setState() called after dispose()
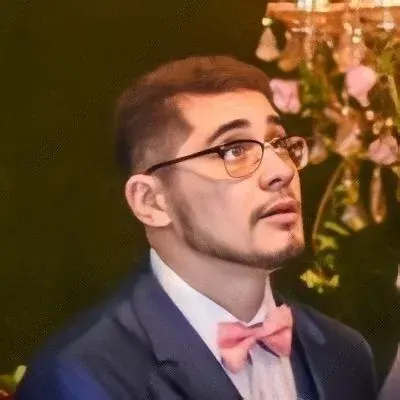
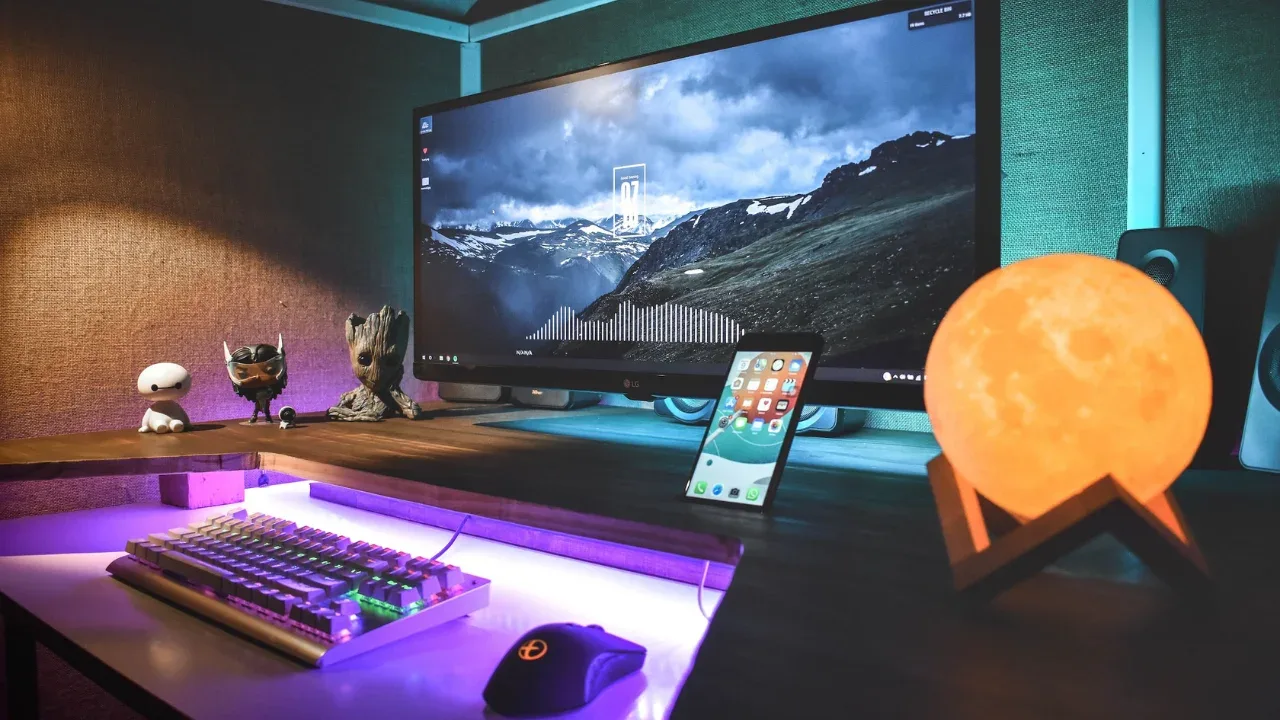
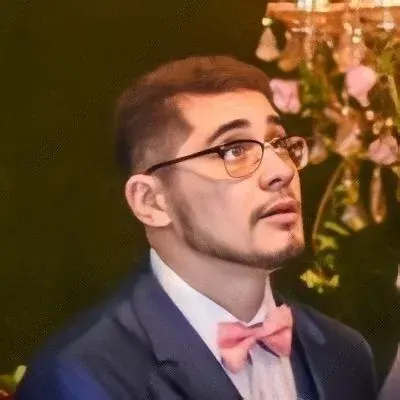
🕒 How to Fix the "setState() called after dispose()" Error in Flutter
Have you ever encountered the error "setState() called after dispose()" in your Flutter project? It can be quite frustrating, especially when you're not sure why it's happening. In this blog post, we'll explore common issues that can cause this error and provide easy solutions to fix it. Let's dive in! 💪
The Problem: setState() called after dispose()
When you see the error message "setState() called after dispose()", it generally means that you're trying to update the state of a widget that has been disposed of. Disposing of a widget typically happens when it's removed from the widget tree, such as when you navigate away from a screen or close a dialog.
