Execute action when back bar button of UINavigationController is pressed
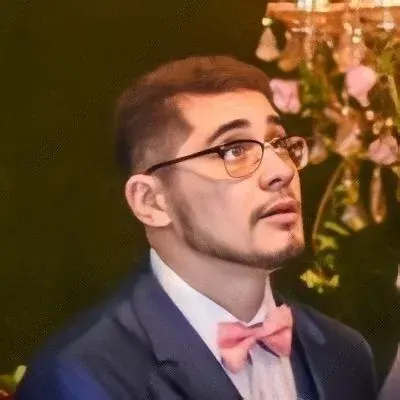
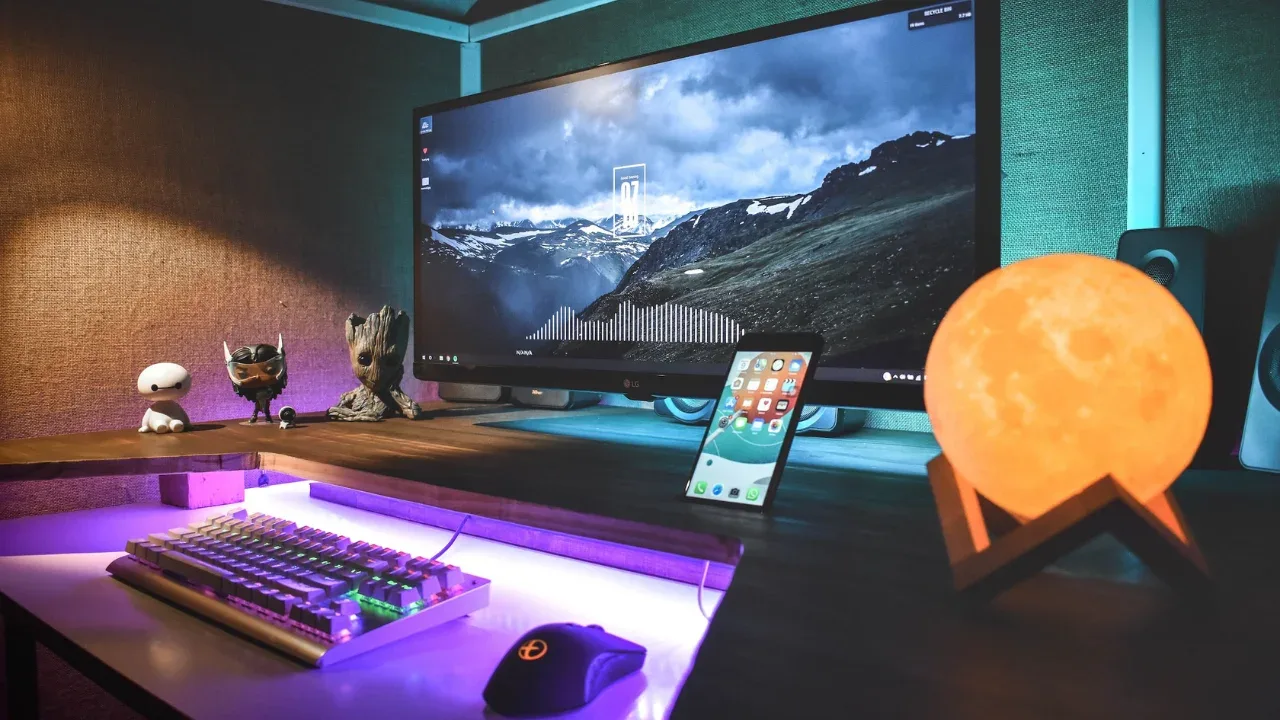
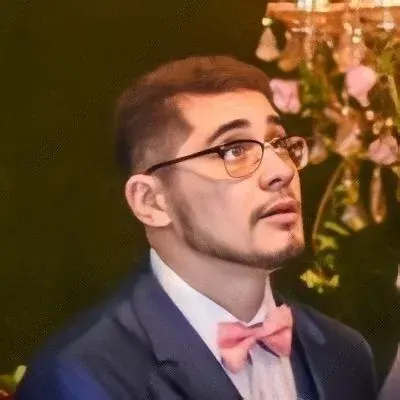
How to Execute an Action When the Back Bar Button of a UINavigationController is Pressed in Swift π
Have you ever needed to perform a specific action when the back button of a UINavigationController is pressed in your iOS app? Maybe you want to clear some data or update a view before navigating back to the previous screen. In this blog post, we'll explore a common issue developers face and provide easy solutions to execute an action when the back button is pressed. Let's dive in! πͺ
The Challenge: Executing an Action While Navigating Back π€
The UINavigationController in iOS provides a built-in back button that pops the top view controller from the navigation stack and returns to the previous screen. However, by default, there is no straightforward way to execute custom code when the back button is pressed.
Here's an example scenario: let's say you have a UINavigationController with multiple view controllers stacked on top of each other. When the user taps the back button, you want to empty an array used by the current view controller.
Solution: Method Swizzling to the Rescue! π¦ΈββοΈ
One way to tackle this issue is by utilizing method swizzling, a technique in which you exchange the implementation of a method with a new one at runtime. By doing so, we can intercept the back button press event and execute our custom code before the default navigation behavior takes place.
Here's how you can implement this approach in Swift:
extension UINavigationController {
private static let swizzleMethod: Void = {
let originalSelector = #selector(UINavigationController.viewWillDisappear(_:))
let swizzledSelector = #selector(UINavigationController.customViewWillDisappear(_:))
let originalMethod = class_getInstanceMethod(UINavigationController.self, originalSelector)
let swizzledMethod = class_getInstanceMethod(UINavigationController.self, swizzledSelector)
method_exchangeImplementations(originalMethod!, swizzledMethod!)
}()
@objc private func customViewWillDisappear(_ animated: Bool) {
// Add your custom code here
// Perform the action you want before navigating back
// Call the original implementation
customViewWillDisappear(animated)
}
open override func viewDidLoad() {
super.viewDidLoad()
UIViewController.initializeSwizzleMethod
}
}
With this extension added to your project, any UINavigationController in your app will automatically swizzle the viewWillDisappear
method to our custom implementation, allowing us to execute additional code upon the back button press.
Example Usage: Emptying an Array When Navigating Back ποΈ
To make things more tangible, let's see an example of how you can use the solution described above to empty an array when navigating back.
Assuming you have a view controller class called MyViewController
that manages the array you want to empty, you can override the viewWillDisappear
method in MyViewController
like so:
class MyViewController: UIViewController {
var myArray: [Any] = []
override func viewDidLoad() {
super.viewDidLoad()
// Configure your view controller as needed
}
override func viewWillDisappear(_ animated: Bool) {
super.viewWillDisappear(animated)
// Perform your custom action here
myArray.removeAll()
}
}
By overriding viewWillDisappear
in MyViewController
, you can safely perform any necessary clean-up operations, such as emptying the array, before the view controller is popped from the navigation stack.
Go Ahead and Customize Your UINavigationController! π¨
Now that you have a clear solution to executing actions when the back button of a UINavigationController is pressed, feel free to customize your navigation flow to fit your app's needs. Whether you need to update views, clear data, or trigger specific actions, you now have the power to do so!
Share your experience with us! Have you faced similar challenges when working with UINavigationController, or do you have other tips to share? Let us know in the comments below. Happy coding! π