Is there any way to change input type="date" format?
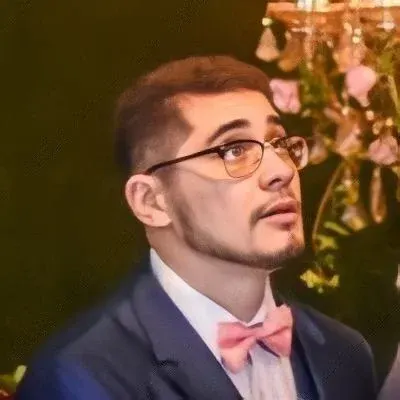
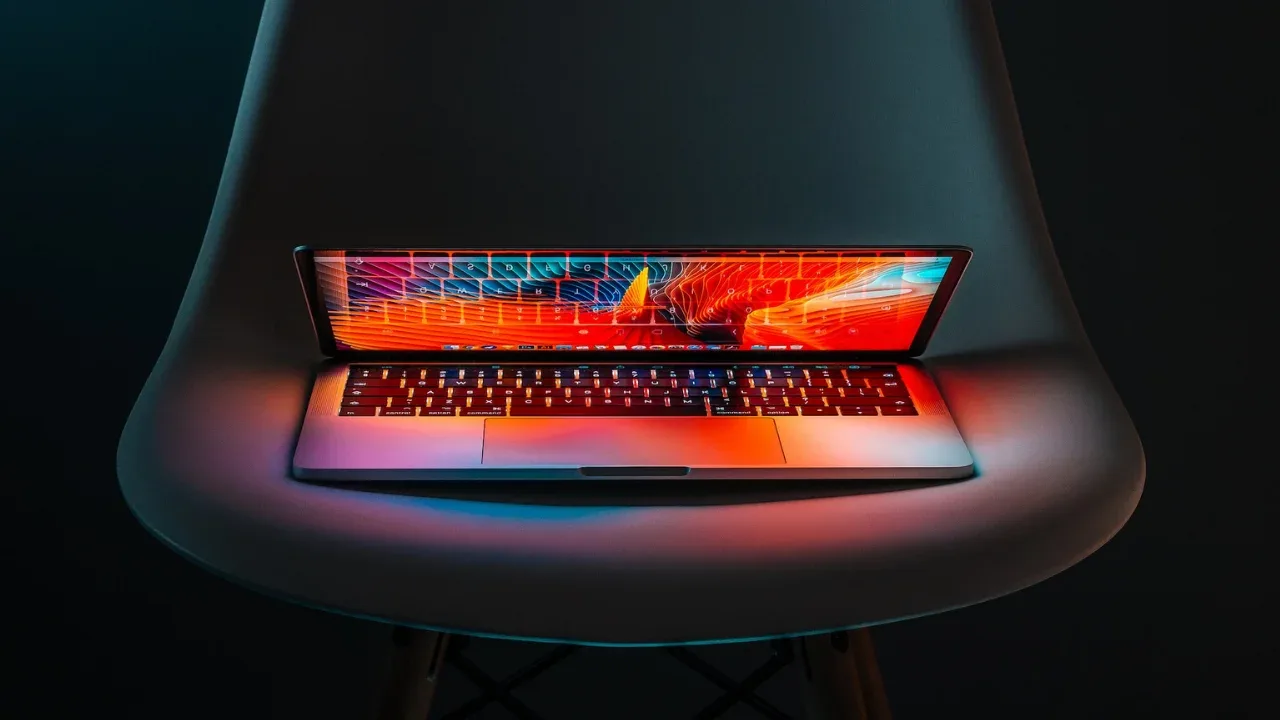
Is there any way to change input type="date" format? 📅
Have you ever felt frustrated when working with the input
element and trying to change the date format? Well, you're not alone! 🤷♀️
By default, the input
with type="date"
displays the date in the format YYYY-MM-DD
. But fear not! We have some tricks up our sleeves to help you personalize the format to your liking. 💪
The Problem 😫
The issue at hand revolves around wanting to display the inputted date in the format DD-MM-YYYY
instead of the default YYYY-MM-DD
. This can be a common requirement when dealing with user-facing applications where regional date formats are preferred.
