Can you animate a height change on a UITableViewCell when selected?
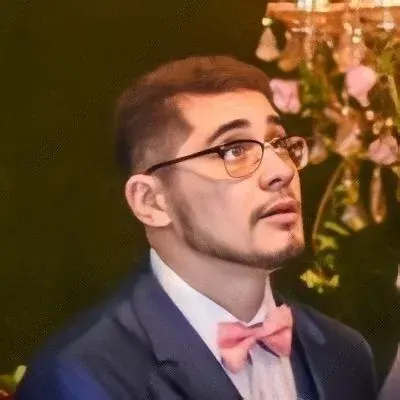
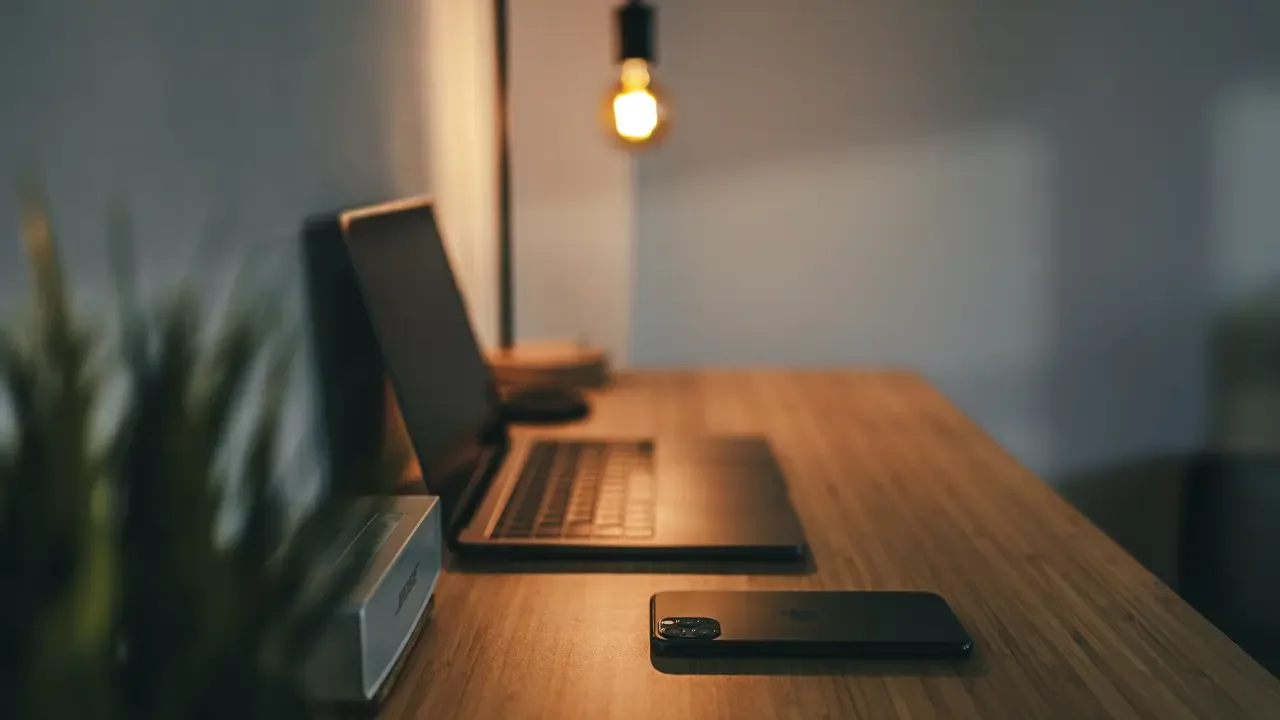
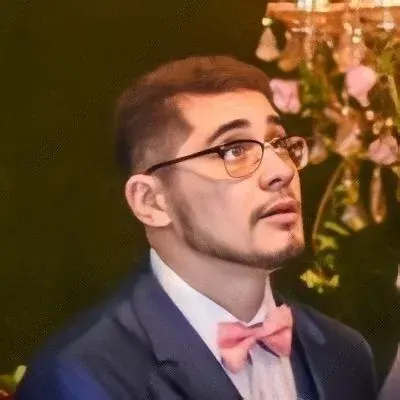
Animating the Height Change on a UITableViewCell When Selected 💥
Are you tired of your UITableView cells being so plain and static? Want to add some fancy animations to your app to make it engaging and delightful for your users? Look no further! In this blog post, I will show you how you can easily animate the height change on a UITableViewCell when selected. Let's dive right in! 🏊♀️📱
The Problem 🤔
You have a UITableView in your iPhone app, and you want to enhance the user experience by making the selected cell grow in height to display additional UI controls for editing the properties of the selected item. However, you're unsure if this is even possible. 😕
The Solution 💡
Step 1: Set Up Your UITableView
First things first, let's set up our UITableView. Create a UITableViewController subclass and override the tableView(_:cellForRowAt:)
method to display your list of people. Each cell should have a unique identifier for easy customization later. Here's an example:
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "PersonCell", for: indexPath)
let person = people[indexPath.row]
cell.textLabel?.text = person.name
return cell
}
Step 2: Implement the Selection Animation
To animate the height change on the selected cell, we can utilize the tableView(_:didSelectRowAt:)
delegate method. This method is called whenever a cell is selected. Inside this method, we'll update the height constraint of the selected cell to achieve the desired animation effect. Here's how you can do it:
override func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
guard let cell = tableView.cellForRow(at: indexPath) else { return }
let expandedHeight: CGFloat = 200 // The desired height of the expanded cell
// Update the height constraint of the selected cell
cell.contentView.heightAnchor.constraint(equalToConstant: expandedHeight).isActive = true
// Animate the cell height change
UIView.animate(withDuration: 0.3) {
tableView.beginUpdates()
tableView.endUpdates()
}
}
Step 3: Reset the Cell Height on Deselection
When the user selects a different cell or taps outside of the selected cell, we need to reset the height of the previously selected cell. We can do this by implementing the tableView(_:willDeselectRowAt:)
delegate method. Here's how you can reset the height:
override func tableView(_ tableView: UITableView, willDeselectRowAt indexPath: IndexPath) -> IndexPath? {
guard let cell = tableView.cellForRow(at: indexPath) else { return indexPath }
// Reset the height constraint of the deselected cell
cell.contentView.heightAnchor.constraint(equalToConstant: UITableView.automaticDimension).isActive = true
// Animate the cell height change
UIView.animate(withDuration: 0.3) {
tableView.beginUpdates()
tableView.endUpdates()
}
return indexPath
}
Call-to-Action ✨
Now that you know how to animate the height change on a UITableViewCell when selected, why not give it a try in your own app? Spice up your user interface and bring your app to life with these engaging animations. Your users will thank you! 😍
If you have any other questions or want to share your creative implementations, leave a comment below! Let's make our apps more dynamic and enjoyable together. Happy coding! 💻🎉