How to pass url arguments (query string) to a HTTP request on Angular?
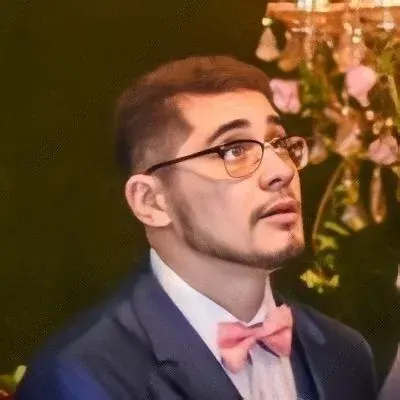
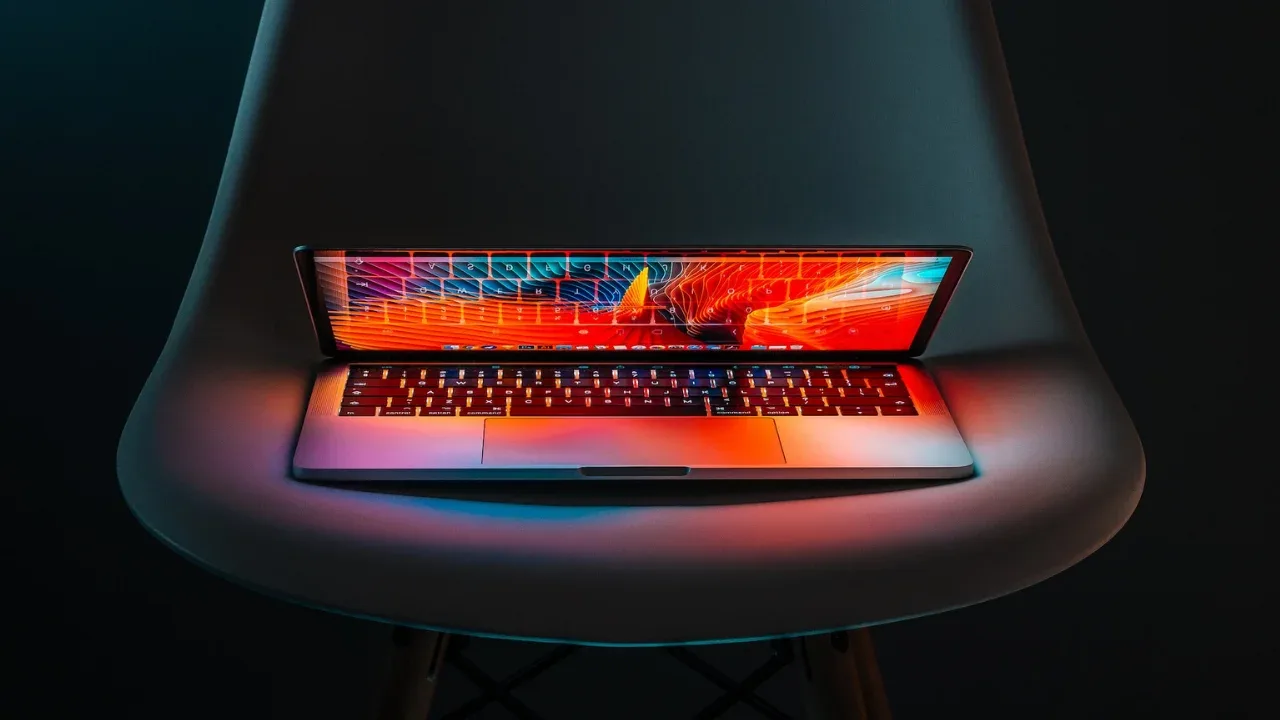
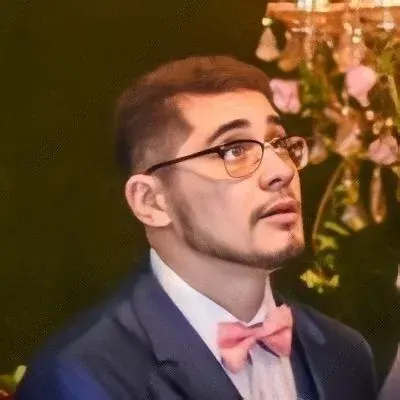
How to Pass URL Arguments (Query String) to an HTTP Request on Angular? π
So you want to trigger an HTTP request from an Angular component, but you're not sure how to add URL arguments (query string) to it? No worries, I've got you covered! πͺ
Let's take a look at the code snippet you provided as an example:
this.http.get(StaticSettings.BASE_URL).subscribe(
(response) => this.onGetForecastResult(response.json()),
(error) => this.onGetForecastError(error.json()),
() => this.onGetForecastComplete()
)
In this example, the StaticSettings.BASE_URL
is a URL without a query string, like http://atsomeplace.com/
. But you want it to be like http://atsomeplace.com/?var1=val1&var2=val2
. So, how do you add var1
and var2
to your HTTP request object as an object? π€
Solution: Adding URL Arguments as an Object π
To achieve this, you can make use of the HttpParams
class provided by Angular's HttpClient
module. Here's how you can modify your code to pass URL arguments as an object:
import { HttpClient, HttpParams } from '@angular/common/http';
// ...
const params = new HttpParams()
.set('var1', 'val1')
.set('var2', 'val2');
this.http.get(StaticSettings.BASE_URL, { params }).subscribe(
(response) => this.onGetForecastResult(response.json()),
(error) => this.onGetForecastError(error.json()),
() => this.onGetForecastComplete()
);
By creating a new instance of HttpParams
, you can chain the set()
method to add the desired URL arguments (query string parameters) as key-value pairs. In this case, var1
and var2
are set to val1
and val2
respectively.
Then, you can pass the params
object as an option in the get()
function of the HttpClient
module. This will attach the URL arguments to your HTTP request, and the HttpClient
module will handle parsing them into a URL query string for you. πͺ
Time to Test it Out! π
Now that you've learned how to pass URL arguments to an HTTP request in Angular, it's time to give it a go! Update your code with the solution provided above and test it out.
If you encounter any issues or have any questions, feel free to drop a comment below. I'm here to help! π¬
Take it to the Next Level! πͺ
Passing URL arguments is just one of the many cool things you can do with Angular's HttpClient
module. If you're interested in learning more about handling HTTP requests in Angular, don't miss out on these engaging blog posts:
"GET, POST, PUT, DELETE: How to Handle Different HTTP Methods in Angular" π
"Handling Error Responses in Angular's HTTP Requests Like a Pro" π
"How to Implement HTTP Interceptors in Angular for Fun and Profit" π
Check them out, level up your Angular skills, and join the league of Angular superheroes! π¦ΈββοΈπ¦ΈββοΈ
Remember, the journey to becoming an Angular expert is full of exciting challenges. Embrace them, learn from them, and keep coding! π
Now go forth, my fellow developer, and conquer the world of HTTP requests in Angular! ππ₯