Easy Solutions 🛠️
Luckily, there are a few solutions for changing the date format:
Using JavaScript to Modify Date Input 🖥️
One way to achieve this is by utilizing JavaScript to dynamically modify the date input. You can attach an
eventListener
to the date input and capture the inputted date. Then, using methods likesplit()
andjoin()
, you can rearrange the date elements accordingly.const dateInput = document.querySelector('input[type="date"]'); dateInput.addEventListener('change', (event) => { const inputtedDate = event.target.value; const [year, month, day] = inputtedDate.split('-'); const formattedDate = `${day}-${month}-${year}`; event.target.value = formattedDate; });
Using a Third-Party Date Picker Library 📆
Another approach is to make use of third-party date picker libraries that provide customizable date formats. Libraries like flatpickr or datepicker allow you to define custom formats and seamlessly integrate them into your code.
<link rel="stylesheet" href="path/to/datepicker.css"> <script src="path/to/datepicker.js"></script> <input type="text" class="datepicker"> <script> const dateInput = document.querySelector('.datepicker'); datepicker(dateInput, { dateFormat: 'dd-mm-yyyy', }); </script>
Using a JavaScript Framework like React or Angular ⚛️
If you're working with a JavaScript framework like React or Angular, there are often components and libraries available that enable you to easily manage and customize the format of the date input.
Conclusion 🎉
Changing the format of the input
with type="date"
doesn't have to be a daunting task anymore! With the solutions provided, you'll be able to embrace flexibility and personalize the date format to suit your needs.
Experiment with these solutions, and see which one fits your project best. Remember, coding is all about exploring and finding creative solutions!
Do you have any other tips or tricks for changing the date input format? Share them with us in the comments below! Let's make the web a more date-friendly place together. 😄✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
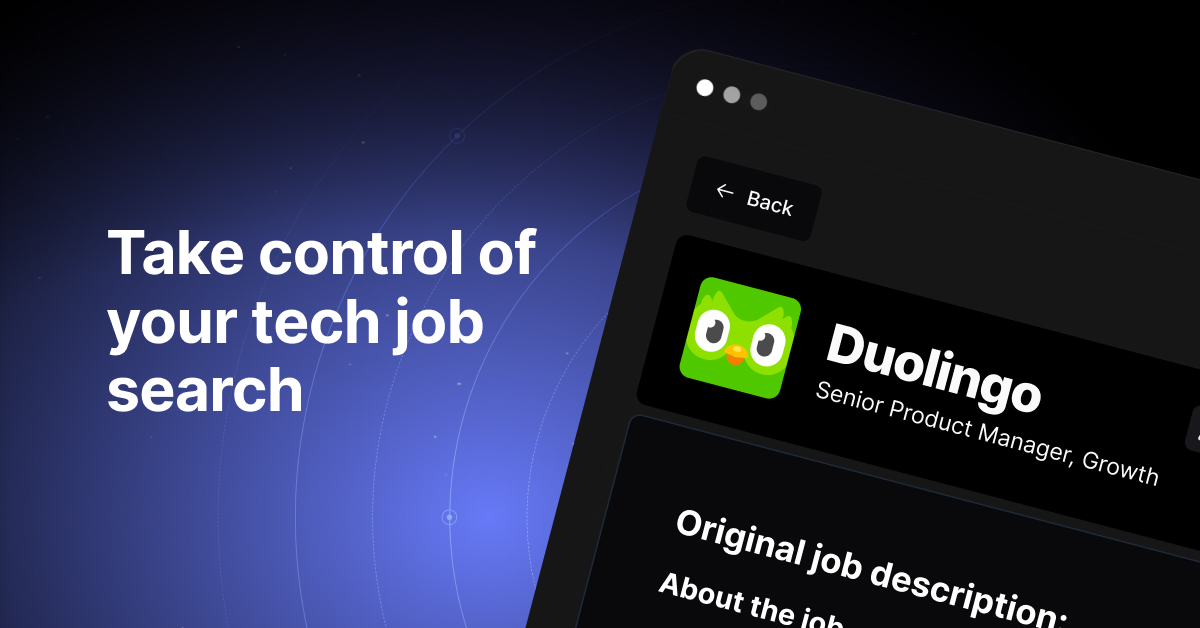