Figure out size of UILabel based on String in Swift
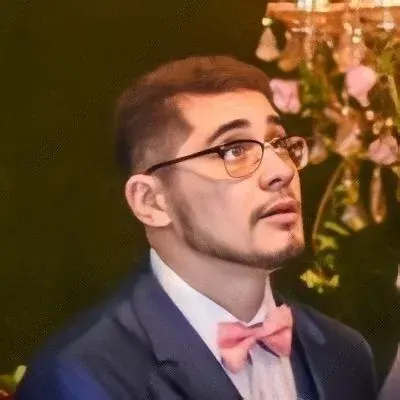
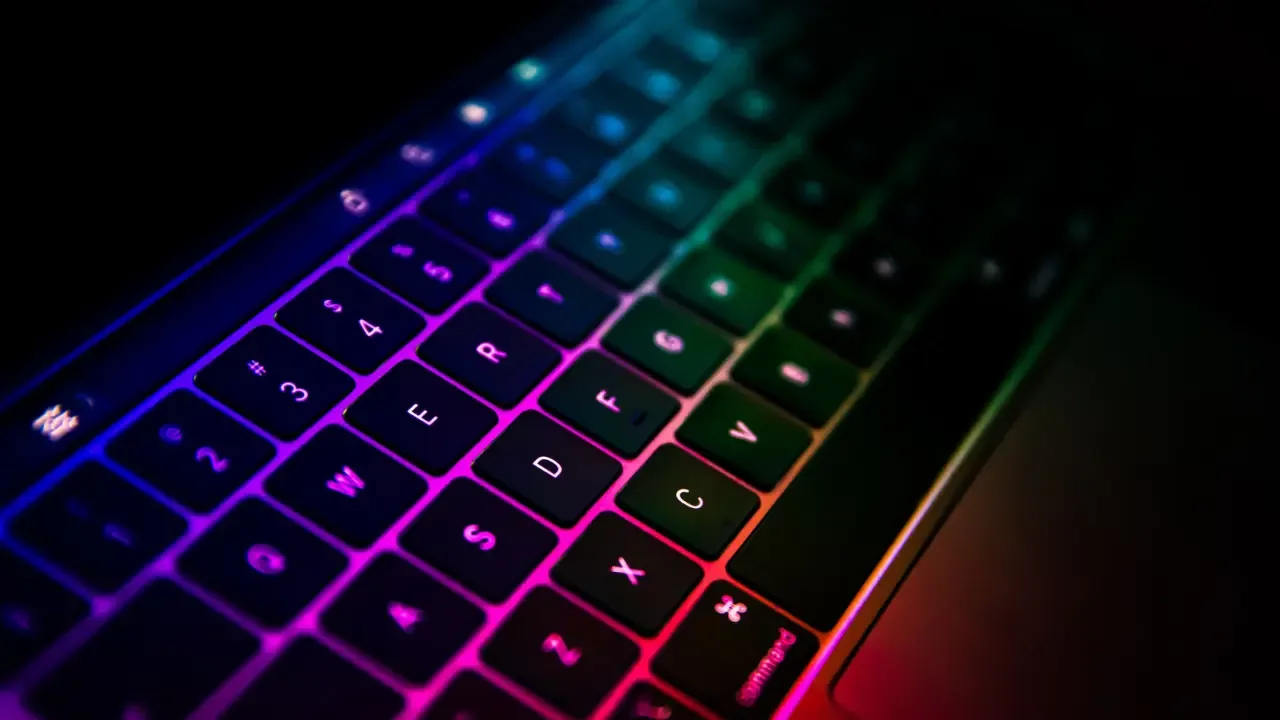
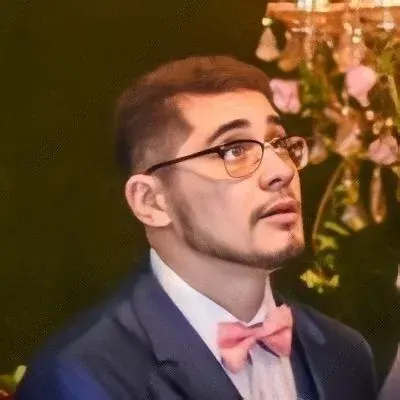
📝 Easy Guide to Calculate UILabel Size in Swift
Are you struggling to figure out the size of a UILabel based on different string lengths in your Swift project? Don't worry, we've got you covered! In this blog post, we will address this common issue and provide you with easy solutions to accurately calculate the height of your UILabel.
🤔 The Problem:
The code snippet provided seems to be facing some difficulties in calculating the expected label size accurately. The existing function, calculateContentHeight()
, is not giving the desired results. But fret not, we'll dive into the code and help you understand the problem!
✨ Solution Approach:
To resolve this issue, we'll need to make a few modifications to the code. Let's break it down step-by-step:
Define the maximum label size: We'll start by defining the maximum size of the UILabel using the
CGSize
struct. In this example, it's set toframe.size.width - 48
for width and9999
for height. Adjust these values according to your requirements.Convert content to NSString: Convert the contentText to an NSString using the
as NSString
conversion.Calculate the expected label size: Use the
boundingRect(with:options:attributes:context:)
method of NSString to calculate the expected size. Pass in the maximum label size, options for line fragment origin, and the desired font attributes. In the provided example, we use[NSFontAttributeName: UIFont.systemFontOfSize(16.0)]
as the font.Print and return the height: Print the expected label size to observe the results. Return only the height from the
expectedLabelSize
as the calculated content height.
Here's an updated version of the code:
func calculateContentHeight() -> CGFloat {
let maxLabelSize = CGSize(width: frame.size.width - 48, height: CGFloat(9999))
let contentNSString = contentText as NSString
let expectedLabelSize = contentNSString.boundingRect(
with: maxLabelSize,
options: .usesLineFragmentOrigin,
attributes: [NSAttributedString.Key.font: UIFont.systemFont(ofSize: 16.0)],
context: nil
)
print("\(expectedLabelSize)")
return expectedLabelSize.size.height
}
🚀 Call-to-Action:
Now that you have a better understanding of how to calculate the size of a UILabel based on different string lengths in Swift, try implementing this solution in your own project. If you encounter any issues or have further questions, feel free to leave a comment below. We're here to help!
If you found this guide helpful, please share it with your fellow developers to save them from the UILabel size calculation struggle! 🙌
Keep coding and stay tuned for future insightful articles! Happy coding! 😄👩💻👨💻