Changing Placeholder Text Color with Swift
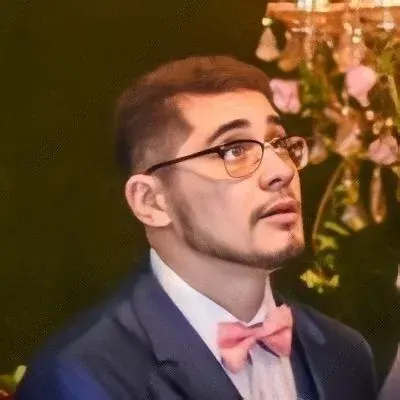
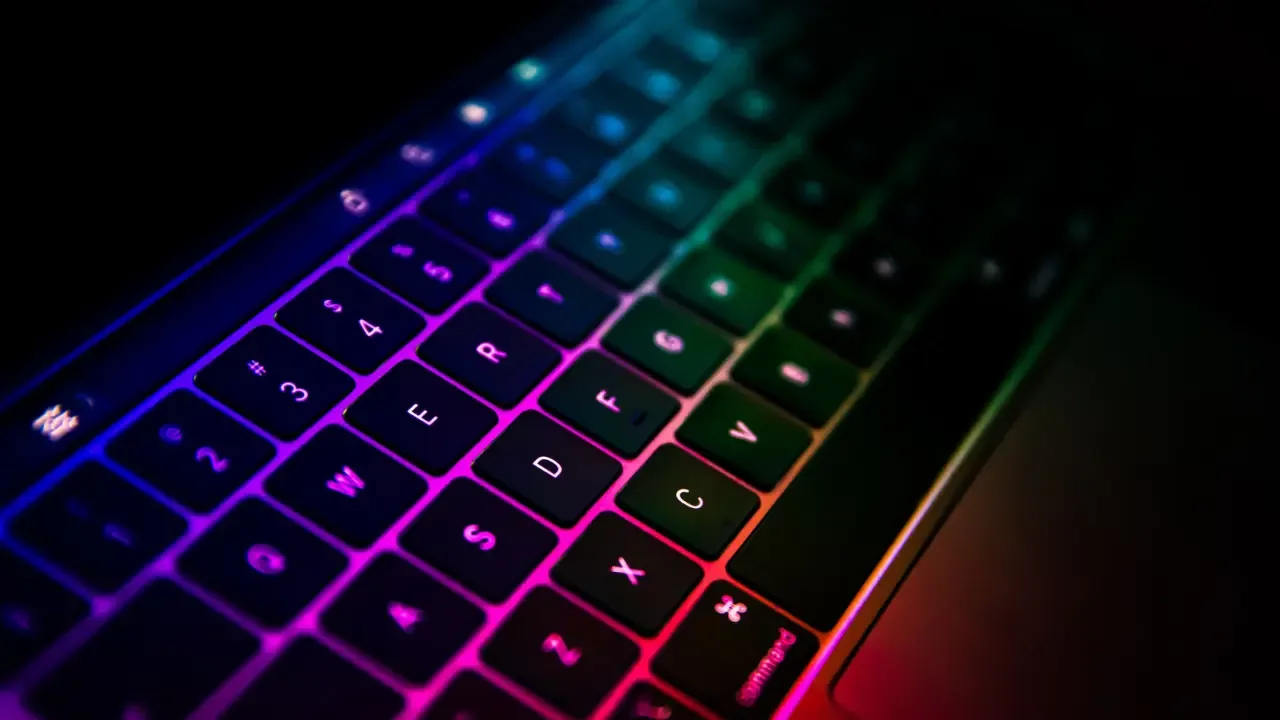
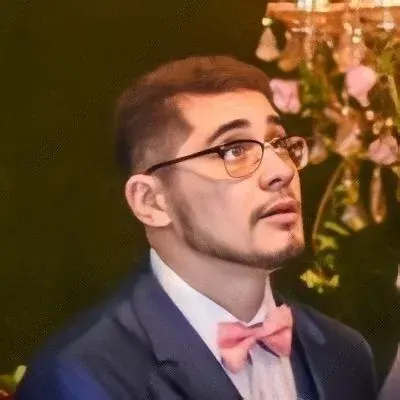
How to Change Placeholder Text Color with Swift
So, you have a beautiful design with a dark blue UITextField
, but there's one problem - the placeholder text is barely visible due to its default dark grey color. 😓 Not to worry, we've got you covered! In this blog post, we'll walk you through step-by-step on how to change the placeholder text color using Swift.
The Problem
Before we dive into the solution, let's understand the problem first. When using Swift and not Objective-C, it can be a bit tricky to find the right solution to change the color of the placeholder text in a UITextField
. But fear not, we'll show you how to do it effectively.
The Solution
To change the placeholder text color in a UITextField
using Swift, follow these simple steps:
Create a subclass of
UITextField
and name it something likeCustomTextField
.
class CustomTextField: UITextField {
}
In the
CustomTextField
class, override thedrawPlaceholder(in rect: CGRect)
method to handle the drawing of the placeholder text.
class CustomTextField: UITextField {
override func drawPlaceholder(in rect: CGRect) {
// Set your desired placeholder text color here
let placeholderColor = UIColor.red
// Set the attributes for the placeholder text
let attributes: [NSAttributedString.Key: Any] = [
.foregroundColor: placeholderColor,
.font: font as Any
]
// Draw the placeholder text with the desired color and attributes
if let placeholder = placeholder {
let placeholderRect = rect.insetBy(dx: 0, dy: (rect.height - font!.pointSize) / 2)
placeholder.draw(in: placeholderRect, withAttributes: attributes)
}
}
}
Now, you can use your
CustomTextField
class wherever you need to change the placeholder text color.
let textField = CustomTextField()
// Customize other properties of the text field as needed
Voila! 🎉 Your placeholder text color is now changed to your desired color.
Going Above and Beyond
Now that you know how to change the placeholder text color, why not take it a step further? Here are a few additional tips to enhance your UI:
Consider using a dynamic placeholder text color that adapts based on the background color or theme.
Experiment with different font styles and sizes for the placeholder text to make it stand out.
Create a reusable extension for
UITextField
that allows you to easily change the placeholder text color without subclassing.
Share Your Success!
We hope this guide helped you solve the challenge of changing the placeholder text color in a UITextField
using Swift. Now it's time for you to shine! Share your success with us by commenting below and let us know how you're using this technique in your own projects. If you have any questions or need further assistance, we're here to help.
Happy coding! 😄🚀