Changing navigation bar color in Swift
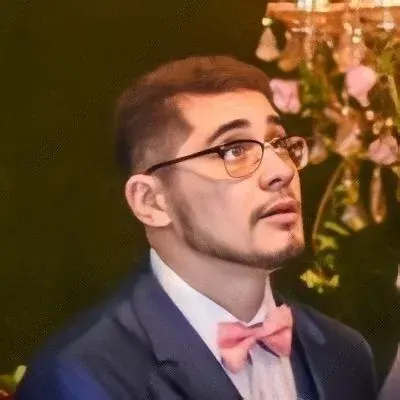
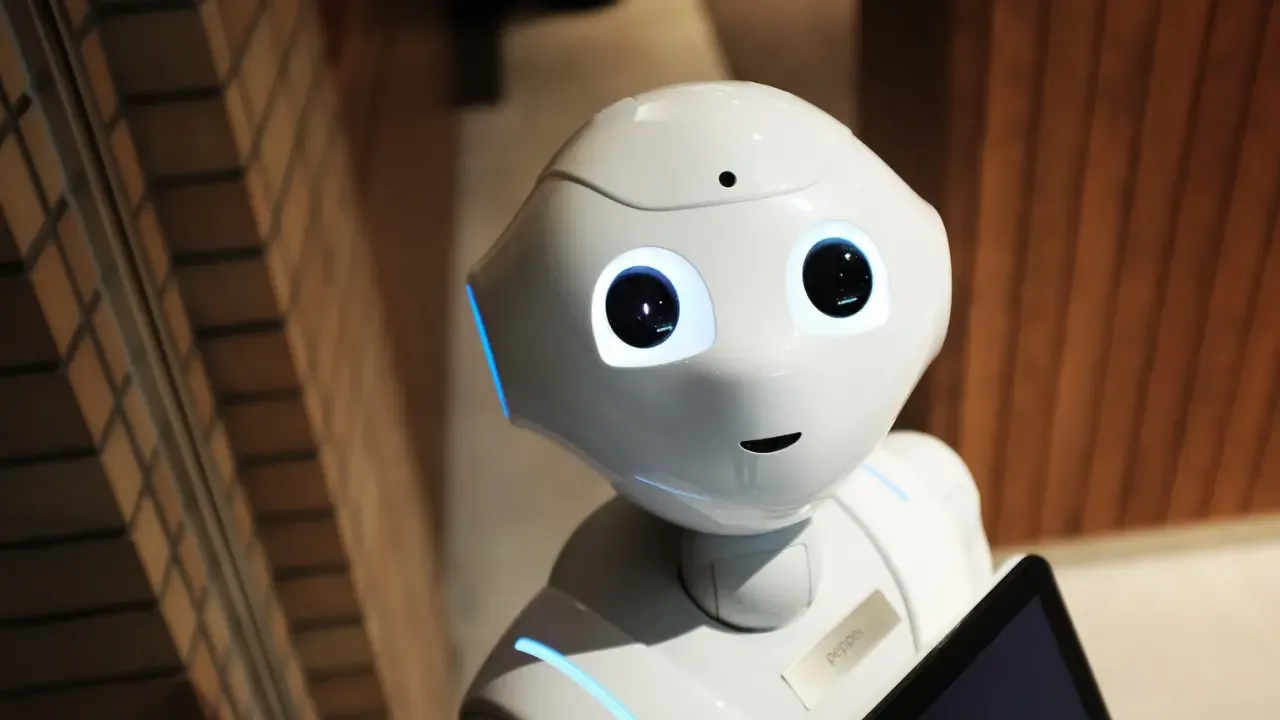
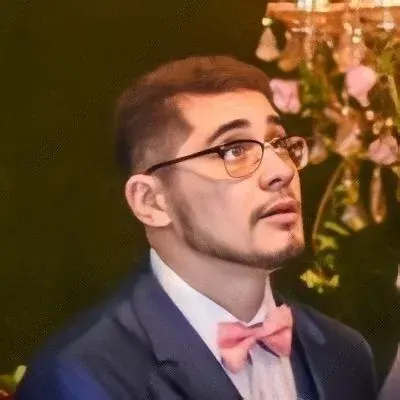
🎨 Changing Navigation Bar Color in Swift 🖌️
Are you looking to add some personal touch to your iOS app? Want to change the navigation bar color and make it match your app's theme? We're here to help! In this blog post, we'll address common issues faced when changing the navigation bar color and provide you with easy solutions in Swift. Let's get started!
🌈 Setting the Navigation Bar Color
To change the color of the navigation bar, we'll need to modify its appearance attributes. Here's an example of how you can do it:
// Inside your view controller's viewDidLoad() method
override func viewDidLoad() {
super.viewDidLoad()
// Change the navigation bar's background color
navigationController?.navigationBar.barTintColor = UIColor.red
// Change the navigation bar's text color
navigationController?.navigationBar.titleTextAttributes = [NSAttributedString.Key.foregroundColor: UIColor.white]
}
In the code snippet above, we're using the barTintColor
property to set the background color of the navigation bar and the titleTextAttributes
property to change the text color. Feel free to experiment with different colors to find the perfect combination that matches your app's design.
🌟 Bonus: Changing Tab Bar Color
If you also want to customize the tab bar color to maintain a consistent theme across your app, it's possible too! Just add the following code to your view controller:
// Inside your view controller's viewDidLoad() method
override func viewDidLoad() {
super.viewDidLoad()
// Change the tab bar's background color
tabBarController?.tabBar.barTintColor = UIColor.blue
}
By using the barTintColor
property of the tabBar
, you can easily modify its background color to ensure it blends beautifully with your navigation bar.
🤓 Engage with Us!
We hope this guide helped you spice up your iOS app's appearance by changing the navigation bar color. Experiment with different colors and have fun creating a unique user experience.
If you have any further questions or need help with other aspects of iOS development, feel free to leave a comment below or reach out to us on our website. We're always here to assist you! 😊
Now go on and make your app stand out with its vibrant navigation bar color!
Note: The code snippets in this blog post are written in Swift. If you're using a different programming language, make sure to adapt the code to your needs.