Always pass weak reference of self into block in ARC?
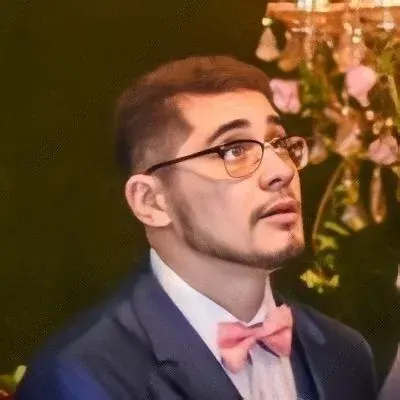
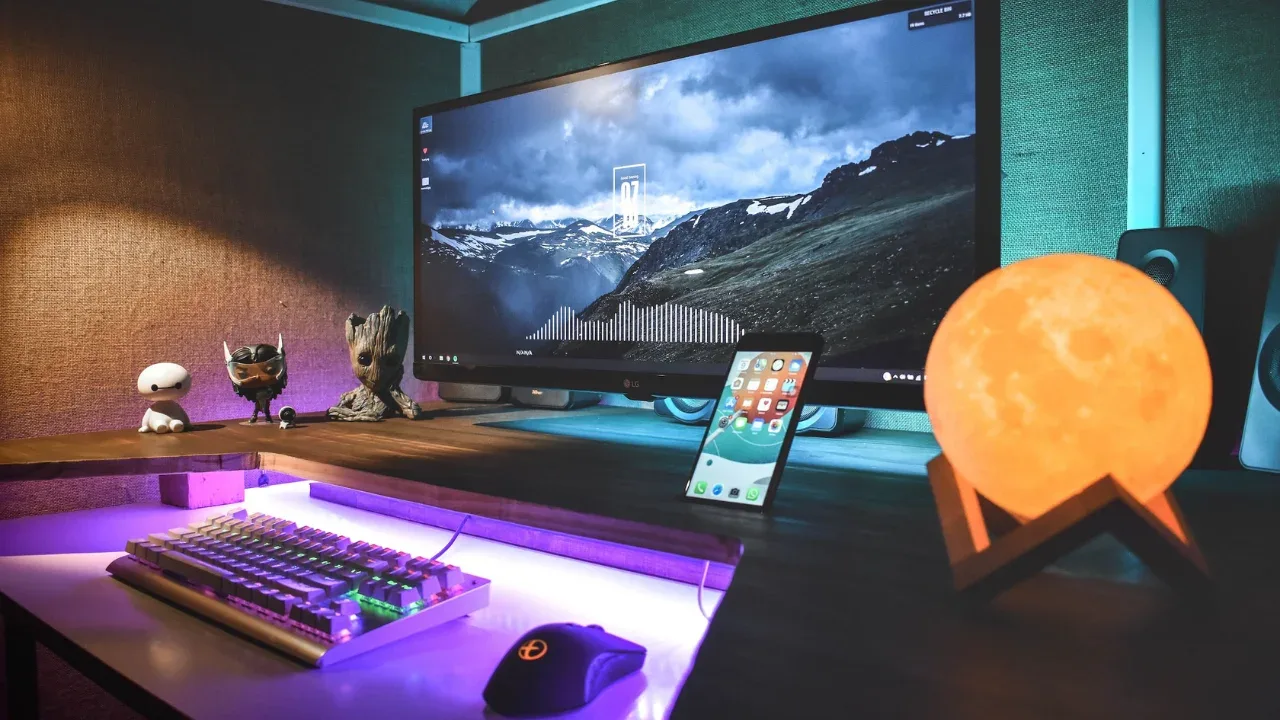
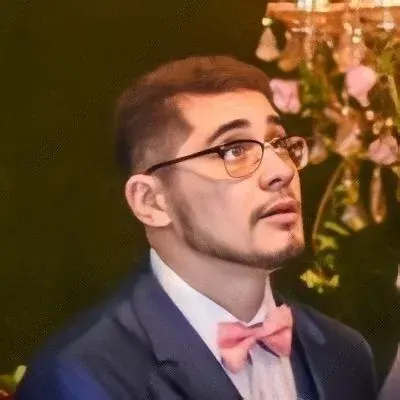
đĄ Understanding Block Usage in Objective-C with ARC
Are you feeling a little bit confused about block usage in Objective-C? đ¤ Don't worry, you're not alone! In this blog post, we'll address a common issue related to blocks and provide easy solutions to ensure your code works smoothly with ARC (Automatic Reference Counting).
