Array from dictionary keys in swift
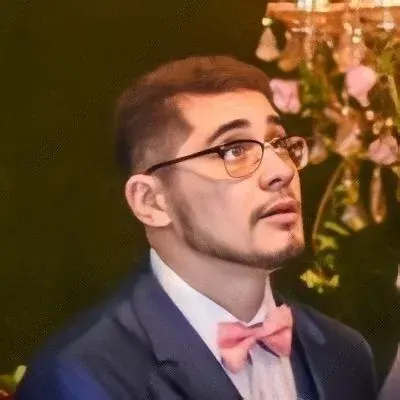
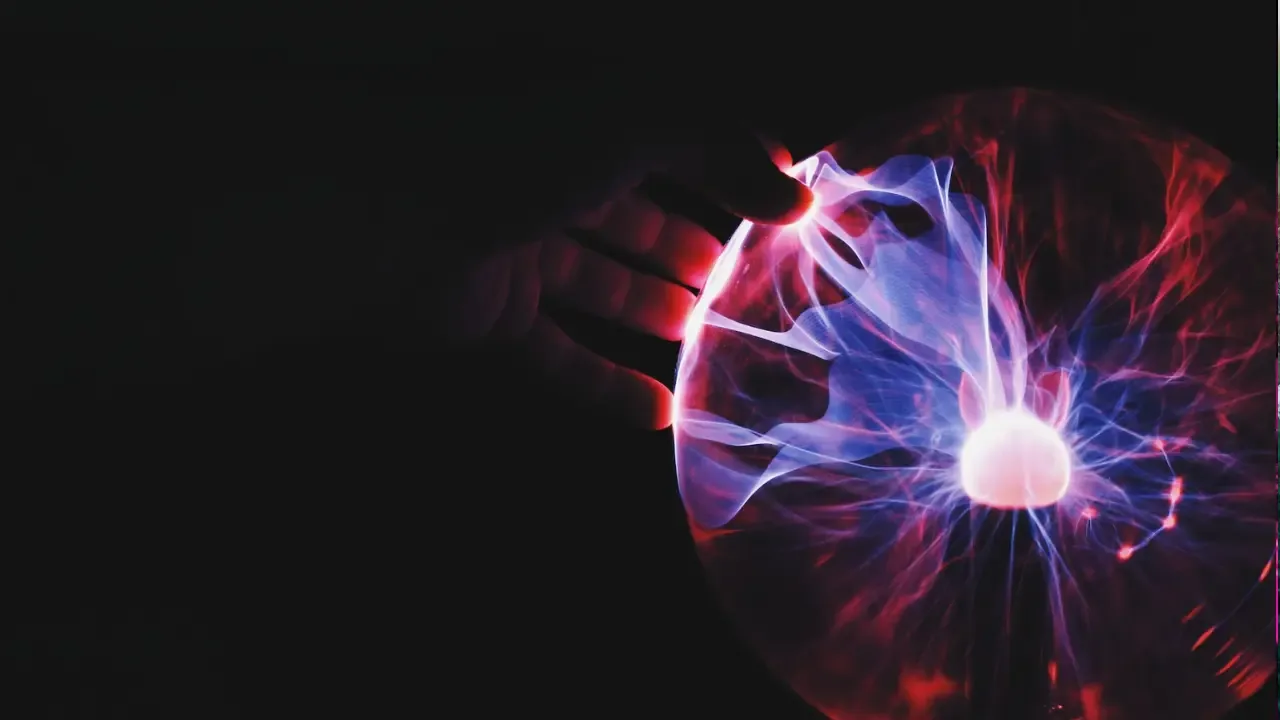
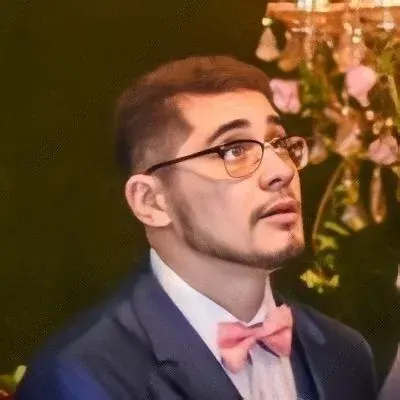
Easy Peasy Lemon Squeezy: Array from Dictionary Keys in Swift
Hey there, tech enthusiast! ๐ Are you trying to fill an array with strings from the keys in a dictionary in Swift? Don't worry, we've got your back! In this blog post, we'll dive into common issues, provide easy solutions, and boost your Swift game to a whole new level. Let's get started! ๐
The Problem
So, you've got a dictionary and you want to create an array by extracting the keys. Seems easy, right? Well, not always. Let's take a look at the code snippet you provided:
var componentArray: [String]
let dict = NSDictionary(contentsOfFile: NSBundle.mainBundle().pathForResource("Components", ofType: "plist")!)
componentArray = dict.allKeys
Unfortunately, when you try to compile the code, you'll encounter the dreaded 'AnyObject' not identical to String
error. ๐ฑ Trust us, you're not alone in facing this issue. But fear not! We've got some nifty solutions up our sleeves. ๐ช
Solution 1: Casting with as?
One way to resolve this issue is by using the as?
operator for conditional casting. By doing this, you can safely attempt to convert each key into a String
. If the conversion succeeds, you'll have your desired array.
if let dictKeys = dict.allKeys as? [String] {
componentArray = dictKeys
// Voilร ! You have your array of strings from dictionary keys!
} else {
// Oops! Something went wrong during the casting process ๐
// Handle the situation gracefully or display an error message
}
With the conditional casting, you can gracefully handle situations where the dictionary keys can't be casted into strings. It's always better to handle potential errors so your code doesn't go haywire. ๐งน
Solution 2: Type Inference with compactMap
Another nifty way to achieve your goal is by using the compactMap
function provided by Swift's array type.
componentArray = dict.allKeys.compactMap({ $0 as? String })
By using compactMap
, you're able to map over each key and filter out any keys that fail the conditional cast. This way, you'll end up with an array containing only the successfully casted keys. Pretty neat, huh? ๐
The Call-to-Action
Now that you have the tools to extract an array from dictionary keys like a pro, it's time to put your newfound knowledge into practice! Try implementing these solutions in your code and let us know how it goes. We're excited to hear about your success stories! ๐ฅ
Remember, sharing is caring! If you found this blog post helpful, make sure to share it with your fellow Swift enthusiasts. Let the tech world unite and conquer these coding challenges together! ๐
Until next time, happy coding! ๐ค๐ป