Angular 2 Checkbox Two Way Data Binding
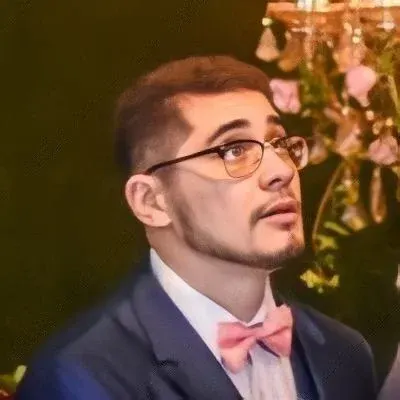
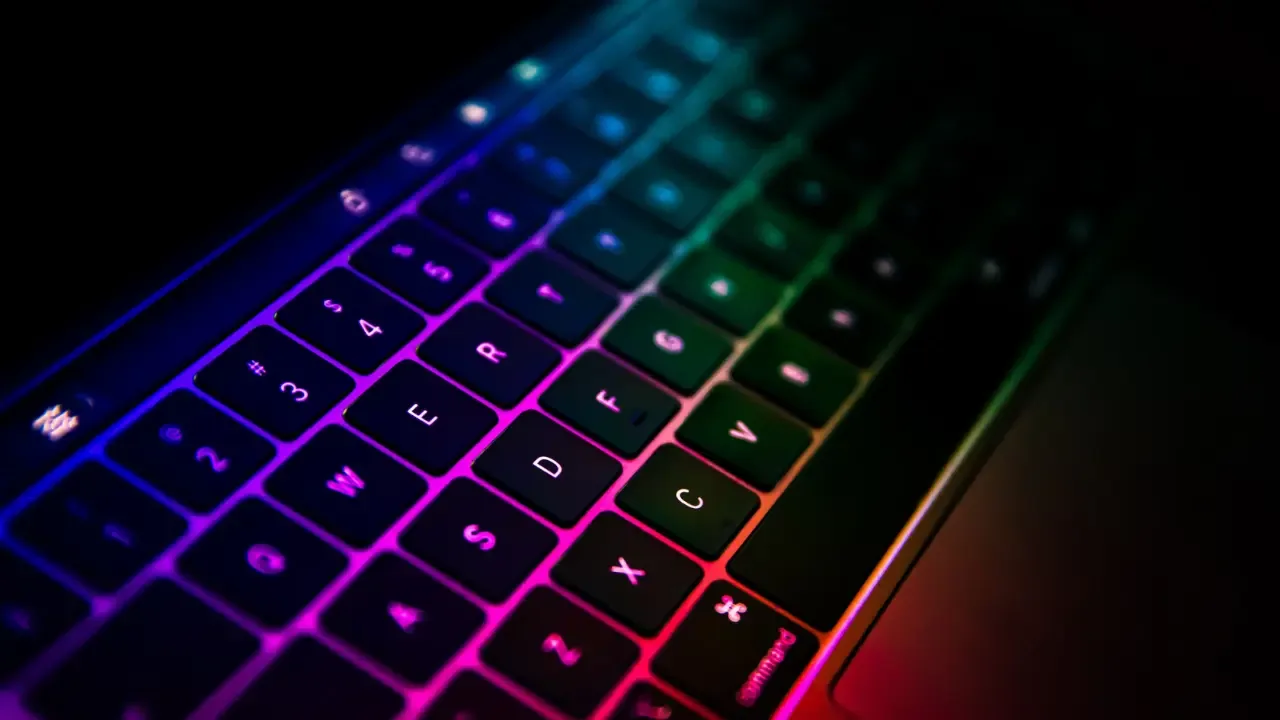
Angular 2 Checkbox Two Way Data Binding: A Complete Guide 📝💡
Are you new to Angular 2 and experiencing issues with two-way data binding for checkboxes in your login component? Don't worry, we've got you covered! 🤩
The Problem 🧐
The problem faced by our fellow Angular 2 developer is that the checkbox does not reflect changes made in the component's TypeScript. In other words, the checkbox does not "get" the new value when it is updated programmatically.
The Reason 🤔
The issue lies in the way two-way data binding is set up for the checkbox in the HTML code. Let's take a closer look at the relevant code snippet:
<div class="checkbox">
<label>
<input #saveUsername [(ngModel)]="saveUsername.selected" type="checkbox" data-toggle="toggle">Save username
</label>
</div>
The checkbox's value is bound to saveUsername.selected
using Angular's ngModel
directive. However, in the component's TypeScript, the value of saveUsername
is directly assigned as a boolean.
