Converting String to Int with Swift
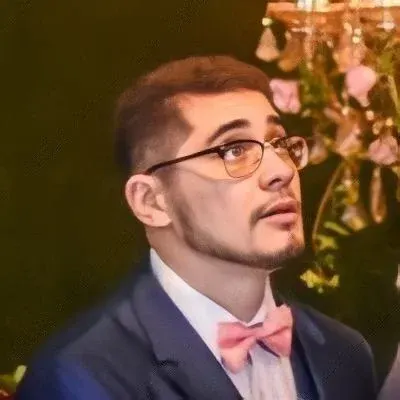
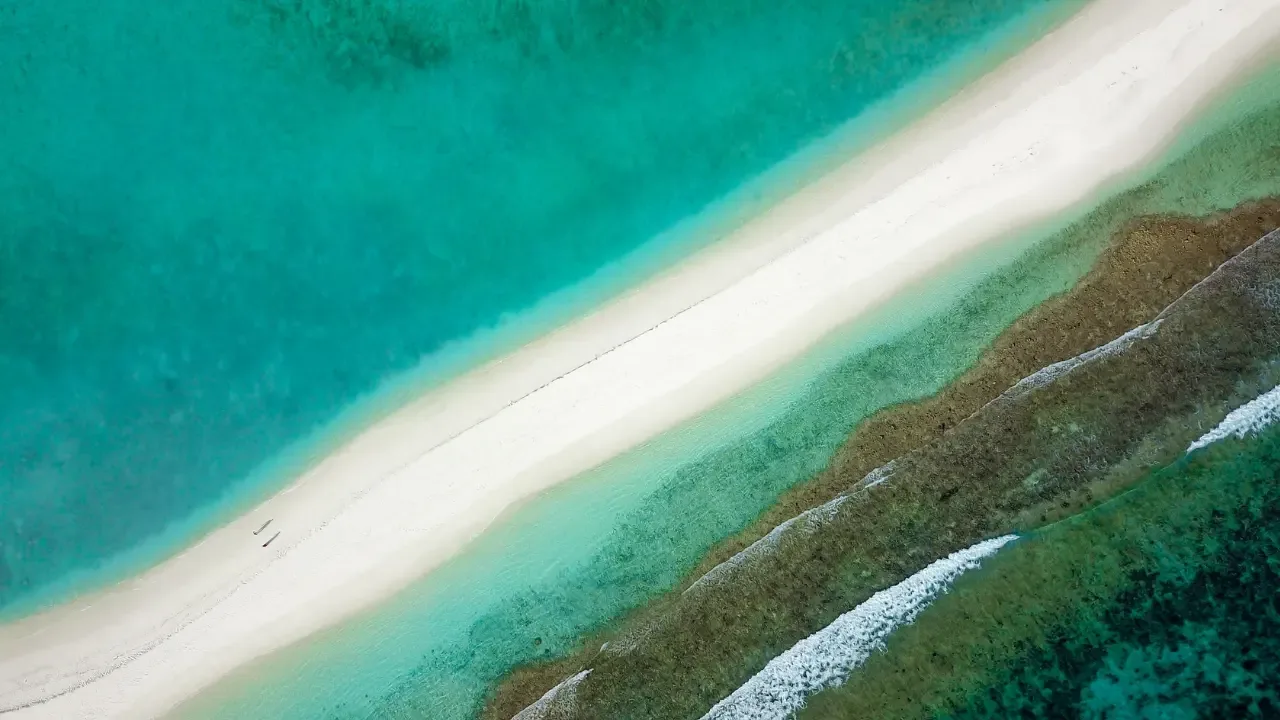
Converting String to Int with Swift: A Guide for iOS Developers
So, you're creating an app that calculates acceleration, but you're facing a common issue - converting string values to integers. Don't worry, we've got you covered! In this guide, we'll walk you through the process of converting strings to integers in Swift, so you can get your app up and running smoothly. Let's dive in! 💻🚀
Understanding the Issue
In the given code snippet, the values in the text boxes are stored as strings (txtBox1, txtBox2, and txtBox3). However, to perform calculations, you need to convert these values into integers. This is where you're facing a challenge.
Solution 1: Using the Int initializer
One way to convert a string to an integer in Swift is by using the Int
initializer. It attempts to convert the string into an integer and returns an optional value. If the conversion is successful, the optional will contain the integer value, otherwise it will be nil
.
