Scaffold.of() called with a context that does not contain a Scaffold
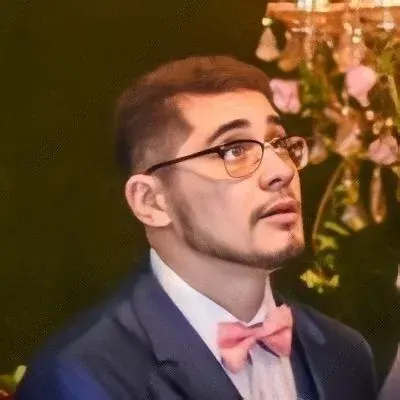
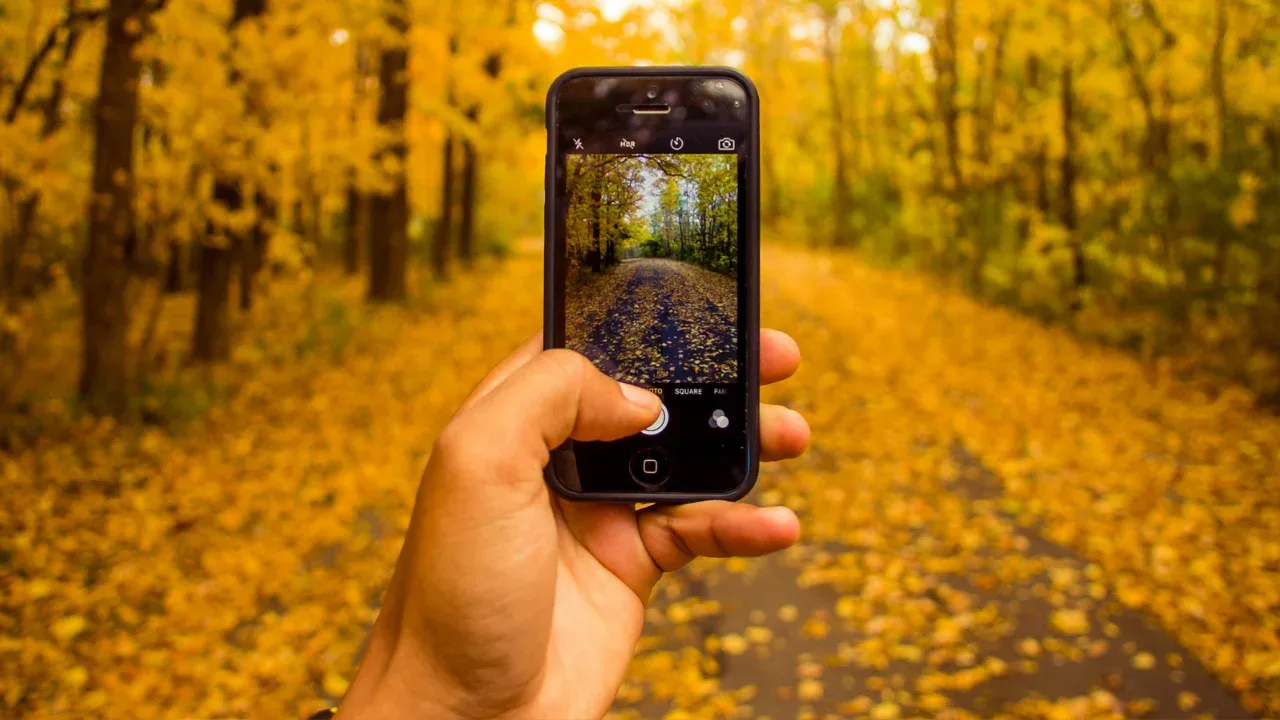
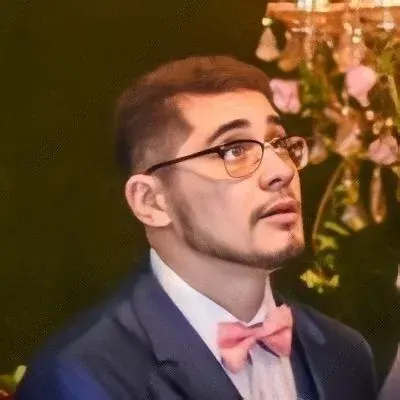
🚧 Avoiding the "Scaffold.of() called with a context that does not contain a Scaffold" Exception 🚧
Have you ever encountered the pesky "Scaffold.of() called with a context that does not contain a Scaffold" exception while working with Flutter? This common issue often occurs when you try to display a SnackBar
in your app's UI but encounter a context problem. But fear not! In this guide, we'll walk you through the problem, explain why it occurs, and provide you with easy solutions to overcome it. Let's dive in! 💪
😕 Understanding the Problem
The Scaffold.of()
method is a powerful tool in Flutter that allows you to access the nearest Scaffold
widget's state from any context. However, it requires a valid BuildContext
that contains a Scaffold
. In the provided code snippet, the error is triggered because the HomePage
widget does not have direct access to a Scaffold
, causing the exception to be thrown. This occurs because Scaffold.of(context)
is called outside the Scaffold
widget.
🛠️ Easy Solutions
Fortunately, there are several straightforward ways to solve this problem. Let's explore two of the most commonly used solutions.
1️⃣ Solution #1: Use a Builder Widget
One easy way to fix the issue is by using a Builder
widget. This widget provides a new BuildContext
that has access to the nearest Scaffold
widget. Here's how you can modify your code to prevent the exception:
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('SnackBar Playground'),
),
body: Builder(
builder: (context) => Center(
child: RaisedButton(
color: Colors.pink,
textColor: Colors.white,
onPressed: () => _displaySnackBar(context), // Use a function reference to prevent immediate invocation
child: Text('Display SnackBar'),
),
),
),
);
}
}
By wrapping the Center
widget with a Builder
widget, we introduce a new BuildContext
