How to Right-align flex item?
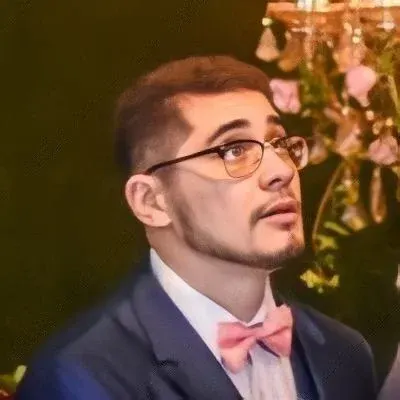
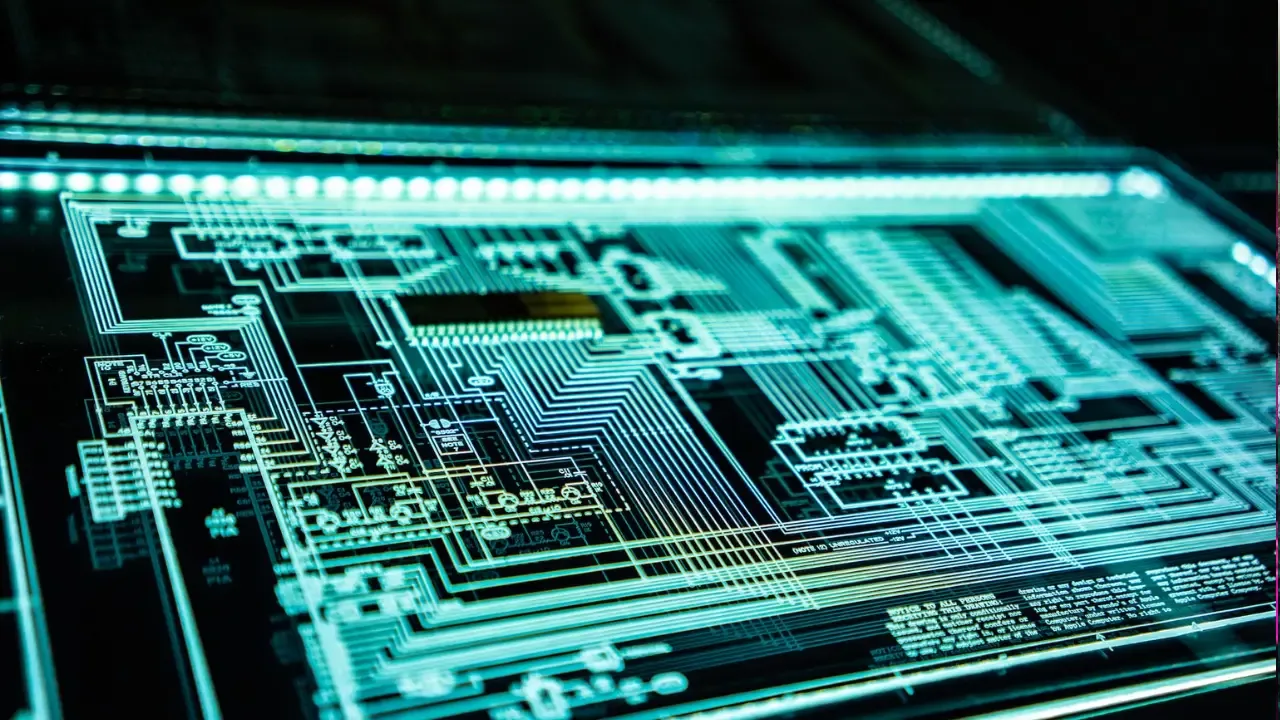
How to Right-align flex item?
You've probably come across a situation where you need to right-align a flex item in a flex container. It could be something like aligning a "Contact" button or any other element to the right side of the container. But the real question is, can we achieve this without using position: absolute
? The answer is yes! In this blog post, I'll show you how to do it in a more "flexboxish" way.
The Problem
Let's start by understanding the problem. In the given code snippet, we have a flex container with three flex items (div.a
, div.b
, div.c
). The div.b
is the one we want to center, while div.c
should be aligned to the right side of the container.