Here's how you can use this approach in your code:
@IBAction func btn1(sender : AnyObject) {
let answer1 = "The acceleration is"
guard let stringValue1 = txtBox1.text,
let intValue1 = Int(stringValue1),
let stringValue2 = txtBox2.text,
let intValue2 = Int(stringValue2),
let stringValue3 = txtBox3.text,
let intValue3 = Int(stringValue3) else {
// Handle invalid input here
return
}
// Use the converted integer values
let acceleration = intValue2 - intValue1 / intValue3
lblAnswer.text = "\(answer1) \(acceleration)"
}
In the above code, we use optional binding (guard let
) to safely unwrap the optional string values and attempt to convert them into integers using the Int
initializer. If any of the conversions fail, we handle the invalid input scenario gracefully.
Solution 2: Using Optional Chaining
Another approach to convert a string to an integer is by using optional chaining. This allows you to chain multiple operations together and gracefully handle any potential failures.
@IBAction func btn1(sender : AnyObject) {
let answer1 = "The acceleration is"
if let stringValue1 = txtBox1.text,
let intValue1 = Int(stringValue1),
let stringValue2 = txtBox2.text,
let intValue2 = Int(stringValue2),
let stringValue3 = txtBox3.text,
let intValue3 = Int(stringValue3) {
// Use the converted integer values
let acceleration = intValue2 - intValue1 / intValue3
lblAnswer.text = "\(answer1) \(acceleration)"
} else {
// Handle invalid input here
}
}
In this code snippet, we use a series of if let
statements to check each conversion and only proceed if all of them succeed. Otherwise, we handle the invalid input scenario.
Additional Tips
To ensure a smooth user experience, consider implementing input validation to handle scenarios where the user enters non-numeric values or leaves the text fields empty.
You can also use the
UITextFieldDelegate
methods to dismiss the keyboard after the user finishes entering the values.
Conclusion
Congratulations! You now have two easy-to-implement solutions for converting string values to integers in Swift. By using the Int
initializer or optional chaining, you can seamlessly convert the input from string format to a format that your acceleration calculation algorithm can work with.
Now it's time to implement these solutions in your app and give it a go! If you encounter any further challenges or have any questions, feel free to reach out to the Swift developer community or leave a comment below. Happy coding! 😄👩💻
Have you ever faced challenges while converting strings to integers in Swift? How did you solve it? Share your experiences and solutions in the comments below!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
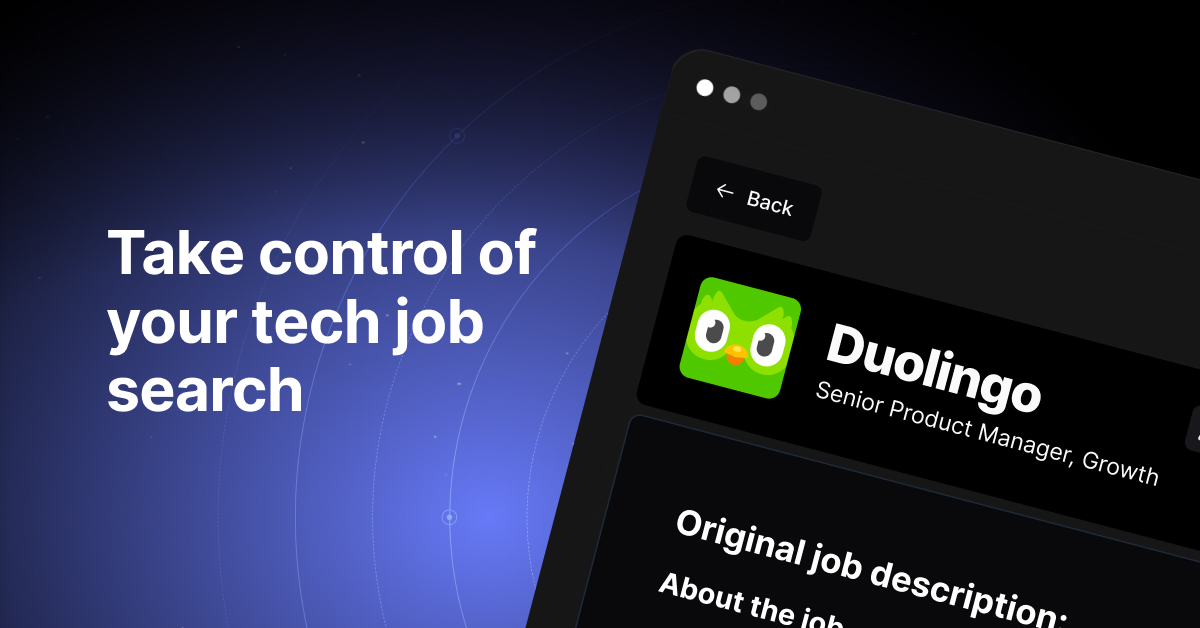