! ππ±
Introduction: Is your iOS Swift app in need of generating random UUID (GUID) strings for various purposes, such as table keys? In this blog post, we will explore a common code snippet to generate UUID and evaluate its safety. Furthermore, we will present you with a better, recommended approach to accomplish this task. Let's dive in and discover the best practices! πͺ
The Existing Approach:
The code snippet you shared, which generates a UUID using the CFUUIDCreateString
function, seems to work perfectly on the surface:
let uuid = CFUUIDCreateString(nil, CFUUIDCreate(nil))
Safety Concerns: While the above code snippet might work, it's important to question its safety and consider potential issues that may arise. π€ Here are a few concerns:
1οΈβ£ Deprecated APIs: The CFUUIDCreate
method, used in the code snippet, has been deprecated since iOS 9. It is no longer recommended for new projects and may eventually be removed from future iOS versions.
2οΈβ£ Thread Safety: The code snippet does not provide thread safety guarantees. In a multi-threaded environment, there is a risk of generating non-unique UUIDs due to race conditions.
3οΈβ£ Performance Overhead: The CFUUIDCreate
function consumes additional system resources by creating a UUID object that is not necessary to generate the UUID string alone. This can impact the app's performance, especially when generating UUIDs in bulk.
The Recommended Approach:
To address the concerns mentioned above and ensure a safer and more efficient approach, we recommend leveraging Swift's UUID
struct, introduced in iOS 8 and later. Here's the updated code:
let uuid = UUID().uuidString
Advantages of the Recommended Approach:
β
Safety: The UUID
struct is designed to generate unique identifiers safely, eliminating the race conditions and deprecated API concerns.
β
Simplicity: Using the UUID
struct provides clearer and more readable code, making it easier for developers to understand and maintain.
β Performance: The new approach generates the UUID string directly without unnecessarily creating a UUID object, improving performance.
Conclusion:
Generating a unique identifier (UUID) in iOS Swift is an essential task for many applications. While the initial code snippet using CFUUIDCreateString
might appear to work, it poses safety concerns and introduces unnecessary performance overhead. By adopting the recommended approach utilizing Swift's UUID
struct, you ensure thread safety, compatibility with newer iOS versions, and an optimized performance. Upgrade your codebase and embrace the power of Swift's built-in capabilities!
π£ Call-to-Action: Have you encountered any issues with generating UUIDs in your iOS app? Share your experience or any alternative approaches you've used in the comments below! Let's generate some engagement and knowledge-sharing! ππ¬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
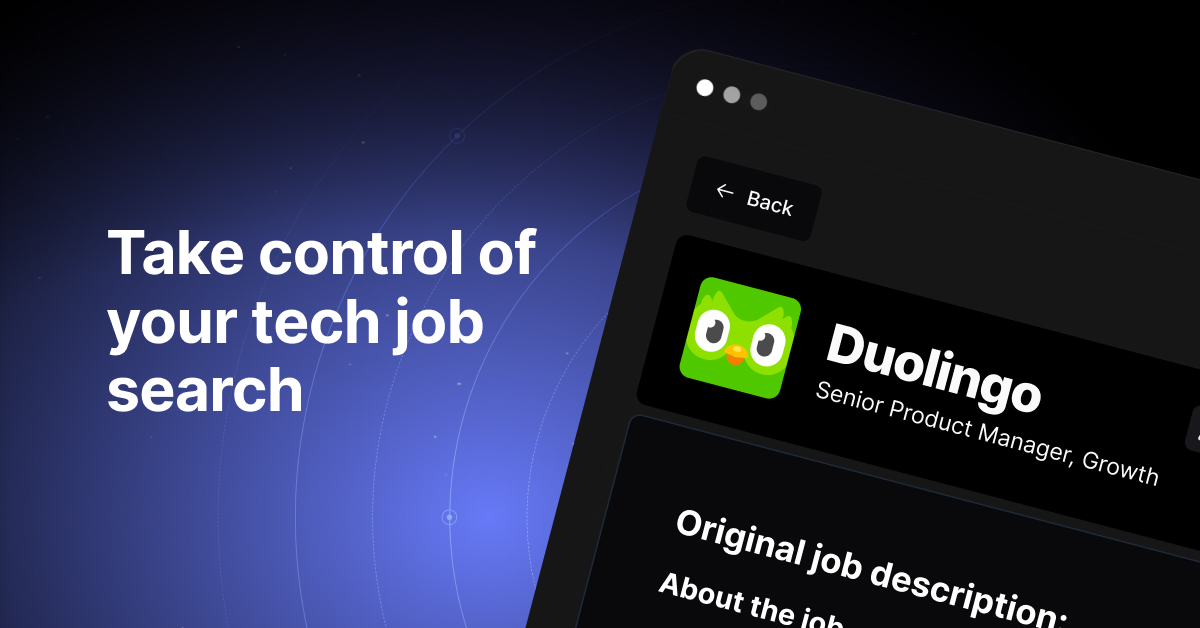