JQuery: "Uncaught TypeError: Illegal invocation" at ajax request - several elements
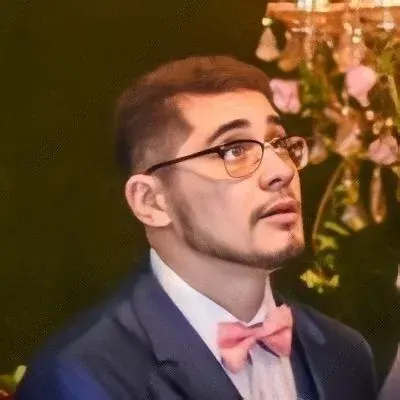
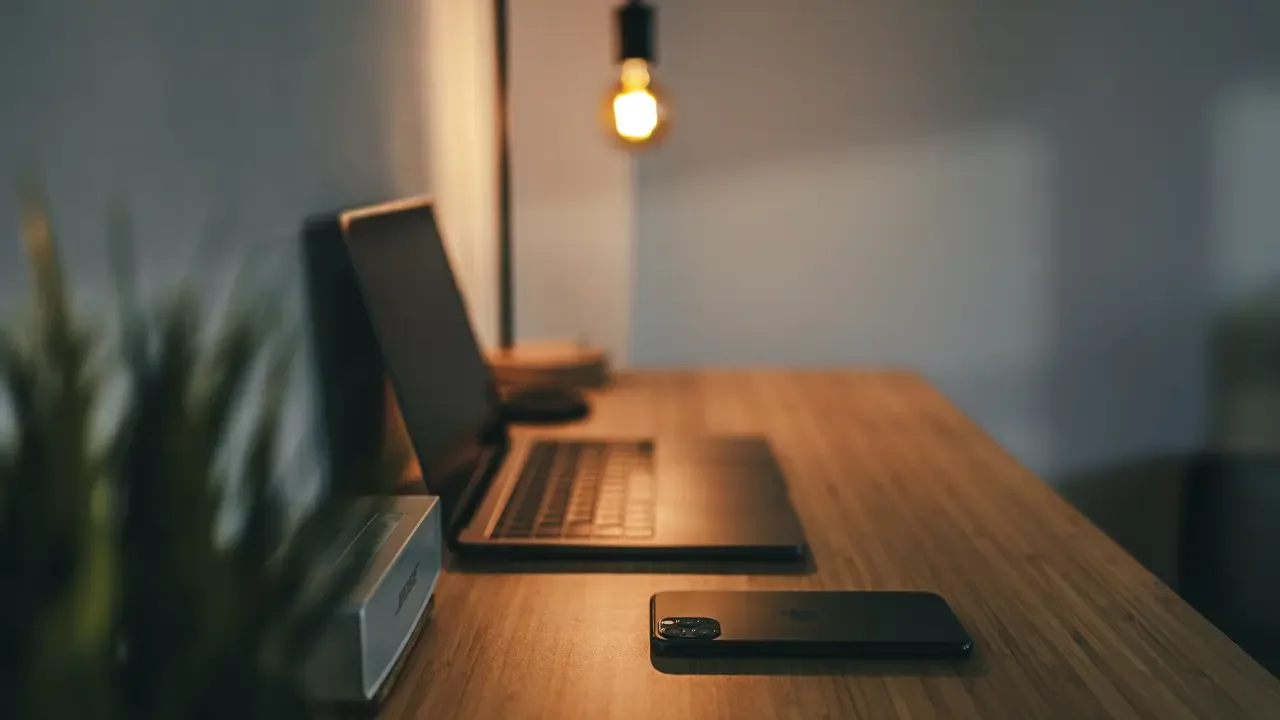
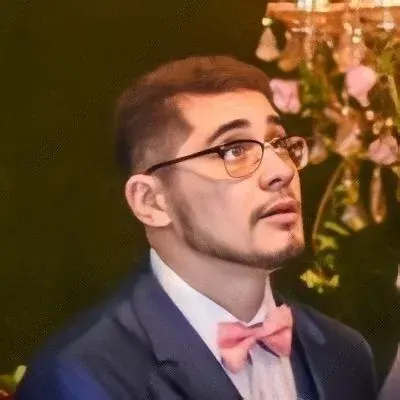
jQuery: 'Uncaught TypeError: Illegal invocation' at ajax request - several elements
Are you encountering the pesky 'Uncaught TypeError: Illegal invocation' error while working with jQuery's ajax request? Don't fret! We've got you covered. In this article, we'll dive into the common issues surrounding this error and provide you with easy solutions to overcome it. Let's get started!
Understanding the problem
The 'Uncaught TypeError: Illegal invocation' error usually occurs when trying to pass an object or an array as the data parameter in the jQuery ajax request. In your case, you are experiencing this error while trying to update the options of element B based on the selected option of element A.
The culprit
Let's examine the code snippet causing the trouble:
var data = {
'mode': 'filter_city',
'id_A': e[e.selectedIndex]
};
do_ajax(city_sel, data, 'ajax_handler.php');
In this code, you have defined the data parameter as an object, which contains key-value pairs. However, passing this object directly to the ajax request results in the 'Uncaught TypeError: Illegal invocation' error.
The solution
To overcome this error, you can simply convert the data object to a string format. Here's how you can do it:
var data = 'mode=filter_city&id_A=' + e[e.selectedIndex];
By converting the data object to a string, you can avoid the 'Uncaught TypeError' error. However, you mentioned that you need the data to be in array format for your server-side PHP code. How can we achieve that? Let's find out!
Converting data object to an array
To send the data as an associative array to the server-side, you can make use of the jQuery.param()
function. This function serializes the data object into a URL-encoded string, which can be easily parsed as an associative array by your PHP code. Here's an example:
var data = {
'mode': 'filter_city',
'id_A': e[e.selectedIndex]
};
data = jQuery.param(data);
By using jQuery.param()
, you can convert the data object to a string with the format "key=value&key=value", which your server-side code can handle as an associative array.
Final thoughts
The 'Uncaught TypeError: Illegal invocation' error can be frustrating, but with the solutions provided above, you can overcome it and make your ajax request work smoothly. Remember to convert the data object to a string format using concatenation or serialize it with jQuery.param()
to pass it as an associative array to your server-side code.
If you found this article helpful or have any questions, feel free to leave a comment below. Happy coding!