Check if my app has a new version on AppStore
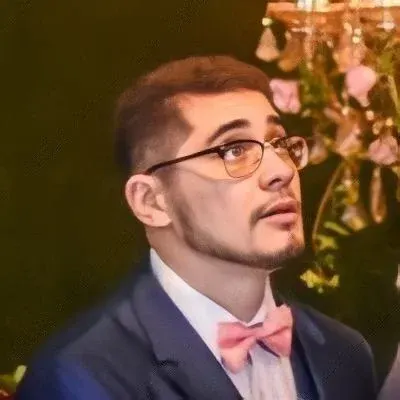
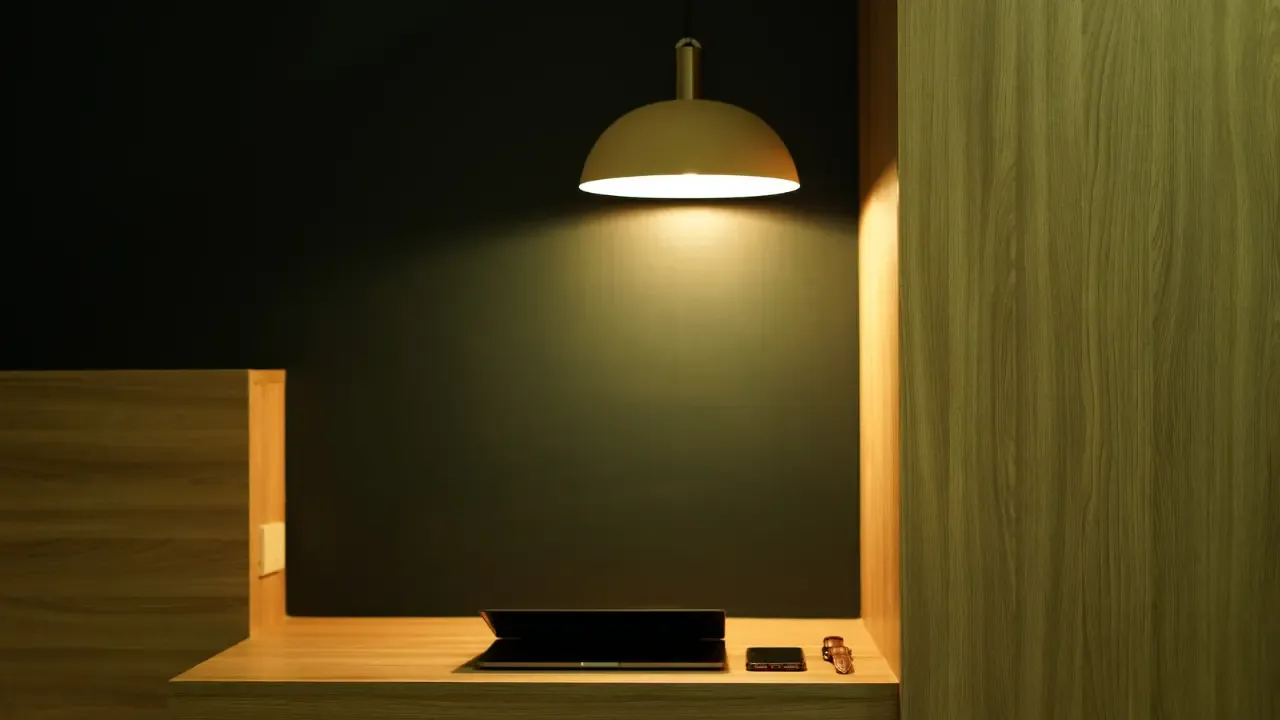
š± How to Check if Your App Has a New Version on AppStore
š Hey there, app developers! š©āš»šØāš» Are you wondering how to manually check if your app has any exciting updates waiting for your users on the AppStore? Look no further! In this guide, I'll show you how to programmatically check your app's version on the AppStore and prompt your users to download the latest and greatest version. Let's dive right in! š„
The Problem:
You have built an awesome app š and want to keep your users up-to-date with the latest features, bug fixes, and enhancements. As a diligent developer, you're considering implementing a way to check if there's a new version available on the AppStore while the user is actively using your app. But how can you make this happen? š¤
The Solution:
Luckily, Apple provides some robust APIs that allow you to programmatically fetch information about your app on the AppStore. By leveraging these APIs, you can compare the version number of your app with the latest version on the AppStore and trigger an update prompt if necessary. Here's how you can get started:
Step 1: Retrieve App Information
To check if there's a new version available, you need to retrieve information about your app from the AppStore. Use the SKStoreProductViewController
class to fetch the current version of your app programmatically. Here's an example using Swift:
import StoreKit
func checkForUpdates() {
let storeViewController = SKStoreProductViewController()
storeViewController.delegate = self
let parameters = [SKStoreProductParameterITunesItemIdentifier: "YOUR_APP_ID"]
storeViewController.loadProduct(withParameters: parameters) { (loaded, error) in
if loaded {
guard let product = storeViewController.productViewController.content as? SKStoreProductViewController else {
return
}
let latestVersion = product.productVersion
let currentVersion = Bundle.main.object(forInfoDictionaryKey: "CFBundleShortVersionString") as? String
// Compare currentVersion with latestVersion and take appropriate action
// e.g., show update prompt or continue with the current version
}
}
}
Make sure to replace "YOUR_APP_ID"
with your actual app's ID.