đ§Š The Problem: Blocks Retaining Self
Let's start by diving into the specific problem mentioned in the context. The question revolves around always referring to self
instead of its weak reference when using blocks in an ARC-enabled environment. The concern is whether this practice could cause blocks to retain self
and prevent it from being deallocated.
To illustrate the issue, let's take a look at the sample code:
-(void)handleNewerData:(NSArray *)arr
{
ProcessOperation *operation =
[[ProcessOperation alloc] initWithDataToProcess:arr
completion:^(NSMutableArray *rows) {
dispatch_async(dispatch_get_main_queue(), ^{
[self updateFeed:arr rows:rows];
});
}];
[dataProcessQueue addOperation:operation];
}
In this example, we have a handleNewerData
method that creates a ProcessOperation
object. Within the closure passed as the completion
parameter, we dispatch an asynchronous task using dispatch_async
. However, notice that we are referencing self
inside the closure.
đĢ The Retain Cycle Conundrum
When you reference self
inside a block, it creates a strong reference to self
, which in turn could cause a retain cycle. A retain cycle occurs when two or more objects hold strong references to each other, preventing them from being deallocated even when they are no longer needed. This can lead to memory leaks and inefficient memory usage in your app. đą
To break this retain cycle and allow self
to be deallocated properly, we need to use a weak reference to self
inside the block.
â The Solution: Always Pass Weak Reference of Self
The answer to the question is a resounding YES đ! You should always pass a weak reference to self
inside a block to prevent retain cycles and ensure proper memory management. By using a weak reference, the block maintains a non-retaining reference to self
, allowing it to be deallocated when no longer needed.
Let's update the code example to include a weak reference to self
:
-(void)handleNewerData:(NSArray *)arr
{
__weak typeof(self) weakSelf = self;
ProcessOperation *operation =
[[ProcessOperation alloc] initWithDataToProcess:arr
completion:^(NSMutableArray *rows) {
__strong typeof(weakSelf) strongSelf = weakSelf;
if (strongSelf) {
dispatch_async(dispatch_get_main_queue(), ^{
[strongSelf updateFeed:arr rows:rows];
});
}
}];
[dataProcessQueue addOperation:operation];
}
In this updated code, we added a weak reference to self
using the __weak
qualifier. Then, we created a strong reference to the weak reference using the __strong
qualifier. This strong reference is necessary to avoid potential crashes if self
becomes deallocated during the block execution.
đŖ Call-To-Action: Improve Your Block Usage Now!
Now that you know the importance of using a weak reference to self
inside blocks, it's time to put this knowledge into practice! Here's a quick three-step guide to help you improve your block usage with ARC:
Identify blocks in your code that reference
self
.Add a weak reference to
self
before the block declaration using__weak typeof(self) weakSelf = self
.Create a strong reference to the weak reference inside the block using
__strong typeof(weakSelf) strongSelf = weakSelf
, and usestrongSelf
instead ofself
within the block.
By following these steps, you can ensure proper memory management and prevent retain cycles caused by block usage.
âšī¸ Remember, block usage can be challenging, but with proper understanding and implementation of weak references, you can avoid memory issues and enhance the performance of your app. đĒ
So go ahead and update those blocks in your code. Your app's memory will thank you! đ
If you found this blog post helpful, make sure to share it with your fellow developers so they can also benefit from these tips. đĨđŦ
Have any questions or suggestions related to block usage or memory management? Let's discuss in the comments below! Let's strengthen our coding skills together! đđģđĨ