Changing text of UIButton programmatically swift
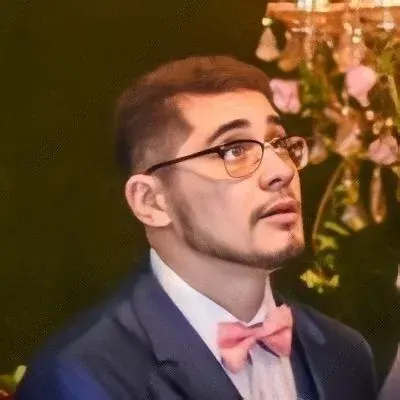
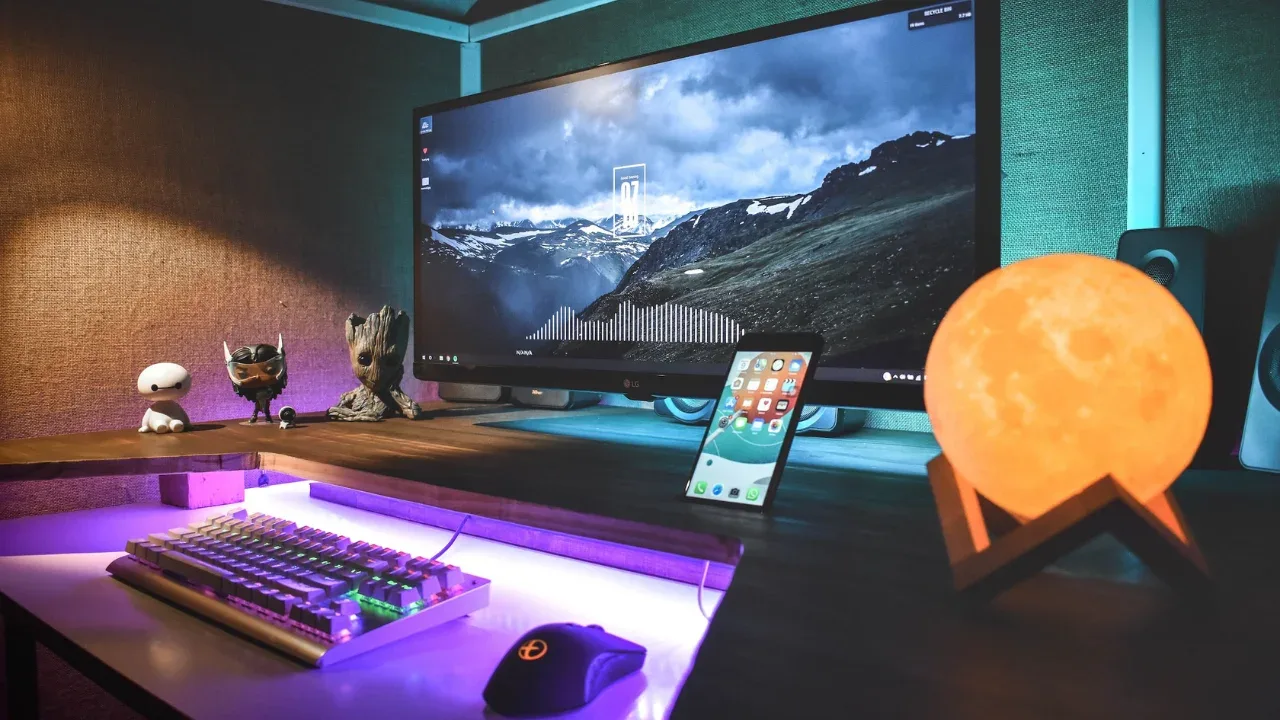
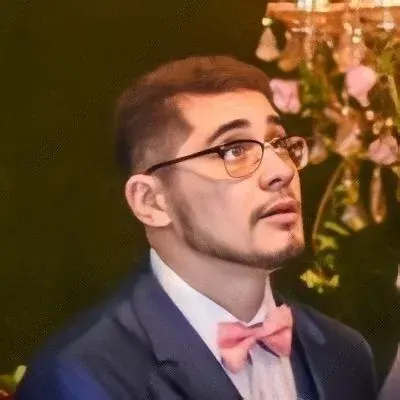
Changing text of UIButton programmatically in Swift
So you want to dynamically change the text of a UIButton in Swift, but you’re facing a roadblock. Don't worry, we’ve got you covered! 🎉
The Problem
The code provided in the question, currencySelector.text = "foobar"
, leads to an "Expected Declaration" error in Xcode. This happens because UIButton doesn't have a text
property, unlike UILabel. But fret not! We have an easy solution for you.
The Solution
To change the text of a UIButton programmatically, we need to use the setTitle(_:for:)
method provided by UIButton. Here's the updated code:
currencySelector.setTitle("foobar", for: .normal)
By using this method, you can specify different titles for different button states, such as .normal
, .highlighted
, and .selected
. In this case, we are setting the title for the normal state.
Example Usage
Here's an example of how you can implement the setTitle(_:for:)
method in your code:
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var currencySelector: UIButton!
override func viewDidLoad() {
super.viewDidLoad()
let newTitle = "foobar"
currencySelector.setTitle(newTitle, for: .normal)
}
}
In the above code snippet, we assume that you have a UIButton IBOutlet named currencySelector
connected to your storyboard or XIB file.
Call-to-Action
You now have the power to dynamically change the text of a UIButton in Swift! 💪 Go ahead and try implementing this code in your project. If you encounter any issues or have any other questions, feel free to leave a comment below. We're always here to help you out!
Remember, learning iOS development is an ongoing journey, so keep exploring and building awesome apps! 🚀