How to use radio buttons in ReactJS?
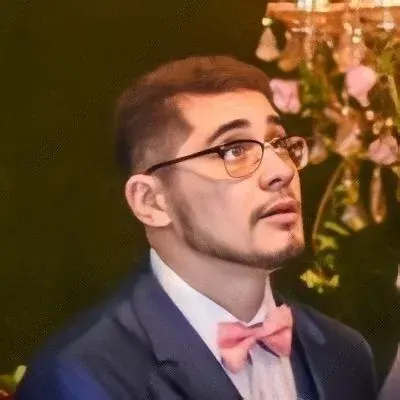
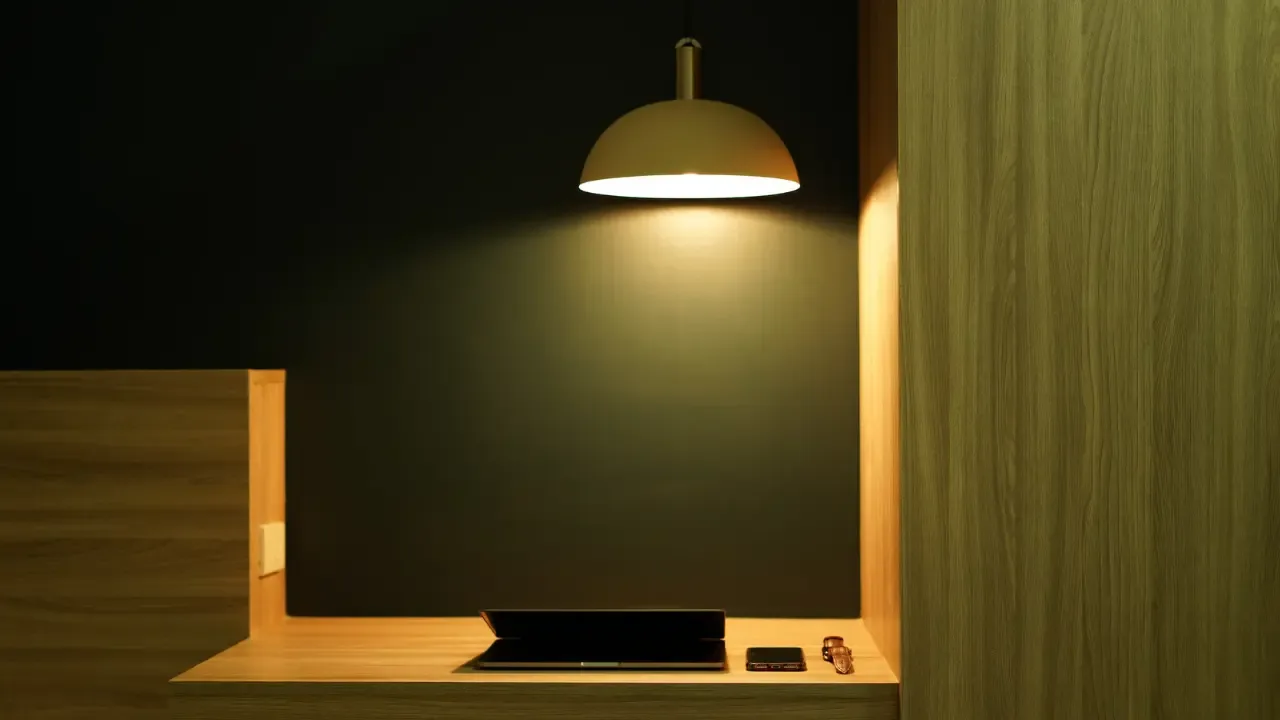
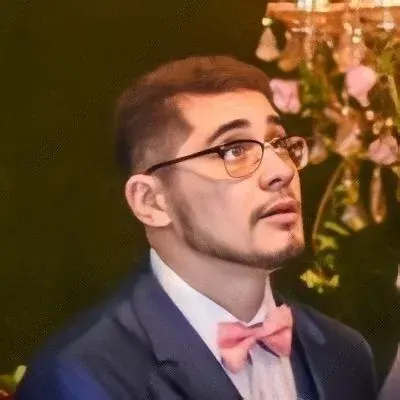
How to Use Radio Buttons in ReactJS: A Complete Guide
Are you new to ReactJS and wondering how to use radio buttons in your project? Don't worry, we've got you covered! In this tutorial, we'll walk you through the process step by step and provide easy solutions to common issues. So let's get started! ๐๐
Understanding the Problem
Let's start by understanding the problem at hand. The user has a component that generates multiple table rows based on the received data. Each row has two columns: site_name
and address
. The user needs to select one option from each column, and display the selected values in the footer of the table. Easy enough, right? ๐
The ReactJS Way to Handle Radio Buttons
In ReactJS, we handle input elements, including radio buttons, in a slightly different way compared to jQuery. Instead of using selectors, we leverage the power of React's state and event handling.
First, let's update the SearchResult
component to include a new state called selectedOptions
:
var SearchResult = React.createClass({
getInitialState: function() {
return {
selectedOptions: {
site_name: '',
address: ''
}
};
},
handleOptionChange: function(event) {
const { name, value } = event.target;
this.setState(prevState => ({
selectedOptions: {
...prevState.selectedOptions,
[name]: value
}
}));
},
render: function () {
// ...
}
});
In the code snippet above, we initialize the selectedOptions
state with empty values for site_name
and address
.
Next, we add an onChange
event listener to each radio button, which calls the handleOptionChange
method. This method updates the corresponding property in the selectedOptions
state whenever a radio button is selected or deselected.
var SearchResult = React.createClass({
// ...
render: function () {
var resultRows = this.props.data.map(function (result, index) {
return (
<tbody key={index}>
<tr>
<td>
<input
type="radio"
name="site_name"
value={result.SITE_NAME}
checked={result.SITE_NAME === this.state.selectedOptions.site_name}
onChange={this.handleOptionChange}
/>
{result.SITE_NAME}
</td>
<td>
<input
type="radio"
name="address"
value={result.ADDRESS}
checked={result.ADDRESS === this.state.selectedOptions.address}
onChange={this.handleOptionChange}
/>
{result.ADDRESS}
</td>
</tr>
</tbody>
);
});
// ...
}
});
In the code snippet above, we added the checked
prop to each radio button. This prop determines whether the radio button should be selected based on the value in the selectedOptions
state.
Displaying the Selected Options
Now that we are capturing the selected options in the component's state, let's display them in the footer of the table.
var SearchResult = React.createClass({
// ...
render: function () {
// ...
return (
<table className="table">
<thead>
<tr>
<th>Name</th>
<th>Address</th>
</tr>
</thead>
{resultRows}
<tfoot>
<tr>
<td>Selected site name: {this.state.selectedOptions.site_name}</td>
<td>Selected address: {this.state.selectedOptions.address}</td>
</tr>
</tfoot>
</table>
);
},
});
In the code snippet above, we utilize the selectedOptions
state to display the selected values in the footer of the table. We access the selected values using this.state.selectedOptions.site_name
and this.state.selectedOptions.address
.
That's It!
And that's how you use radio buttons in ReactJS! ๐๐
By following the steps outlined in this guide, you have learned how to handle radio buttons with ease and display the selected options using React's state management.
Feel free to experiment with this code in your own ReactJS project. ๐งชโจ
If you found this guide helpful, share it with your fellow React enthusiasts and let us know your thoughts in the comments below. We'd love to hear from you! โค๏ธ
Keep coding and happy Reacting! ๐ฉโ๐ป๐จโ๐ป