node.js hash string?
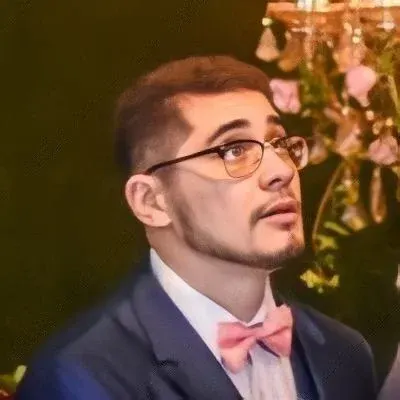
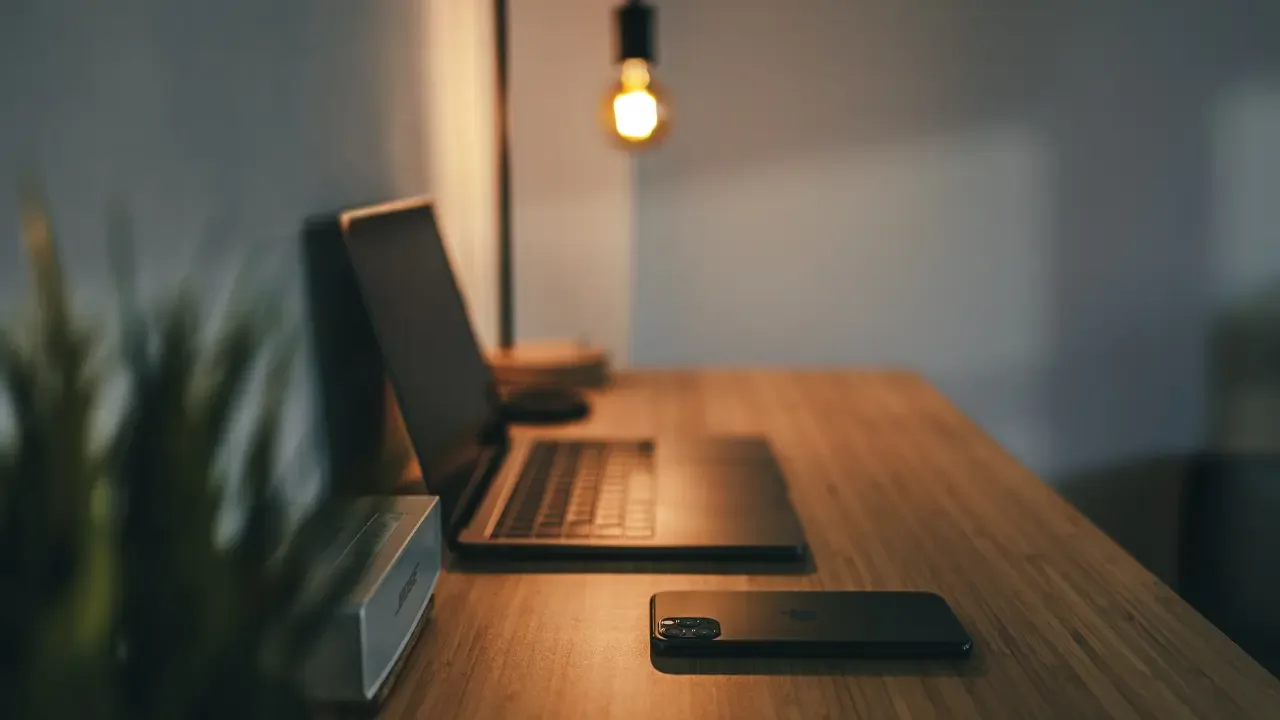
🔒🔍 Hashing Strings with Node.js: Versioning Made Easy! 🔍🔒
Have you ever wondered how to generate a hash for a string in Node.js? Well, fret no more! In this blog post, we'll explore the easiest and most efficient way to hash a string in Node.js, specifically for versioning purposes, not for security.
