Add placeholder text inside UITextView in Swift?
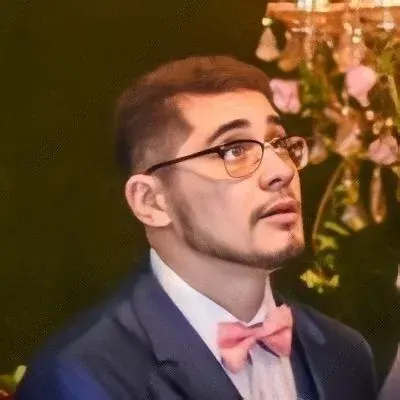
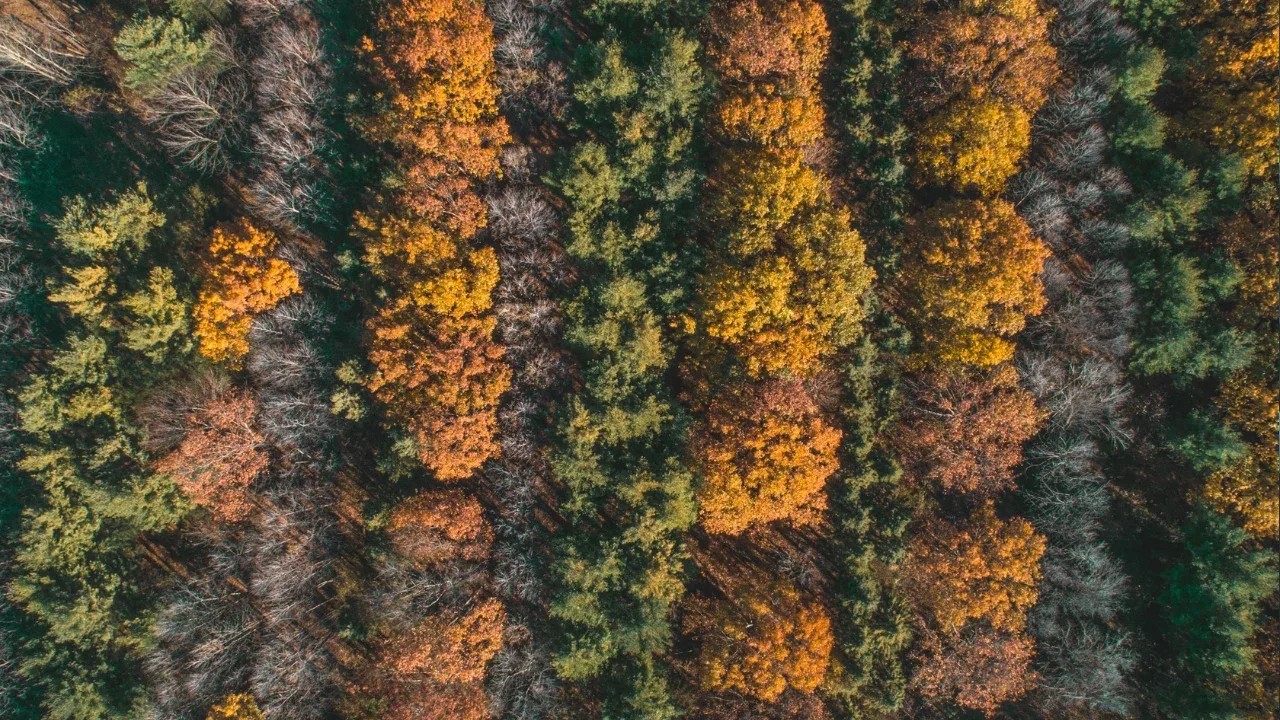
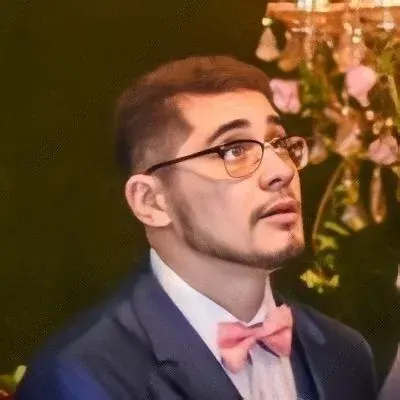
đđĨī¸ Blog Post: How to Add Placeholder Text Inside UITextView in Swift? đđĨī¸
Hey there, Swift developers! đ
Are you puzzled about adding a placeholder text in a UITextView, just like you can in a UITextField? đ Don't worry, because I've got you covered! In this blog post, we'll explore the common issues faced and provide you with easy solutions to implement a placeholder text inside a UITextView using Swift. So let's dive in and make your UITextView user-friendly! đ
đ The Problem: The UITextView class doesn't have a built-in property to set a placeholder text, similar to UITextField's placeholder property. This can be frustrating when you want to guide your users on what to input in the text view.
đĄ Easy Solutions: 1ī¸âŖ Option 1: Subclassing UITextView: One approach is to create a custom subclass of UITextView that includes a placeholder functionality. Here's an example implementation:
class PlaceholderTextView: UITextView {
let placeholderLabel = UILabel()
override var text: String! {
didSet {
placeholderLabel.isHidden = !text.isEmpty
}
}
override func awakeFromNib() {
super.awakeFromNib()
setupPlaceholderLabel()
}
private func setupPlaceholderLabel() {
placeholderLabel.textColor = .lightGray
placeholderLabel.numberOfLines = 0
addSubview(placeholderLabel)
// Set your desired constraints for the placeholder label
// ...
}
}
With this subclass, you can now set the placeholder text by using the placeholderLabel.text
property. The placeholder label will be hidden whenever text is present.
2ī¸âŖ Option 2: UITextView Extension: If subclassing feels excessive for your needs, you could consider using an extension instead. Here's an example of an UITextView extension that adds a placeholder:
extension UITextView {
func addPlaceholder(_ placeholder: String) {
let placeholderLabel = UILabel()
placeholderLabel.text = placeholder
placeholderLabel.textColor = .lightGray
placeholderLabel.numberOfLines = 0
placeholderLabel.sizeToFit()
addSubview(placeholderLabel)
sendSubviewToBack(placeholderLabel)
// Set your desired constraints for the placeholder label
// ...
}
}
To utilize the extension, simply call addPlaceholder(_:)
on your UITextView instance and pass in the desired placeholder text.
đšī¸ Call-to-Action: Now that you have two easy solutions to implement a placeholder text inside a UITextView, it's time to give them a try! Choose the approach that suits your needs best and start making your UITextView more user-friendly today. đĒ
đĨ Share your Experience: Have you faced the placeholder text issue in UITextView before? Which solution did you find most helpful? Share your thoughts and experiences in the comments below! Let's learn and grow together as a community. đ
That's all for today's blog post! Hope you found this guide useful and now feel confident while adding a placeholder text inside a UITextView using Swift. If you enjoyed this article, don't forget to share it with your fellow Swift enthusiasts. Happy coding! đ
[blog image]
đâī¸ Subscribe: Want to receive more helpful guides and tutorials straight to your inbox? Don't forget to subscribe to our newsletter and be the first to know about our latest content.
Keep coding and stay tuned for more awesome Swift tips and tricks! đ
Until next time, Your Name