The argument type "Function" can"t be assigned to the parameter type "void Function()?" after null safety
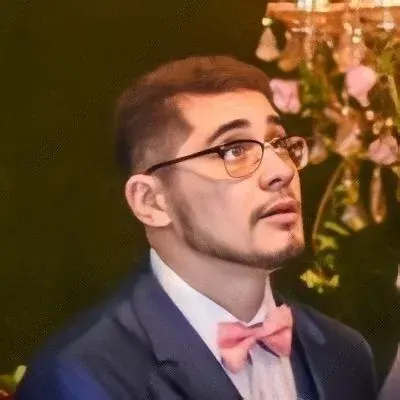
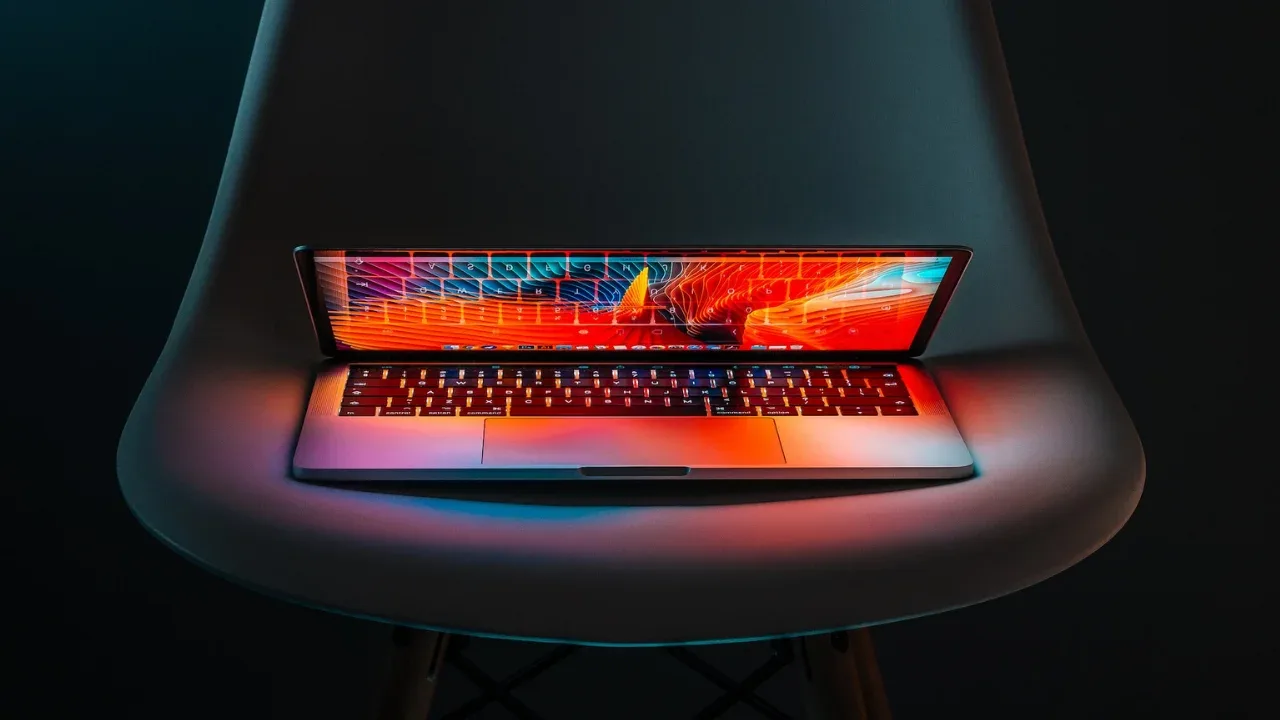
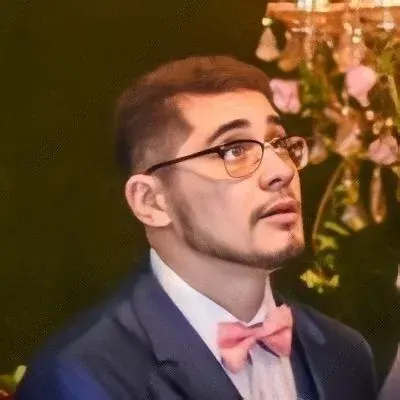
How to Solve the Argument Type Error in Flutter after Null Safety
Hey there fellow Flutter enthusiasts! 👋 Today we dive into a common issue that many of us face when working with Flutter after enabling null safety. 🤔 You may have encountered the following error while trying to assign a function to a callback parameter:
The argument type 'Function' can't be assigned to the parameter type 'void Function()'
In this blog post, we'll break down why this error occurs and provide you with easy solutions to fix it. So, let's get started! 💪
The Context
Before we dive into solving the issue, let's understand the context in which this error is occurring. In the given code snippet, we have a DrawerItem
class that represents an individual item in a drawer menu. It has two properties - text
to display the item label and onPressed
as a callback function when the button is pressed.
class DrawerItem extends StatelessWidget {
final String text;
final Function onPressed;
const DrawerItem({Key key, this.text, this.onPressed}) : super(key: key);
@override
Widget build(BuildContext context) {
return FlatButton(
child: Text(
text,
style: TextStyle(
fontWeight: FontWeight.w600,
fontSize: 18.0,
),
),
onPressed: onPressed,
);
}
}
The error is occurring on the onPressed
parameter, where we want to assign a function to be executed when the button is pressed. But what's causing this error? Let's find out! 🔍
Understanding the Error
The error message tells us that the argument type, which is Function
, cannot be assigned to the parameter type, which is 'void Function()'
. In Dart, 'void Function()'
represents a function that takes no arguments and returns nothing. So, why can't we assign a general Function
type to this specific type?
Well, the reason lies in the changes introduced with null safety. After enabling null safety, Dart becomes stricter with type checking, ensuring that nullability is properly handled.
The Solution
To resolve this error, we need to specify the correct type for the onPressed
parameter explicitly. Since onPressed
should be a function that takes no arguments and returns nothing, we can use the type VoidCallback
instead of the generic Function
type.
Let's update the code to reflect this change:
class DrawerItem extends StatelessWidget {
final String text;
final VoidCallback onPressed; // Use VoidCallback instead of Function
const DrawerItem({Key key, this.text, this.onPressed}) : super(key: key);
@override
Widget build(BuildContext context) {
return FlatButton(
child: Text(
text,
style: TextStyle(
fontWeight: FontWeight.w600,
fontSize: 18.0,
),
),
onPressed: onPressed,
);
}
}
By using VoidCallback
, we ensure that the assigned function matches the expected signature of taking no arguments and returning nothing.
Call to Action
Voila! 🎉 You have successfully resolved the argument type error and can now assign your desired function to the onPressed
parameter without any issues. Remember, when working with null safety, it's important to be explicit about the types to ensure safer and more reliable code.
If you found this blog post helpful, feel free to share it with your fellow Flutter developers. Also, don't hesitate to leave any comments or questions below. Happy Fluttering! 😄✨