Can I use Objective-C blocks as properties?
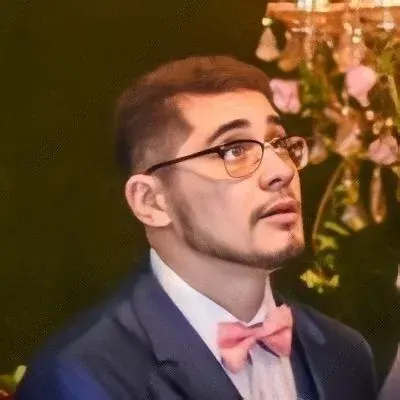
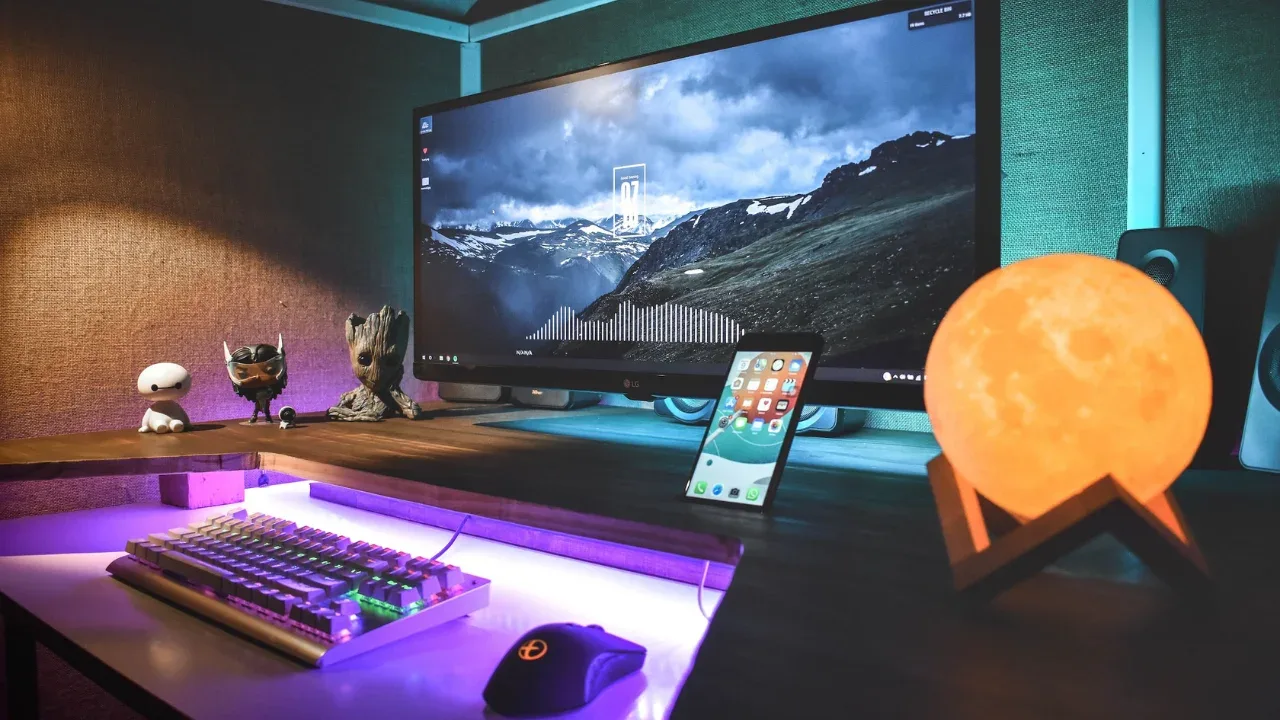
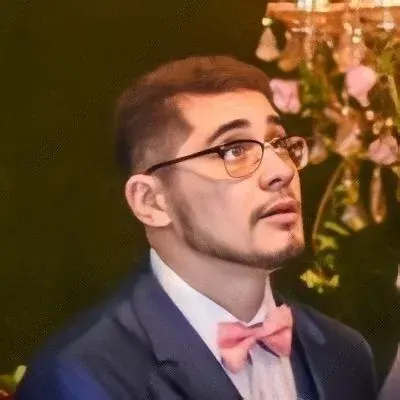
Can I use Objective-C blocks as properties?
Is it possible to have blocks as properties using the standard property syntax? π€ And what about changes in ARC? Let's dive into these questions!
Understanding Objective-C Blocks
Before we dig into the main question, let's quickly understand what Objective-C blocks are. Think of blocks as anonymous functions that capture and encapsulate a piece of code, allowing you to use it later. They are similar to lambda expressions in other programming languages.
Blocks as Properties - The Standard Property Syntax
π‘Yes, you can use Objective-C blocks as properties using the standard property syntax! However, there are a few things you need to consider when doing so.
Property Declaration
To declare a block as a property, you need to use the @property
syntax, just like you would with any other object:
@property (nonatomic, copy) void (^myBlock)(void);
Here, nonatomic
specifies that the property is not thread-safe, and copy
is used to ensure that the block is retained when it is assigned to the property.
Creating and Assigning the Block
To create and assign a block to the property, you can use the block literal syntax:
self.myBlock = ^{
// Your block code here
};
Accessing the Block
To access the block, you treat it like any other property:
self.myBlock();
Memory Management with ARC
When using Automatic Reference Counting (ARC), there are a couple of additional considerations when working with blocks as properties.
Weak References
If the block captures a strong reference to self
, it can lead to a strong reference cycle, causing a memory leak. To avoid this, you should use a weak reference to self
within the block:
__weak typeof(self) weakSelf = self;
self.myBlock = ^{
typeof(self) strongSelf = weakSelf;
// Use strongSelf inside the block to avoid a retain cycle
};
Copying Blocks
When using ARC, assigning a block to a property with the copy
attribute creates a copy of the block, ensuring that it is retained correctly:
@property (nonatomic, copy) void (^myBlock)(void);
Wrapping It Up
β
You can indeed use Objective-C blocks as properties using the standard property syntax. Just remember to use the @property
syntax and handle memory management correctly, especially when using ARC. π
If you want to dive deeper into blocks or have any questions, feel free to reach out in the comments section below. Happy coding! π»π