Add an element to an array in Swift
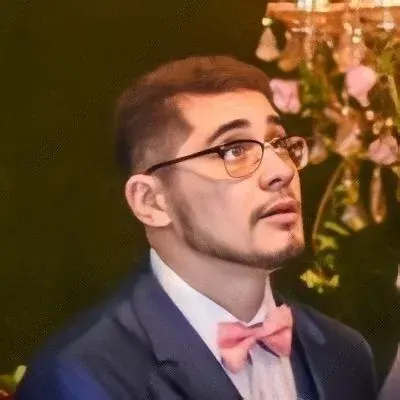
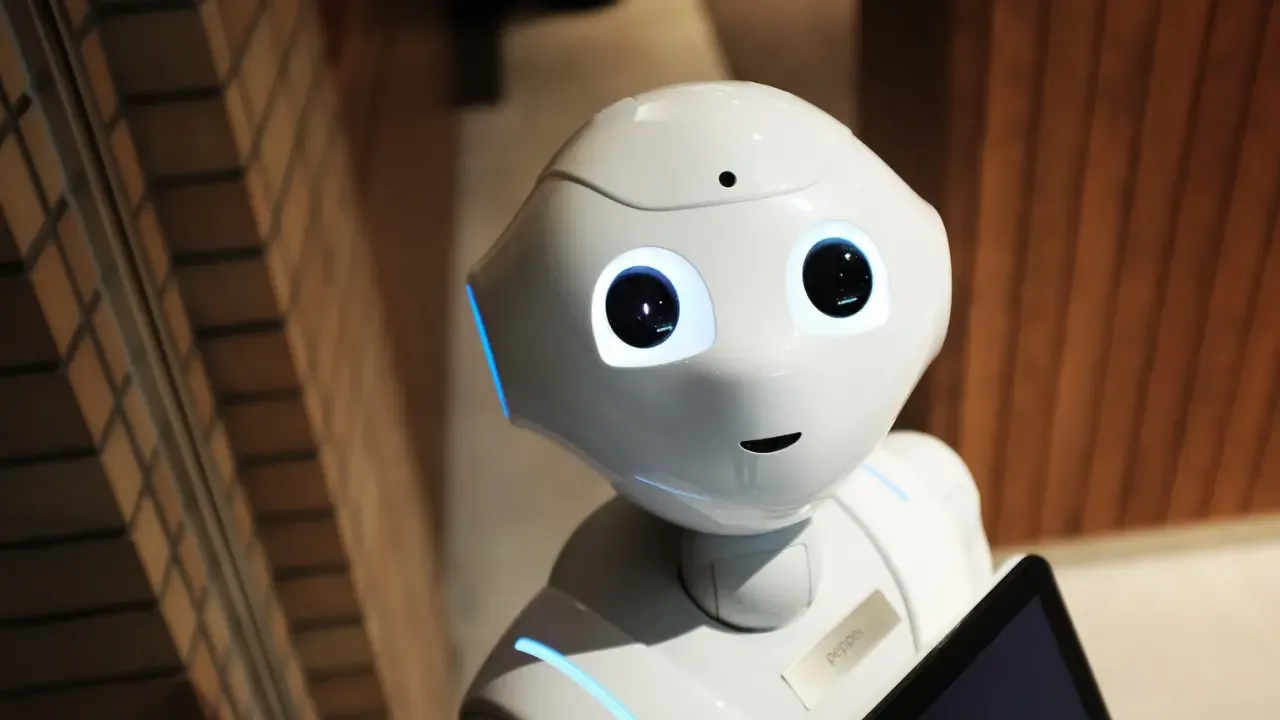
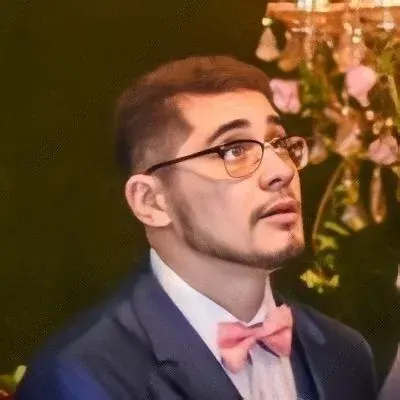
Adding an Element to an Array in Swift 📚
So you have an array in Swift, and you want to add an element to it. 🤔 Whether you need to append an element to the end of the array or insert it at the front, we've got you covered! In this guide, we'll tackle both scenarios and provide you with easy solutions.
📥 Appending an Element to the End
Let's start with the case where you want to add an element to the end of the array. Using the given array:
var myArray = ["Steve", "Bill", "Linus", "Bret"]
You want to push/append an element to the array, resulting in:
["Steve", "Bill", "Linus", "Bret", "Tim"]
The method you are looking for is the append
method. It allows you to add an element to the end of an array. 💪
Here's how you can use it:
myArray.append("Tim")
That's it! 🙌 The element "Tim" has been successfully added to the end of your array.
📌 Inserting an Element at the Front
Now, what if you want to add an element to the front of the array? You might be wondering if there's a constant time unshift
method like in some other programming languages. Unfortunately, there isn't a direct equivalent method in Swift.
However, you can achieve the same result by using the insert(_:at:)
method. This method allows you to insert an element at a specific index in the array.
Here's an example:
myArray.insert("John", at: 0)
In this case, "John" will be added at index 0, shifting all existing elements to the right:
["John", "Steve", "Bill", "Linus", "Bret"]
Voila! 🎉 You just added an element to the front of your array.
🎯 Take Action and Level Up!
Now that you know how to add an element to an array in Swift, it's time to put your new knowledge into action! ✨
Try implementing these methods in your Swift projects and see how they can enhance your code. Experiment, explore, and keep building awesome things with Swift. 🚀
And don't forget to share your experiences and any cool tricks you discover along the way! Let's continue the conversation in the comments below. 👇
Happy coding! 😊💻