Can you attach a UIGestureRecognizer to multiple views?
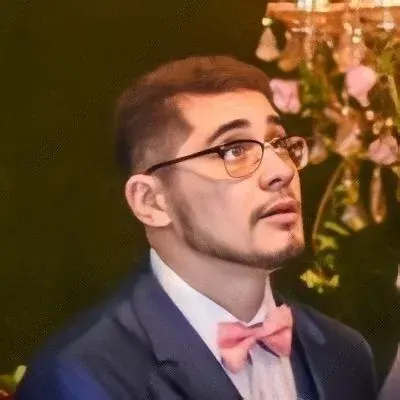
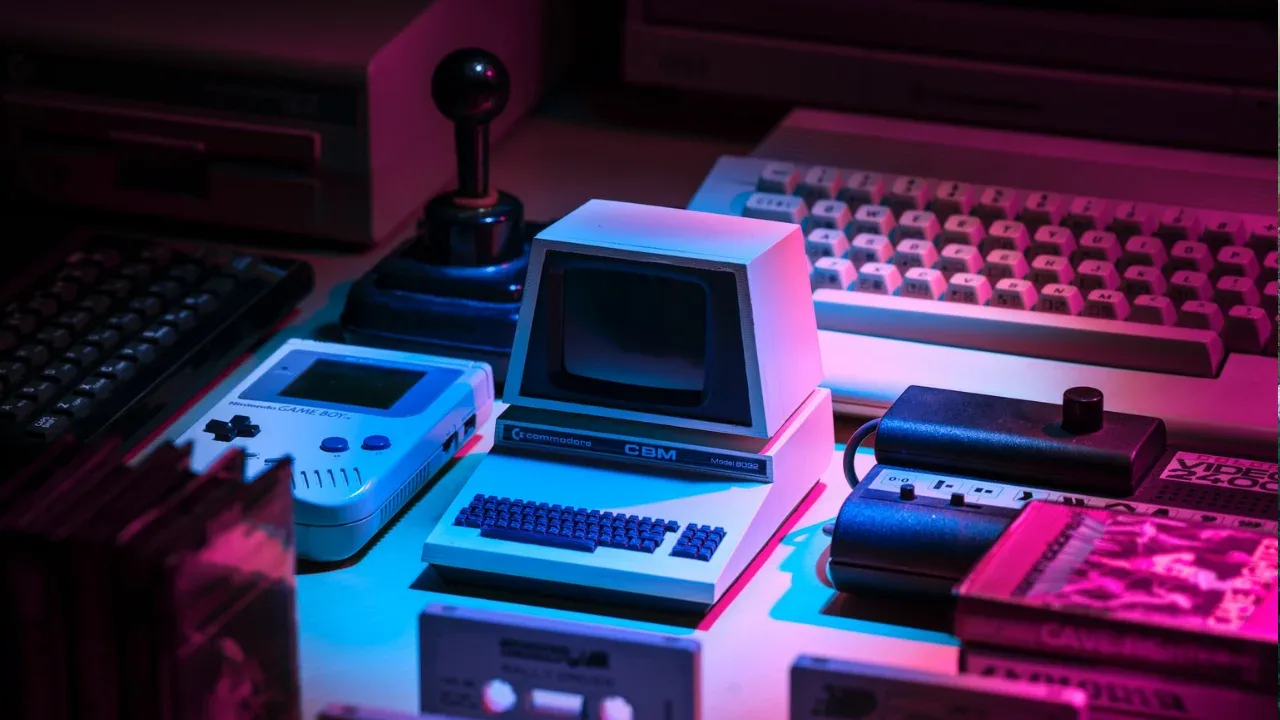
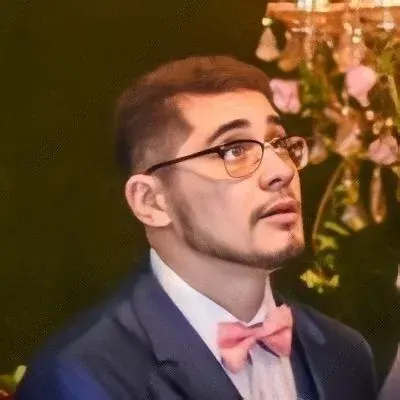
📝 Tech Blog Post: Can you attach a UIGestureRecognizer to multiple views?
