Converting NSString to NSDate (and back again)
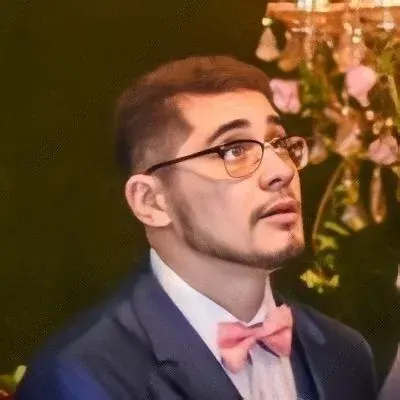
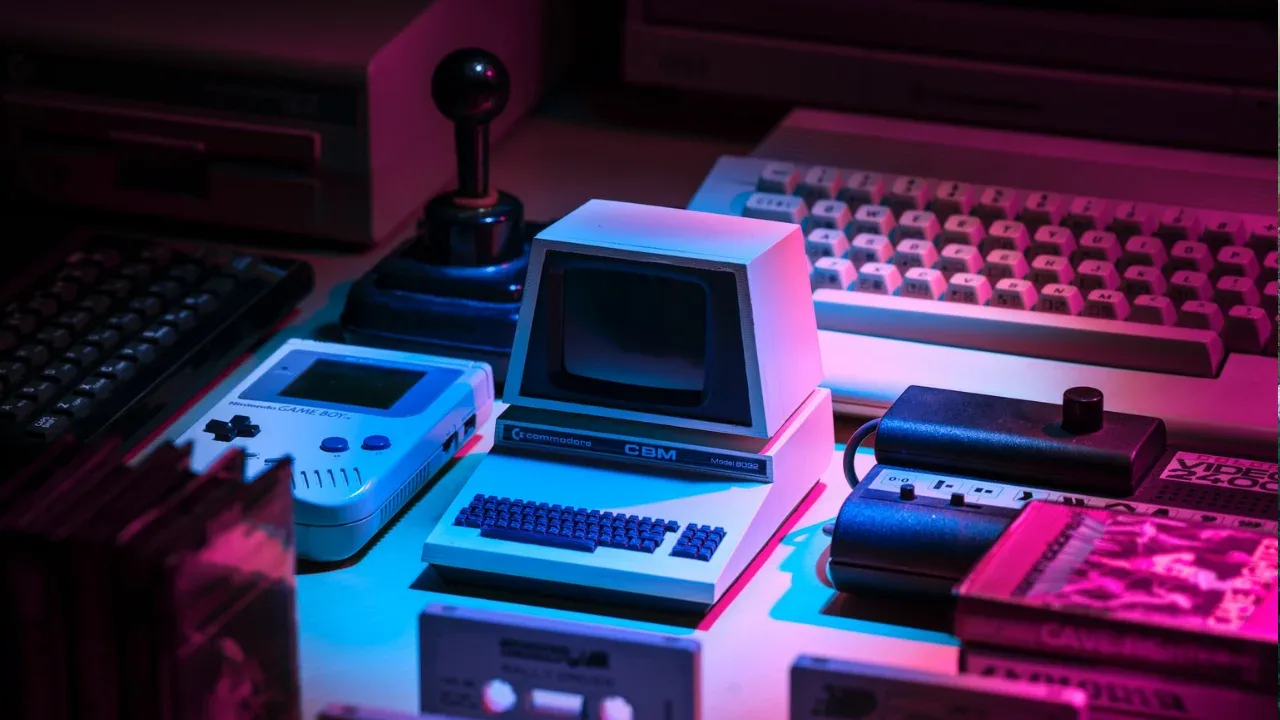
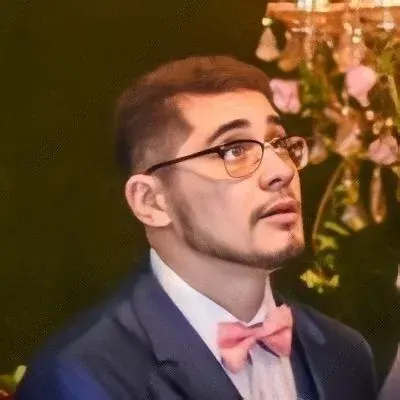
Converting NSString to NSDate (and back again)
š š¬ | #NSDate | #NSString | #Conversion | #TipsAndTricks
Have you ever found yourself in a situation where you needed to convert an NSString
to an NSDate
(and vice versa)? š Fear not! In this blog post, we'll dive into the realm of date conversions and provide you with easy solutions to this common problem. š”
The Problem: Converting NSString to NSDate
Let's start with the first part of the question: converting an NSString
like "01/02/10" (representing 1st February 2010) into an NSDate
. š
By default, NSDateFormatter
is the go-to class for parsing and formatting dates in Objective-C or Swift. Here's the code snippet that achieves this conversion:
let stringDate = "01/02/10"
let dateFormatter = DateFormatter()
dateFormatter.dateFormat = "MM/dd/yy"
let convertedDate = dateFormatter.date(from: stringDate)
That's it! By setting the appropriate date format using dateFormat
, you'll be able to convert the NSString
to an NSDate
. Easy peasy! š
The Solution: Converting NSDate back to NSString
Now, let's move on to the second part of the question: converting the NSDate
back into a string. š
To accomplish this, we'll make use of dateFormatter
again, but this time in the reverse direction. Here's the code snippet to convert NSDate
to NSString
:
let convertedDateString = dateFormatter.string(from: convertedDate ?? Date())
Using the string(from: Date)
method of dateFormatter
, you can convert the NSDate
object back to an NSString
. How cool is that? š
Handling Common Issues
While the above code should work smoothly in most cases, there are a few common issues that you might encounter:
ā¹ļø Unpredictable input formats: If you're dealing with user-generated input, remember to handle various date formats gracefully. Use a more flexible date format like "yyyy-MM-dd'T'HH:mm:ssZ" to accommodate different inputs.
āļø Timezone discrepancies: Keep in mind that
NSDate
objects are timezone-independent. When converting fromNSString
toNSDate
, be sure to clarify the timezone in the date string or adjust it accordingly in your code.
Conclusion
Converting an NSString
to NSDate
(and back again) doesn't have to be a headache anymore! Armed with the knowledge and solutions provided in this blog post, you can confidently handle date conversions in your apps.
Remember, when dealing with date conversions, always pay attention to input format variations and timezone considerations. This will ensure accurate and reliable conversions.
So go ahead, give it a try! Convert those date strings like a pro! š And don't forget to share your experiences or any additional tips in the comments section below. Happy coding! š©āš»šØāš»
š Calling all developers and tech enthusiasts! For more informative tech guides like this, be sure to subscribe to our newsletter and follow us on social media for daily bite-sized tech goodness. Let's learn and grow together! šš”