Static vs class functions/variables in Swift classes?
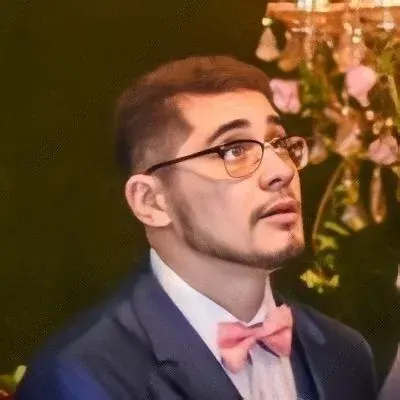
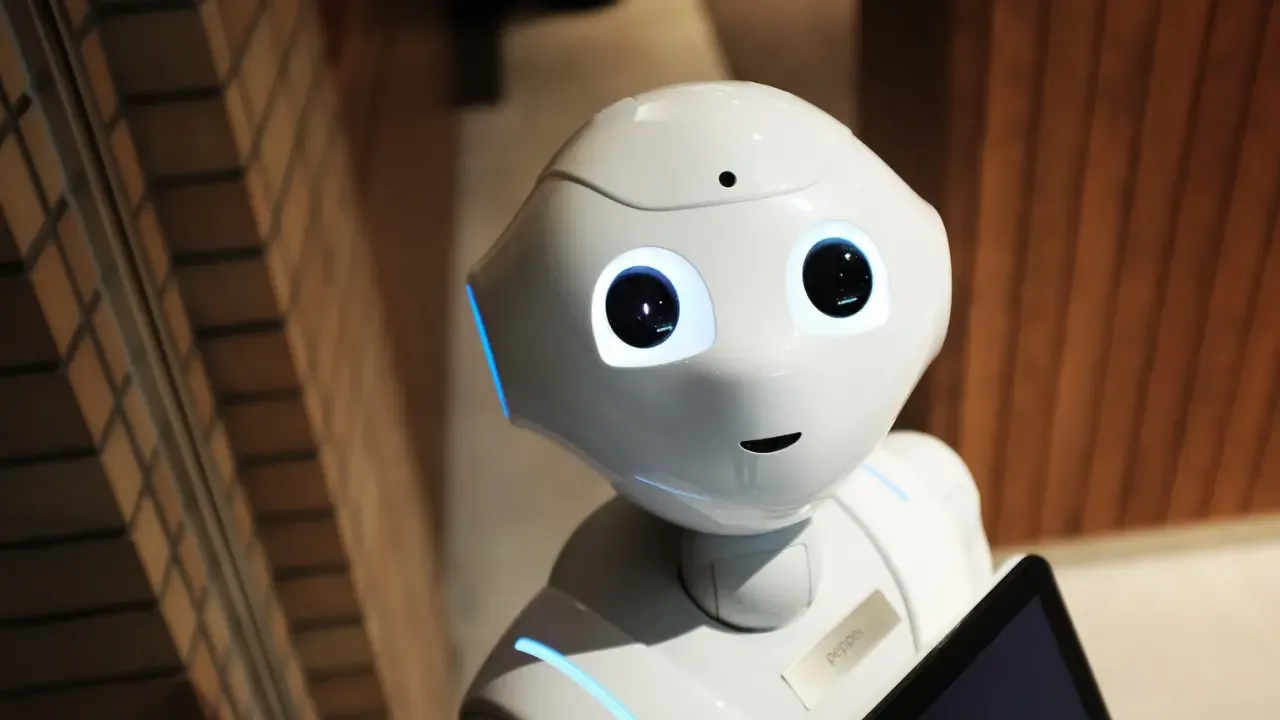
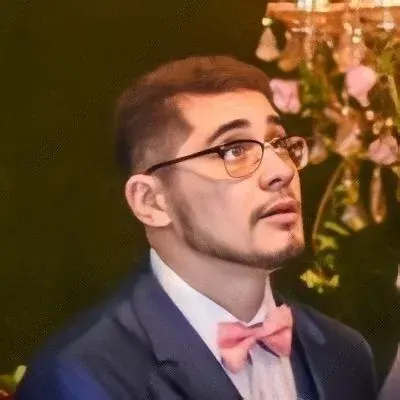
Static vs Class Functions/Variables in Swift Classes: What's the Difference and When to Use Them?