Step 2: Compare Versions
Now that you have both the current version of your app and the latest version from the AppStore, you can compare them to determine if an update is available. You can use comparison operators such as >
or <
to check if the latest version is higher than the current version. If it is, you can prompt the user to update their app.
Step 3: Trigger an Update Prompt
When an update is available, you can display a custom alert or UI prompt to notify your users about the new version and provide a way for them to download it. You can use Apple's UIAlertController
to create a customized alert or design your own UI elements to fit your app's branding.
Remember to provide clear and concise instructions to guide your users through the update process and ensure a smooth experience.
šÆ Call-to-Action: Engage and Delight Your Users
Congratulations! You now have the power to programmatically check if your app has a new version on the AppStore. By implementing this feature, you can keep your users engaged, informed, and excited about the latest updates to your app.
Go ahead, give it a try! Your users will appreciate being in the know and having access to the newest features and enhancements. Share your success stories and let us know how this feature helps you in the comments below. Happy coding! šš»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
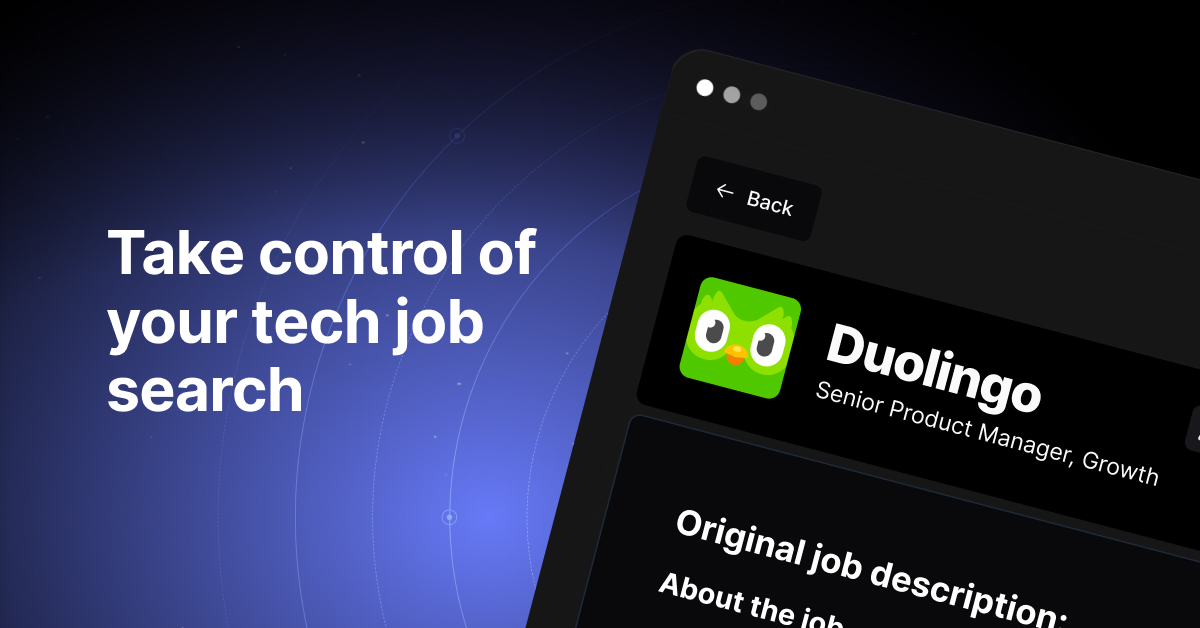