How to set Custom height for Widget in GridView in Flutter?
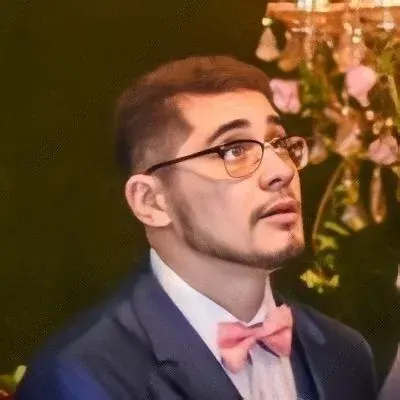
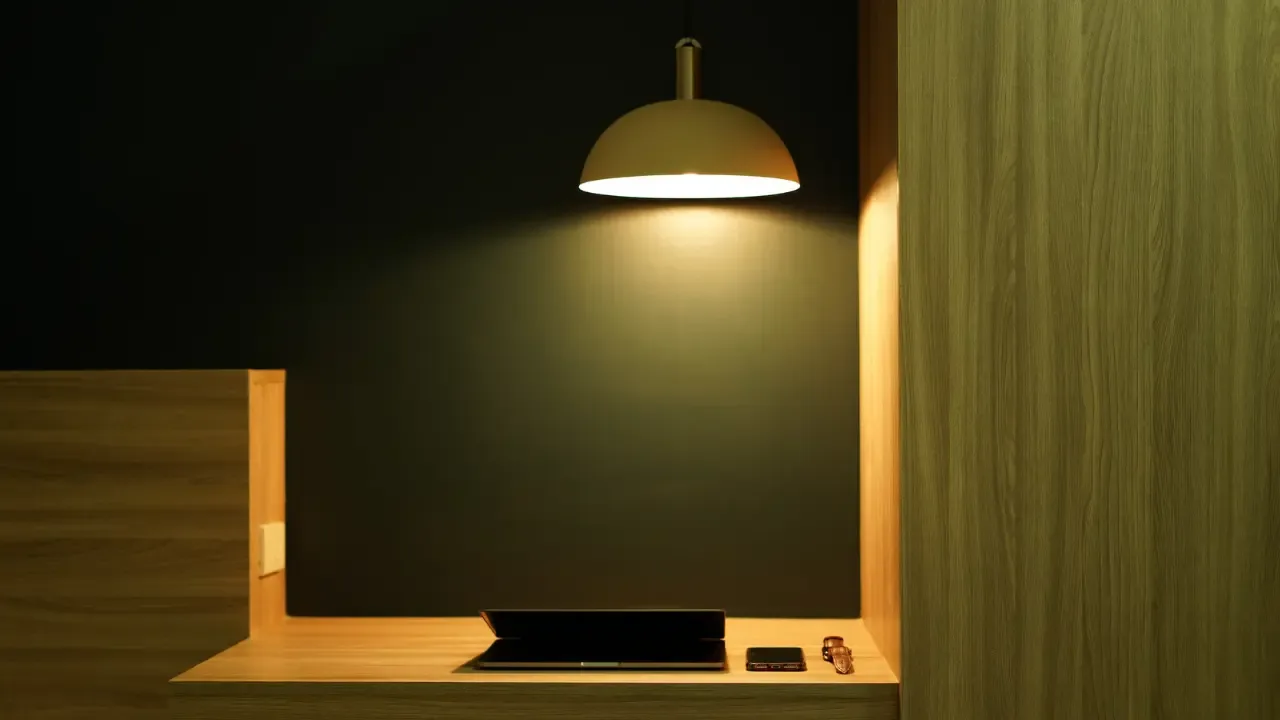
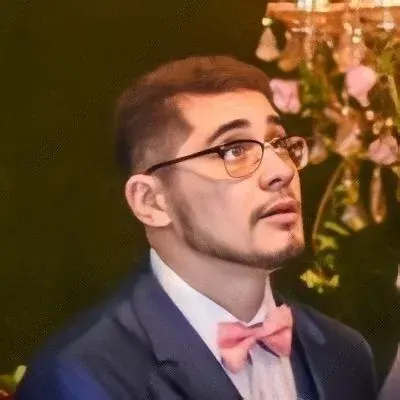
How to set Custom height for Widget in GridView in Flutter? 📏📏📏
So you want to customize the height of widgets in a GridView in Flutter, but no matter what you do, you end up with square widgets? Don't worry, you're not alone! Many developers face this issue when working with GridViews in Flutter. In this blog post, we'll explore the common problems and provide easy solutions to help you achieve the desired custom height for your widgets. Let's get started! 💪💪💪
The Problem 🤔🤔🤔
Let's take a look at the code snippet you provided:
class MyHomePage extends StatefulWidget {
// ...
}
class _MyHomePageState extends State<MyHomePage> {
List<String> widgetList = ['A', 'B', 'C'];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Container(
child: GridView.count(
crossAxisCount: 2,
controller: ScrollController(keepScrollOffset: false),
shrinkWrap: true,
scrollDirection: Axis.vertical,
children: widgetList.map((String value) {
return Container(
height: 250.0,
color: Colors.green,
margin: EdgeInsets.all(1.0),
child: Center(
child: Text(
value,
style: TextStyle(fontSize: 50.0, color: Colors.white),
),
),
);
}).toList(),
),
),
);
}
}
By setting the height
property of the Container
to 250.0
, you expected to get widgets with a custom height of 250.0
. However, the output you're getting is a GridView with square widgets. Not what you were aiming for, right? 😫😫😫
The Solution 👍👍👍
To achieve a custom height for your widgets in the GridView, you need to wrap your GridView.count
with an Expanded
widget. The Expanded
widget, when used inside a ListView or GridView, allows its child widget to occupy the remaining space in the scrollable area. By doing this, you ensure that the custom height you set for your widgets is respected. 🙌🙌🙌
Here's the updated code with the solution:
class MyHomePage extends StatefulWidget {
// ...
}
class _MyHomePageState extends State<MyHomePage> {
List<String> widgetList = ['A', 'B', 'C'];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Container(
child: Expanded(
child: GridView.count(
crossAxisCount: 2,
controller: ScrollController(keepScrollOffset: false),
shrinkWrap: true,
scrollDirection: Axis.vertical,
children: widgetList.map((String value) {
return Container(
height: 250.0,
color: Colors.green,
margin: EdgeInsets.all(1.0),
child: Center(
child: Text(
value,
style: TextStyle(fontSize: 50.0, color: Colors.white),
),
),
);
}).toList(),
),
),
),
);
}
}
By wrapping the GridView.count
with an Expanded
widget, you ensure that the custom height of 250.0
is applied to each widget in the GridView. Run the code now, and voila! You should see your desired output with custom height widgets in the GridView. 🎉🎉🎉
Conclusion 🎯🎯🎯
Setting a custom height for widgets in a GridView in Flutter is easily achievable by using the Expanded
widget. By following the solutions provided in this blog post, you can now customize the height of widgets in your GridViews with ease. Happy coding! 😊😊😊
Have you faced any other challenges with Flutter development? Let us know in the comments below, and we'll be happy to help you out! 🔥🔥🔥