When working with Swift classes, you might come across two types of functions and variables: static and class. Although they may seem similar, there are subtle differences between the two. In this guide, we'll demystify these differences and help you understand when to use each type.
Static Functions and Variables
Let's first talk about static functions and variables. In the provided code snippet, myMethod1()
and myVar1
are both declared as static.
🔧 Common Issues: The main issue here is understanding what exactly a static function or variable does, and when it's appropriate to use them.
🍕 Easy Solution: In simple terms, a static function or variable is associated with the class itself rather than an instance of that class. This means you can use them without creating an instance of the class. Static members are also accessible using the class name followed by the function or variable name.
For example, in the code snippet, you can call myClass.myMethod1()
or access myClass.myVar1
directly, without needing to create an instance of myClass
.
💡 Example Explanation: Imagine you have a Car
class with a static variable maxSpeed
. This variable represents the maximum speed that any car can achieve. Since this value is the same for all instances of the Car
class, it makes sense to declare it as a static variable.
Static functions and variables are useful when you want to store or perform operations that are relevant to the class as a whole, rather than specific instances of the class.
✨ Compelling Call-to-Action: Next time you encounter a situation where a value or method applies to the entire class and not just specific instances, try using static functions and variables to keep your code clean and efficient. Give it a go and see the difference it makes!
Class Functions and Variables
Now, let's move on to class functions and variables. In the provided code snippet, myMethod2()
is declared as a class function.
🍕 Common Issues: Understanding when to use a class function instead of a static function can be confusing for some developers.
🔧 Easy Solution: Class functions, like static functions, are associated with the class itself rather than any specific instance. However, the main difference here is that class functions can be overridden by subclasses.
In the code snippet, if you were to subclass myClass
, you could override myMethod2()
in the subclass, providing custom functionality while still referencing the same function defined in the parent class.
💡 Example Explanation: Continuing with our Car
class example, let's say you have a class function called startEngine()
. When called, this function starts the engine of any car. However, subclasses of Car
might have different engine starting mechanisms. By declaring startEngine()
as a class function, you allow subclasses to provide their own implementations while maintaining the same overall structure and interface.
🔥 Compelling Call-to-Action: Next time you're designing a class hierarchy where some methods might have different behaviors across subclasses, consider using class functions. It helps foster modularity and flexibility in your codebase, making it easier to extend and maintain.
Static Variables vs Class Variables
Finally, let's briefly touch on the difference between static variables and class variables, even though the latter is not supported in the current version used in the provided code snippet.
🍕 Common Issues: The lack of support for class variables might raise questions about their potential use and how they differ from static variables.
🔧 Easy Solution: In the context of classes, both static variables and class variables are shared among all instances and the class itself. The main difference lies in how they handle inheritance.
When class variables are supported, the main distinction will be that subclasses can override class variables, whereas they cannot override static variables. This means that subclasses can provide their own values for class variables while still referencing the same variable defined in the parent class.
💡 Example Explanation: Let's suppose we have a Vehicle
class with a class variable numWheels
. This variable represents the number of wheels that all vehicles have. While most vehicles have four wheels, a subclass like Motorcycle
can override numWheels
and set it to two, reflecting the appropriate value for motorcycles.
🔥 Compelling Call-to-Action: Keep an eye out for future updates where class variables will be supported for use in Swift. When that time comes, leverage class variables to provide flexibility in your class hierarchies while maintaining a consistent interface across subclasses.
💬 Final Thoughts: Understanding when to use static functions and variables versus class functions and variables in Swift classes can greatly improve the structure and readability of your code. Static members are useful when the functionality or value is relevant to the class itself, while class members allow for customization and extension within subclasses. Stay tuned for updates on class variables in Swift, as they will add another layer of flexibility in your programming.
So, go ahead and embrace the power of static and class members in your Swift classes! Try implementing them in your next project and share your experiences with us. We'd love to hear how these concepts have improved your code quality and design. 🚀✨