Create singleton using GCD"s dispatch_once in Objective-C
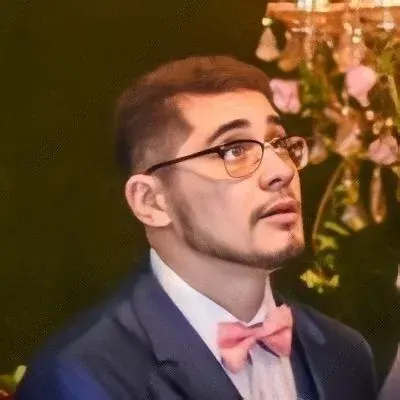
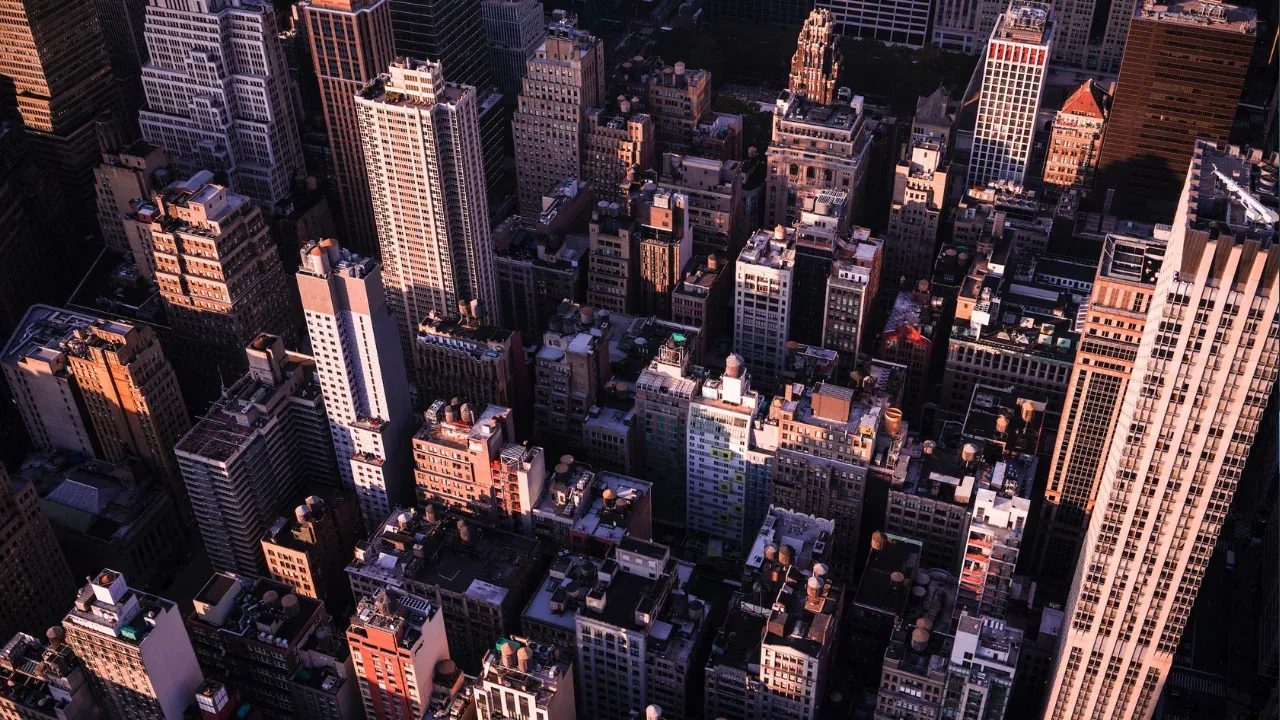
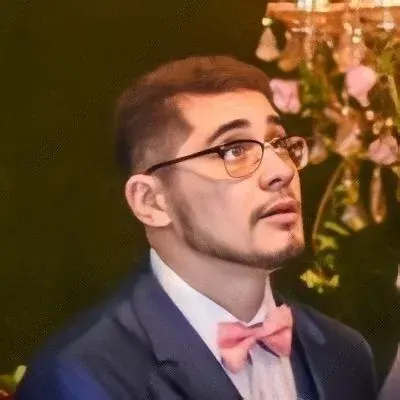
Creating a Singleton Using GCD's dispatch_once in Objective-C ๐
So, you want to create a singleton in Objective-C that is thread safe? ๐งต
Look no further! In this blog post, we'll explore how you can achieve this using GCD's dispatch_once
function. Let's dive right in!
The Problem ๐ค
When creating a singleton, we want to ensure that only one instance of the class is ever created, regardless of the number of threads accessing it. This guarantees consistency and prevents race conditions.
Traditionally, developers have used synchronization techniques like @synchronized
or pthread_mutex
to achieve thread safety. However, with the introduction of Grand Central Dispatch (GCD), we can leverage its powerful dispatch_once
function to simplify our code and ensure thread safety.
The Solution ๐ก
To create a singleton using dispatch_once
, follow these simple steps:
+ (instancetype)sharedInstance {
static dispatch_once_t once;
static id sharedInstance;
dispatch_once(&once, ^{
sharedInstance = [[self alloc] init];
});
return sharedInstance;
}
Let's break down what's happening here:
We declare a
dispatch_once_t
variable namedonce
. This acts as a token to ensure the block of code withindispatch_once
is executed only once.We also define a
static
variablesharedInstance
of typeid
. This will hold the singleton instance.Inside the
dispatch_once
block, we initialize thesharedInstance
variable by allocating and initializing a new instance of the class. This block of code is only executed once, regardless of how many threads call thesharedInstance
method.Finally, we return the
sharedInstance
.
How It Works ๐ ๏ธ
The magic here lies in the dispatch_once
function. It guarantees that the provided block of code is executed in a thread-safe manner, preventing multiple threads from simultaneously creating multiple instances of the singleton.
The block is executed atomically, meaning that once a thread enters the dispatch_once
block, any other waiting threads are automatically blocked until the block completes execution.
Conclusion ๐
And there you have it! By utilizing GCD's dispatch_once
function, we've created a thread-safe singleton in Objective-C.
Not only does this approach simplify our code by eliminating the need for manual synchronization techniques, but it also ensures that we have a single instance that can be accessed across multiple threads without any worries.
So go ahead, give it a try! And if you have any questions or suggestions, feel free to leave a comment below. โ๏ธ
Happy coding! ๐ป๐