Detect current device with UI_USER_INTERFACE_IDIOM() in Swift
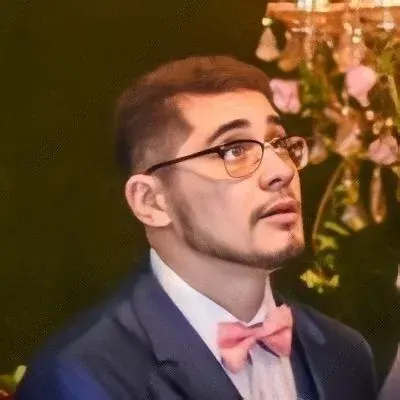
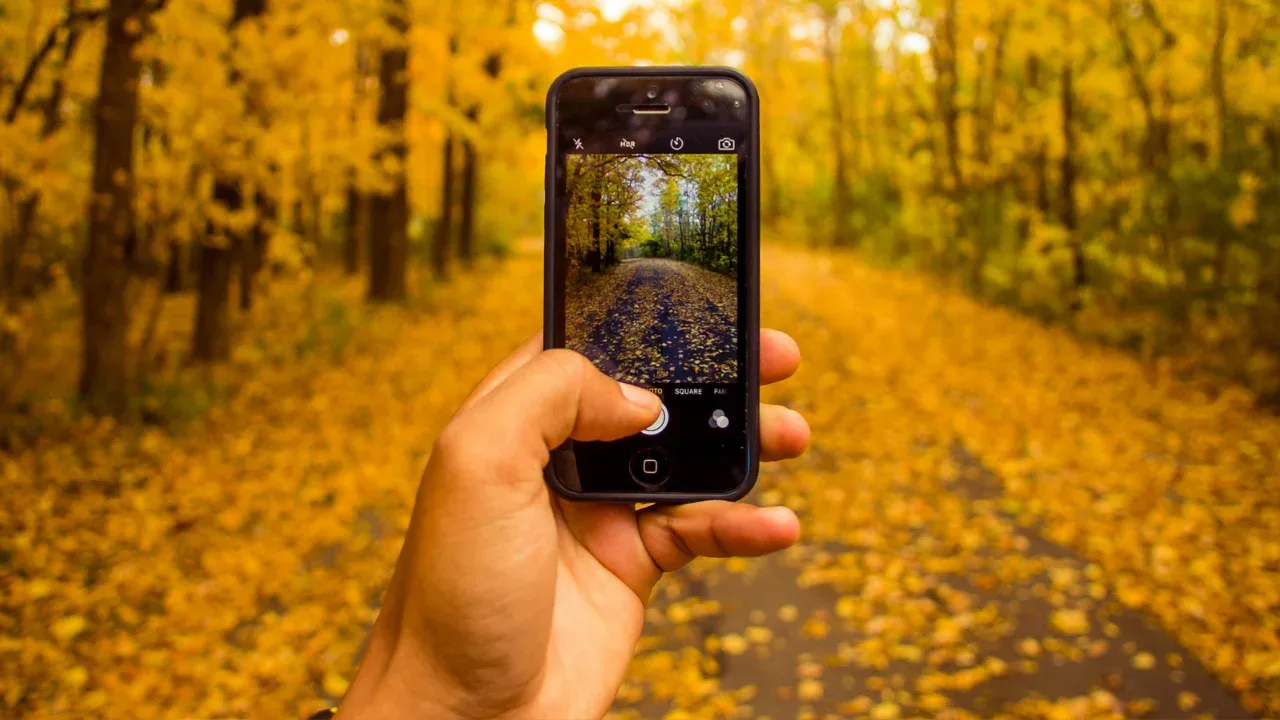
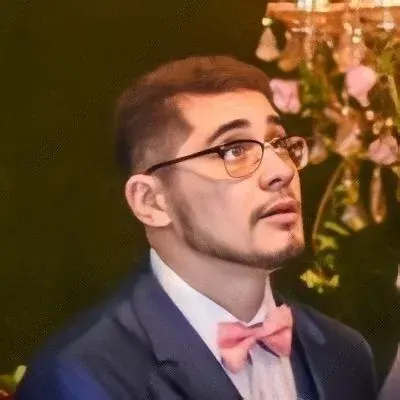
📱🖥️ How to Detect the Current Device in Swift
Are you developing an iOS app and need to detect whether it's running on an iPhone or an iPad? 🤔 Look no further! In this blog post, we'll explore the equivalent of UI_USER_INTERFACE_IDIOM()
in Swift and how to use it to identify the current device.
The Problem: "Use of unresolved identifier" error
The first hurdle you might encounter when trying to use UI_USER_INTERFACE_IDIOM()
in Swift is the dreaded "Use of unresolved identifier" error. 😱 This error typically occurs when you're trying to use an undefined variable or function.
The Solution: Use UIDevice.current.userInterfaceIdiom
In Swift, the equivalent of UI_USER_INTERFACE_IDIOM()
is UIDevice.current.userInterfaceIdiom
. 🎉 This property returns an enumeration value representing the type of device the app is currently running on.
Here's an example of how you can use it to detect the current device:
import UIKit
func getCurrentDevice() -> String {
switch UIDevice.current.userInterfaceIdiom {
case .phone:
return "iPhone"
case .pad:
return "iPad"
case .tv:
return "Apple TV"
case .carPlay:
return "CarPlay"
case .mac:
return "Mac"
@unknown default:
return "Unknown"
}
}
let currentDevice = getCurrentDevice()
print("The app is running on: \(currentDevice)")
In this example, we define a function getCurrentDevice()
that uses a switch
statement to check the value of UIDevice.current.userInterfaceIdiom
. Depending on the device type, it returns a string representing the device.
💡 Pro Tip: Using the iPad-specific features
Detecting the current device can be useful when you want to provide a different user interface or functionality for iPad-specific features. For example, you might want to display a split view controller only on iPads.
To target only iPads, you can modify the getCurrentDevice()
function like this:
func isiPad() -> Bool {
UIDevice.current.userInterfaceIdiom == .pad
}
if isiPad() {
// Custom logic for iPad
} else {
// Logic for other devices, like iPhones
}
What about SwiftUI?
If you're using SwiftUI, you can use the @Environment
property wrapper to access the UIDevice
instance and its userInterfaceIdiom
property.
Here's an example of how you can use it in SwiftUI:
import SwiftUI
struct ContentView: View {
@Environment(\.horizontalSizeClass) var horizontalSizeClass
var body: some View {
if horizontalSizeClass == .compact {
Text("Running on an iPhone")
} else {
Text("Running on an iPad")
}
}
}
In this example, we use the @Environment(\.horizontalSizeClass)
property wrapper to access the current horizontalSizeClass
value, which indicates whether the device is an iPhone or an iPad.
Conclusion
Detecting the current device in Swift is essential for providing a tailored user experience on different iOS devices. By using UIDevice.current.userInterfaceIdiom
, you can easily identify whether the app is running on an iPhone, iPad, or other devices.
Now that you know how to detect the current device, go ahead and make your iOS app shine on every screen size! ✨
If you found this blog post helpful, don't forget to share it with your fellow developers. And if you have any questions or suggestions for future topics, leave a comment below! Let's keep the conversation going! 👇