🔧 Problem: Generating the Hash So, you have a string that you want to hash for versioning. The big question is, what's the easiest way to make this happen in Node.js? Don't worry, we got you covered!
💡 Solution: Utilizing the 'crypto' Module The 'crypto' module in Node.js provides a straightforward and convenient way to generate a hash. Here's an example of a simple function that takes in a string and returns its hash:
const crypto = require('crypto');
function generateHash(data) {
const hash = crypto.createHash('sha256');
hash.update(data);
const hashedData = hash.digest('hex');
return hashedData;
}
const myString = 'Hello, World!';
const hashedString = generateHash(myString);
console.log(hashedString); // Output: cd9de5fe6175e6bc9ccf34443a457aeafeb23829e3de1ce9e19975ae34ed9fd9
Here, we utilize the createHash()
method to create a Hash object, specify the hashing algorithm (in this case, 'sha256'), and then use the update()
method to pass in the data we want to hash. Finally, the digest()
method returns the hashed data as a hexadecimal string.
📣 Call-to-Action: Engage with Us! That's it! Now you know how to generate a hash for versioning using Node.js. But wait, there's more! We'd love to hear from you. Share your thoughts, questions, or any other topic you'd like us to cover in the comments below.
Let's engage and learn from each other! 😄🚀
Don't forget to share this blog post with your fellow developers who might benefit from it. Happy versioning! 🎉🔢
Note: Remember, this hashing method is suitable for versioning, not for security purposes. If your use case involves sensitive data or authentication, you should research encryption methods or consult a security expert.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
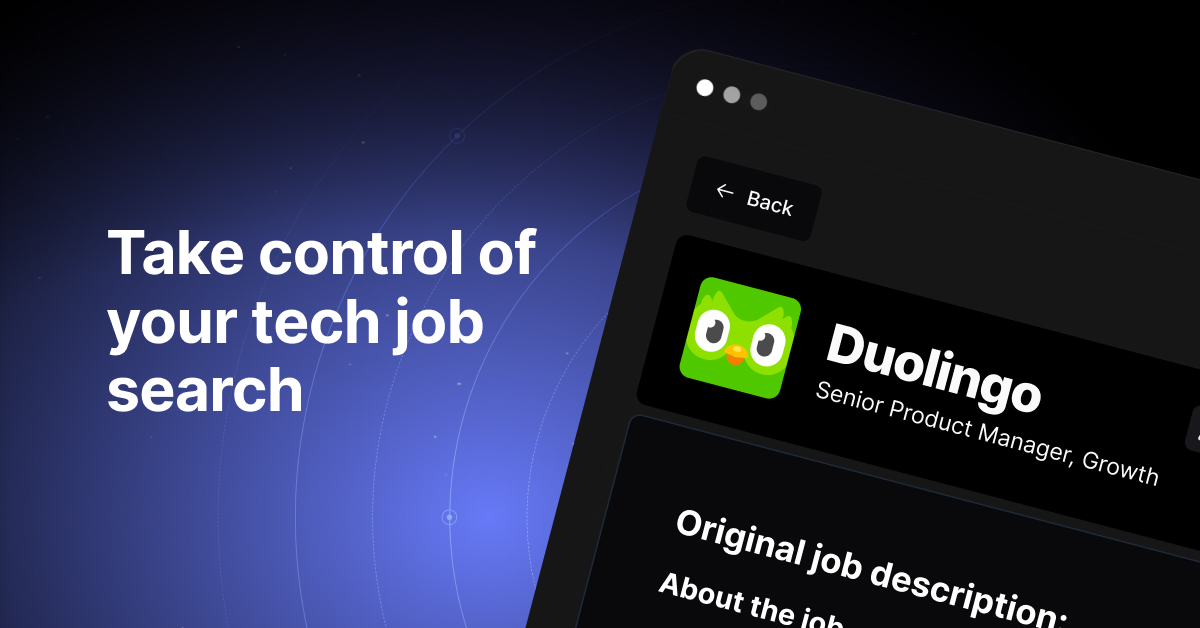