Hey tech fam! 👋 Today we're diving into the fascinating world of 🖐️ UI gesture recognizers in iOS development. 📱 Specifically, we'll be addressing a common question: can you attach a UIGestureRecognizer to multiple views? Let's find out! 🕵️♀️
Understanding the Issue
So, here's the scenario: you have two views, let's call them view1
and view2
, and you want to attach a UITapGestureRecognizer to both of them. You'd think that by reusing the same gesture recognizer, you could save some coding time, right? 🤔
Well, here's the catch. In the code example you provided, the UITapGestureRecognizer is added to both view1
and view2
. However, only taps on view2
are recognized. Strange, isn't it? 😕
Exploring the Problem
Before we jump into solutions, let's take a moment to understand why this issue occurs. The problem lies in how UIKit handles gesture recognizers. While it technically allows you to attach a gesture recognizer to multiple views, there's a caveat – a gesture recognizer can only be effectively active for one view at a time. 🤷♂️
In your case, when you attach the same gesture recognizer to both view1
and view2
, UIKit automatically removes it from view1
and reassigns it to view2
. Hence, only taps on view2
are recognized. This behavior is by design, so it's not a bug but rather a feature.
Finding Solutions
Now that we understand the issue, let's explore a couple of practical solutions to attach a gesture recognizer to multiple views:
Solution 1: Create Separate Gesture Recognizers
The simplest solution is to create separate instances of the UITapGestureRecognizer for each view. By doing this, you can attach a unique gesture recognizer to each view, ensuring that taps on both view1
and view2
are recognized. Check out the modified code snippet below:
UITapGestureRecognizer *tapGesture1 = [[UITapGestureRecognizer alloc] initWithTarget:self action:@selector(tapTapTap:)];
[self.view1 addGestureRecognizer:tapGesture1];
[tapGesture1 release];
UITapGestureRecognizer *tapGesture2 = [[UITapGestureRecognizer alloc] initWithTarget:self action:@selector(tapTapTap:)];
[self.view2 addGestureRecognizer:tapGesture2];
[tapGesture2 release];
Now, both view1
and view2
will respond to taps separately, and you can customize their individual gesture recognizer behaviors if needed.
Solution 2: Implement Gesture Recognizer Delegate
Another approach is to leverage the UIGestureRecognizerDelegate
protocol to handle gesture events for both views with a single gesture recognizer. This method requires you to implement the delegate methods and perform manual handling based on the sender (the view that triggered the gesture).
Here's an example of how you can achieve this:
UITapGestureRecognizer *tapGesture = [[UITapGestureRecognizer alloc] initWithTarget:self action:@selector(tapTapTap:)];
tapGesture.delegate = self;
[self.view1 addGestureRecognizer:tapGesture];
[self.view2 addGestureRecognizer:tapGesture];
[tapGesture release];
In your delegate implementation, you can identify the view that triggered the gesture and perform specific actions accordingly. This allows you to attach a single gesture recognizer to multiple views while maintaining control over their responses.
Embrace the Power of Gesture Recognizers! ✨
Congratulations! 🎉 You've just learned how to attach a UIGestureRecognizer to multiple views. Whether you choose to create separate gesture recognizers or leverage the power of the delegate pattern, you now have the knowledge and tools to handle gesture interactions like a pro! 💪
As always, don't hesitate to leave a comment below if you have any questions, other solutions, or want to share your experiences with gesture recognizers. Let's continue our tech conversations and keep learning together! 🤓💬
Happy coding! ✍️🚀