Vertical viewport was given unbounded height
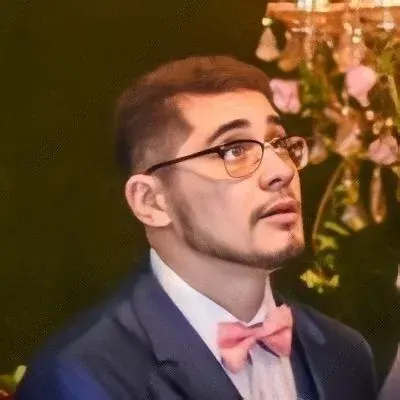
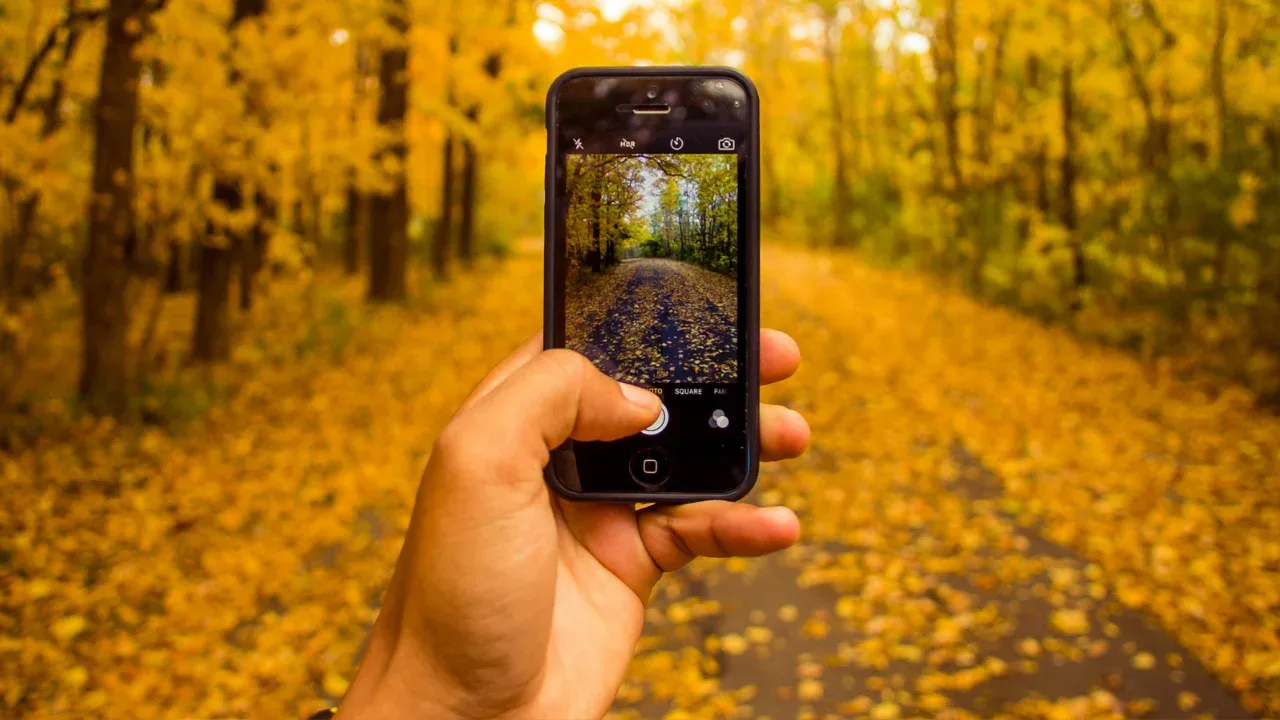
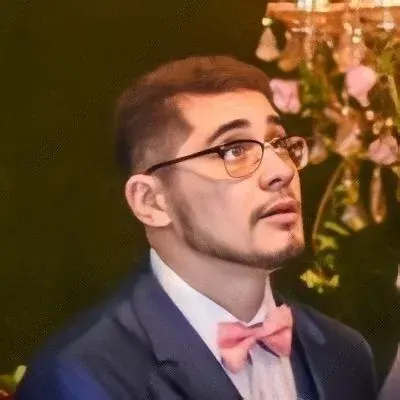
📚 Tech Blog: Easy Solutions for the "Vertical Viewport was given unbounded height" Issue 📚
Are you experiencing the dreaded "Vertical viewport was given unbounded height" error in your Flutter code? Don't worry, you're not alone! Many developers face this issue when working with scrollable widgets. But fear not, because in this blog post, we'll explain the common causes of this error and provide easy solutions to get your code back on track.
🔍 Understanding the Error
Let's start by understanding the error message itself. When you see the "Vertical viewport was given unbounded height" error, it means that there's an issue with the height configuration of a viewport widget in your code.
A viewport expands to fill its container in the scrolling direction, but in this case, a vertical viewport has been given an unlimited amount of vertical space to expand. This often happens when a scrollable widget is nested inside another scrollable widget.
🔎 Identifying the Problem
To identify the specific problem in your code, let's take a closer look at the code snippet you provided:
@override
Widget build(BuildContext context) {
return new Material(
color: Colors.deepPurpleAccent,
child: new Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
new GridView.count(
crossAxisCount: _column,
children: new List.generate(_row * _column, (index) {
return new Center(
child: new CellWidget(),
);
}),
),
],
),
);
}
Notice that you're using a GridView.count
widget inside a Column
widget. This combination can often lead to the "Vertical viewport was given unbounded height" error.
🤔 Easy Solutions
Now that we understand the problem, let's explore some easy solutions to solve it.
Solution 1: Use a
ListView
instead ofGridView
: If you don't specifically need a grid layout, consider changing theGridView.count
widget to aListView
widget. This change will ensure that the height is automatically adjusted based on the available content.Solution 2: Wrap the
GridView
with anExpanded
widget: If you really need a grid layout, you can wrap theGridView.count
widget with anExpanded
widget. This will enable theGridView
to take up all the available vertical space in theColumn
. Here's an example:new Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ new Expanded( child: new GridView.count( crossAxisCount: _column, children: new List.generate(_row * _column, (index) { return new Center( child: new CellWidget(), ); }), ), ), ], ),
Solution 3: Use a
SingleChildScrollView
: If you have a small number of grid items or you want to keep the current layout structure, consider wrapping theGridView
with aSingleChildScrollView
. This will give theGridView
the ability to scroll and accommodate its height based on the content.new Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ new SingleChildScrollView( child: new GridView.count( shrinkWrap: true, crossAxisCount: _column, children: new List.generate(_row * _column, (index) { return new Center( child: new CellWidget(), ); }), ), ), ], ),
🔥 Take Action!
Now that you have multiple solutions to the "Vertical viewport was given unbounded height" error, it's time to give them a try! Choose the solution that best suits your requirements and update your code accordingly.
If you found this blog post helpful, don't forget to share it with your fellow developers facing this issue. And if you have any questions or other topics you'd like us to cover, leave a comment below. Happy coding! 💻🚀