How can I conditionally require form inputs with AngularJS?
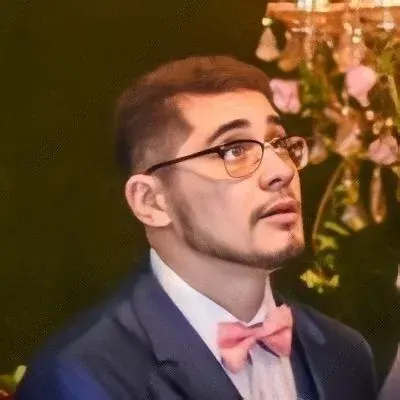
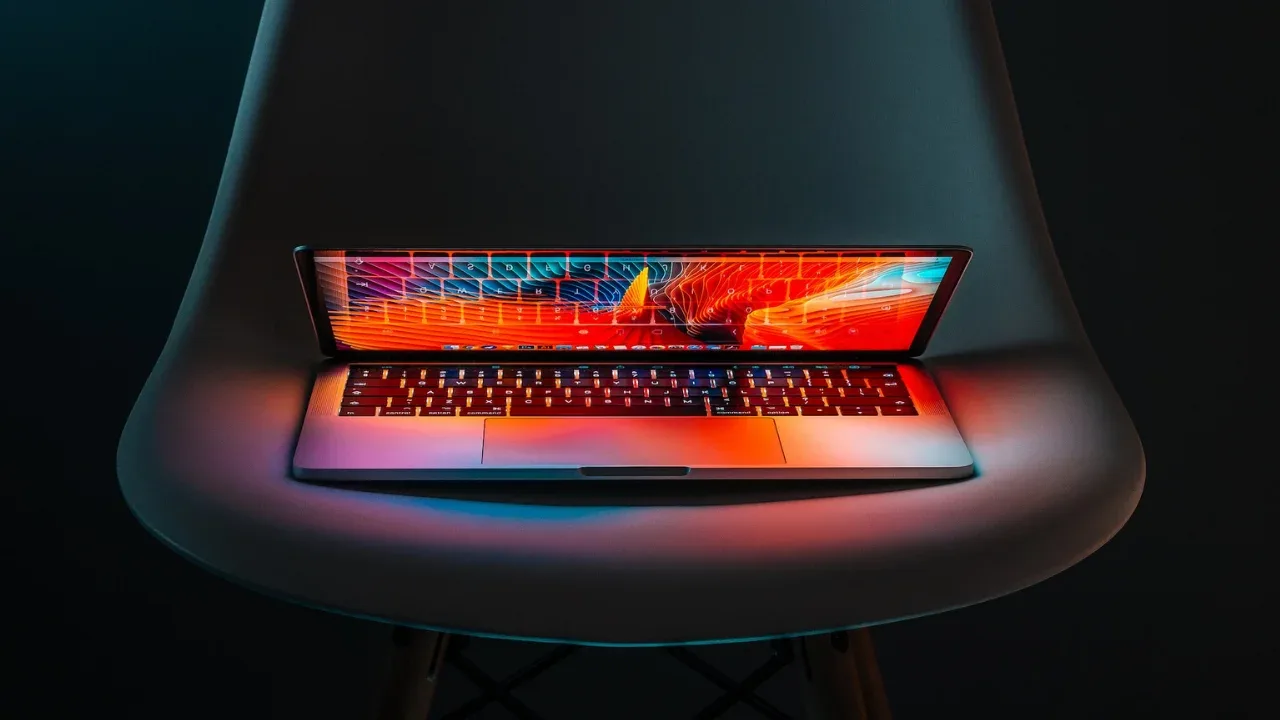
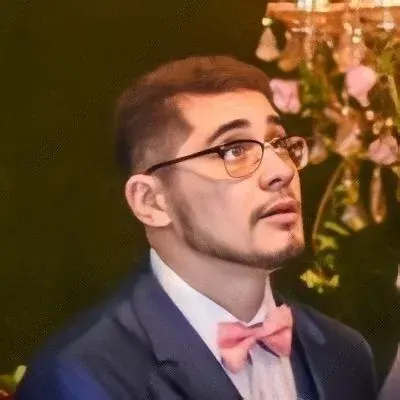
๐ Conditional Form Input Requirement in AngularJS: A Handy Guide ๐ค๐ค
Are you building an address book application with AngularJS and stuck on how to conditionally require form inputs? ๐ซ๐ญ Don't fret, we've got you covered! In this blog post, we'll unravel the mysteries behind this common issue and provide you with easy solutions. Let's dive in! ๐โโ๏ธ๐ฅ
The Challenge ๐คจ
Imagine you have a contact form with inputs for email and phone number. You want to require either the email or phone number field, but not both. If the phone number input is empty or invalid, the email input should be required, and vice versa. Sounds tricky, right? ๐ฅ
The AngularJS required
Directive โ
AngularJS provides a required
directive that allows you to specify if a form field is required or not. However, it might not be immediately clear how to use it in this specific case. ๐ค
The Solution ๐
Fortunately, a custom directive is not needed for this scenario. AngularJS offers a powerful feature called conditional validation that we can leverage. Here's how you can achieve it: โ๏ธ๐ง
In your form input fields, assign unique names to the email and phone number inputs, e.g.,
name="email"
andname="phone"
.In the
required
attribute of each input field, add a dynamic expression that checks if the other field is empty or invalid. For example, for the email input, you can userequired="!phone.$valid || !phone.$dirty"
. This expression requires the email input if the phone input is either invalid or not yet interacted with (dirty).!phone.$valid
checks if the phone input is invalid.!phone.$dirty
checks if the phone input has not been interacted with yet.
Repeat the same approach for the phone number input field. For example, use
required="!email.$valid || !email.$dirty"
.
Voila! ๐ By using these dynamic expressions within the required
attribute, you've successfully created the conditional form input requirement in AngularJS.
A Practical Example ๐ก๐
To help you grasp the concept better, let's take a closer look at the code snippet below:
<form name="contactForm">
<input type="email" name="email" required="!phone.$valid || !phone.$dirty">
<input type="tel" name="phone" required="!email.$valid || !email.$dirty">
<button type="submit">Save</button>
</form>
In this example, the email input will be required if the phone input is either invalid or not yet interacted with. Similarly, the phone input will be required if the email input is either invalid or not yet interacted with. Simple and effective! ๐ช๐
Engage with us! ๐ฃ๐
Learning AngularJS can be challenging, but we're here to support you every step of the way! ๐ฅ๐ก Have you encountered any other AngularJS hurdles while working on your project? Let us know in the comments section below! ๐ฏ๏ธ๐
Remember, sharing is caring! If you found this blog post helpful, share it with your fellow AngularJS enthusiasts to help them conquer their form validation woes. Together, we can level up our web development skills! ๐๐
Happy coding! ๐๐ป