<h2>With title</h2>
<div class="main">
<div class="a"><a href="#">Home</a></div>
<div class="b"><a href="#">Some title centered</a></div>
<div class="c"><a href="#">Contact</a></div>
</div>
<h2>Without title</h2>
<div class="main">
<div class="a"><a href="#">Home</a></div>
<!-- <div class="b"><a href="#">Some title centered</a></div> -->
<div class="c"><a href="#">Contact</a></div>
</div>
The CSS applied to these elements is as follows:
.main {
display: flex;
}
.a,
.b,
.c {
background: #efefef;
border: 1px solid #999;
}
.b {
flex: 1;
text-align: center;
}
.c {
position: absolute;
right: 0;
}
As you can see, the div.c
is right-aligned using position: absolute
, but this solution is not very flexible and might cause issues in some cases. So let's find a better way!
The Solution
To right-align the flex item without using position: absolute
, we can make use of the margin-left: auto
trick. Here's how you can modify the code:
.c {
margin-left: auto;
}
With this simple addition to the div.c
class, the item will be automatically right-aligned within the flex container.
Code Snippet
To see the modified code in action, you can check out this JSFiddle.
Conclusion
Right-aligning a flex item doesn't have to involve complex positioning techniques. By using the margin-left: auto
trick, you can achieve the desired result in a more flexible and efficient way. Remember, flexbox is all about making our lives easier as developers!
So go ahead, give it a try, and let us know how it worked for you. Feel free to share your thoughts and any other tips you have in the comments section below. Happy coding!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
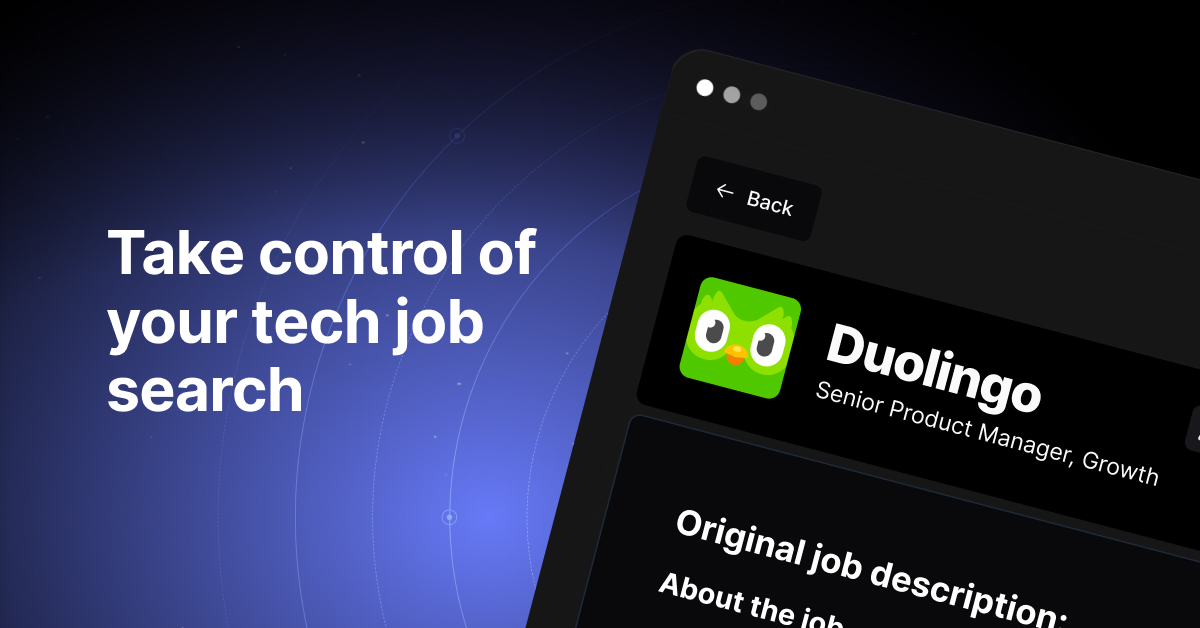