The Solution 🛠️
To make the checkbox reflect changes made to saveUsername
in the component, we need to update our code accordingly. Here's what you need to do:
Update the HTML code of the checkbox as follows:
<div class="checkbox">
<label>
<input #saveUsername [(ngModel)]="saveUsername" type="checkbox" data-toggle="toggle">Save username
</label>
</div>
In the TypeScript code of the
LoginComponent
, remove the.selected
property fromsaveUsername
.
export class LoginComponent implements OnInit {
private saveUsername: boolean = true;
// Rest of the code...
}
By removing the .selected
property, the checkbox will now be bound directly to the saveUsername
variable in the component.
Testing the Solution ✅
To ensure everything is working as expected, let's test the updated code. Open your browser's developer console and follow these steps:
Initially, the console should log
true
for bothsaveUsername
andautoLogin
. This is because theOnInit
method sets the values totrue
.When you click on the checkbox, the console should correctly log the updated value of
saveUsername
.To test updating the checkbox programmatically, open the developer console and execute the following command:
document.querySelector('input[type="checkbox"]').checked = false;
Now, check the console again. The value of saveUsername
should be false
, and the checkbox should reflect this change.
Wrapping Up 🎉
Congratulations! You've successfully resolved the issue with two-way data binding for checkboxes in your Angular 2 login component. 🙌
If you have any further questions or face any other challenges, feel free to reach out. As always, happy coding! 💻✨
Call-to-Action: Engage with Us! 📣💌
We hope this guide has been helpful in solving your Angular 2 checkbox data binding issue. If you found it useful, don't forget to share it with your fellow developers! Let them benefit from this solution too. 😊🚀
If you have any suggestions, questions, or other topics you'd like us to cover, leave a comment below. We love hearing from our readers and are always looking for ways to improve. Let's keep the conversation going! 💬👥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
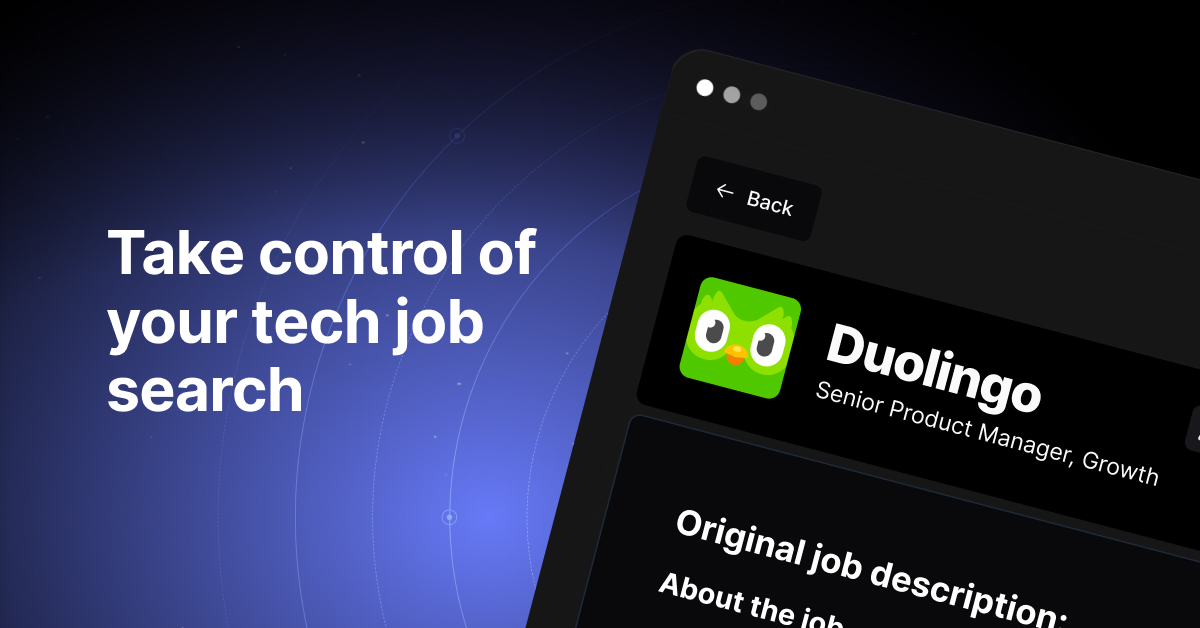