In your case, the error occurs when you wait for 5 seconds and then confirm the selected time in the TimePicker. But why does Flutter try to update the state when you're not actively interacting with the widget? Let's find out! 🤔
The Example Code
Let's take a look at the example code provided to understand the context better:
class DateTimeButton extends State<DateTimeButtonWidget> {
DateTime selectedDate = new DateTime.now();
Future initTimePicker() async {
final TimeOfDay picked = await showTimePicker(
context: context,
initialTime: new TimeOfDay(hour: selectedDate.hour, minute: selectedDate.minute),
);
if (picked != null) {
setState(() {
selectedDate = new DateTime(selectedDate.year, selectedDate.month, selectedDate.day, picked.hour, picked.minute);
});
}
}
@override
Widget build(BuildContext context) {
return new RaisedButton(
child: new Text("${selectedDate.hour} ${selectedDate.minute}"),
onPressed: () {
initTimePicker();
}
);
}
}
This code defines a DateTimeButton
widget that displays the selected time and opens a TimePicker when pressed. The selected time is stored in the selectedDate
variable, and calling setState()
updates the widget's state.
The Possible Cause: Widgets with TickerProviderStateMixin
In your project, you mentioned that the issue seems to be related to the usage of TabBar
and TabBarView
, specifically with the mixin with TickerProviderStateMixin
. This mixin is required for animations and provides a ticker that triggers widgets to rebuild when needed.
The continuous refreshing and random state updates you observe might be due to the TabBar
and TabBarView
widgets constantly triggering state changes. However, let's explore possible solutions to mitigate this issue! 🛠️
The Solutions
To solve the "setState() called after dispose()" error, you can try one or more of the following solutions:
1. Cancel Timer Before Disposing
In your initTimePicker()
method, make sure to cancel any active timers before disposing of the widget. This ensures that setState()
is not called after the widget has been disposed. Here's an example:
@override
void dispose() {
_timer?.cancel(); // Cancel any active timers
super.dispose();
}
2. Use a StatefulWidget for the Parent Widget
If you're using a StatefulWidget
for the DateTimeButton
widget and experiencing the error, try converting the parent widget of DateTimeButton
into a StatefulWidget
as well. This can help manage the lifecycle of both widgets together and avoid any potential conflicts.
3. Wrap the Parent Widget with a Builder
Widget
Another solution is to wrap the parent widget containing the DateTimeButton
with a Builder
widget. The Builder
widget rebuilds its child whenever its builder
callback is called. This ensures that any state changes within the parent widget are handled correctly. Here's an example:
Widget build(BuildContext context) {
return Builder(
builder: (BuildContext context) {
// Your parent widget code here
},
);
}
📣 Call-to-Action: Share Your Experience and Solutions
Have you encountered the "setState() called after dispose()" error in your Flutter projects? How did you solve it? Share your experience and solutions in the comments below and help fellow developers overcome this issue!
If you found this blog post helpful, make sure to share it with your friends and colleagues who might be facing similar challenges. Happy coding! 😄🚀