that contains a Scaffold
. This allows us to safely call Scaffold.of(context)
without encountering the exception.
2️⃣ Solution #2: Use a GlobalKey
Another solution involves using a GlobalKey<ScaffoldState>
to access the Scaffold
's state directly. This approach eliminates the need for a Builder
widget. Here's what your modified code would look like:
class HomePage extends StatefulWidget {
@override
_HomePageState createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
final GlobalKey<ScaffoldState> _scaffoldKey = GlobalKey<ScaffoldState>();
@override
Widget build(BuildContext context) {
return Scaffold(
key: _scaffoldKey, // Assign the GlobalKey<ScaffoldState> to the Scaffold
appBar: AppBar(
title: Text('SnackBar Playground'),
),
body: Center(
child: RaisedButton(
color: Colors.pink,
textColor: Colors.white,
onPressed: () => _displaySnackBar(),
child: Text('Display SnackBar'),
),
),
);
}
_displaySnackBar() {
final snackBar = SnackBar(content: Text('Are you talkin\' to me?'));
_scaffoldKey.currentState.showSnackBar(snackBar); // Access the Scaffold's state directly
}
}
By creating a GlobalKey<ScaffoldState>
and assigning it to the key
property of your Scaffold
, you can then call currentState.showSnackBar()
to display your SnackBar
without encountering the previous exception. Remember, this solution requires using a StatefulWidget
to hold the state and the GlobalKey
.
📣 Your Turn!
Now that you know how to solve the "Scaffold.of() called with a context that does not contain a Scaffold" exception, it's time to put your newfound knowledge into practice! Try fixing this issue in your Flutter app, and don't hesitate to share your experience and any additional solutions you discover.
Whether you choose to use the Builder
widget or go with the GlobalKey
approach, both solutions will help you overcome this pesky exception and have your app running smoothly.
If you found this guide helpful or have any questions, feel free to drop a comment below. Happy coding! ✌️