Null check operator used on a null value
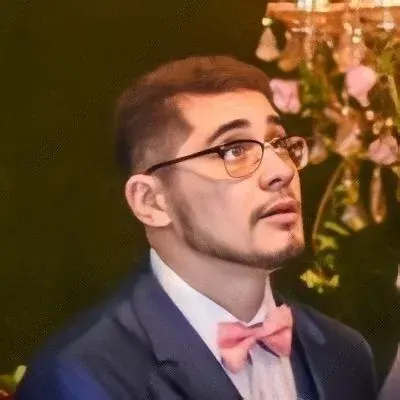
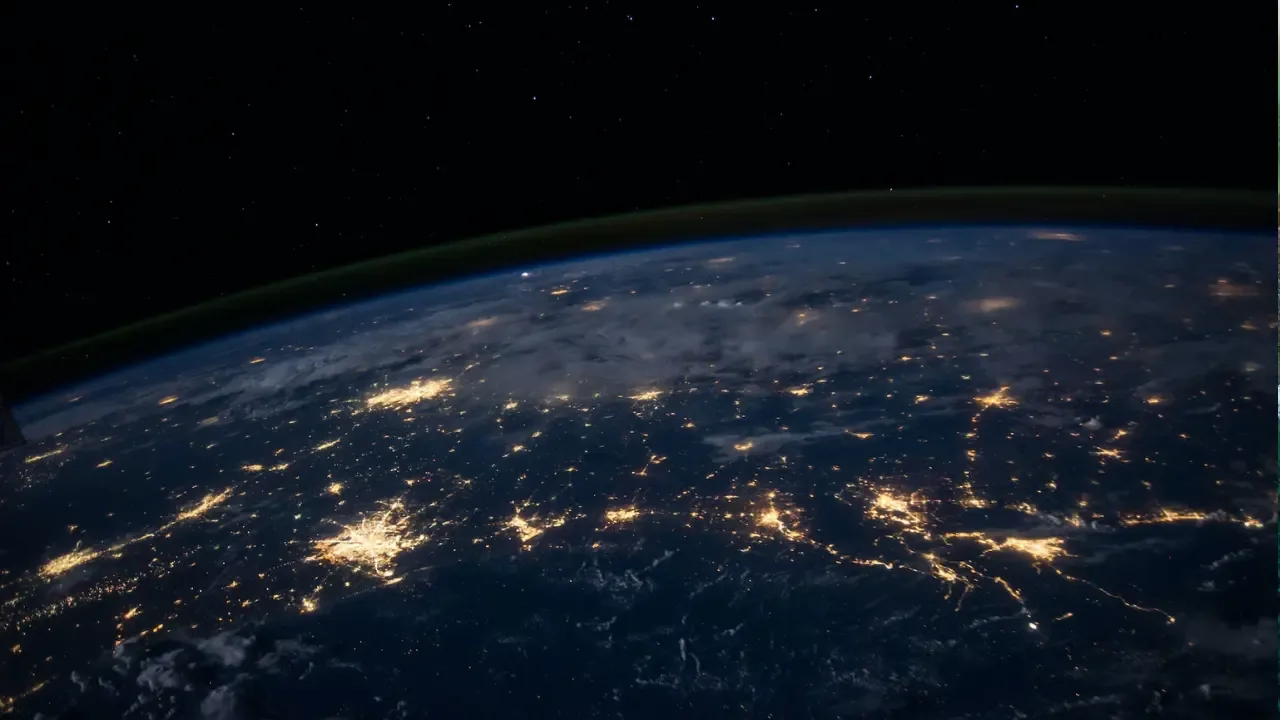
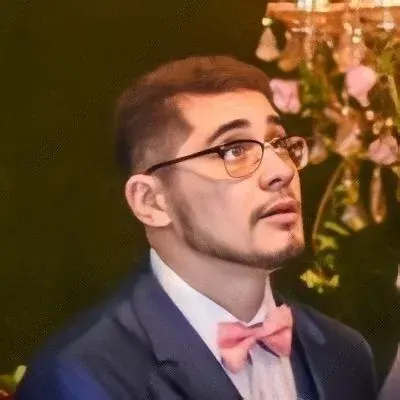
Null check operator used on a null value: Troubleshooting Guide
Are you a beginner in Flutter and encountered the error "Null check operator used on a null value" while running a simple Flutter app? Don't worry, you're not alone! In this guide, we will address common issues related to this error and provide you with easy solutions to fix it.
Understanding the Error
The error "Null check operator used on a null value" typically occurs when you try to access a property or call a method on a variable that is null. In Flutter, the null check operator (!
) is used to tell the compiler that a variable is not null. However, if you mistakenly use this operator on a null value, it will throw an exception.
Common Causes of the Error
Missing Initialization: One common cause of this error is when a variable is not properly initialized. For example, if you forget to assign a value to a variable before using it, it will be null by default, and using the null check operator on it will result in an error.
Incorrect Widget Composition: Another possible cause is incorrect widget composition. If you're trying to access a property of a widget that has not been instantiated correctly or is null, you will encounter this error.
Solutions
Now that we understand the causes, let's dive into some solutions to fix the "Null check operator used on a null value" error.
Solution 1: Check for Null before Using the Operator
The simplest solution is to check for null before using the null check operator (!
) on a variable. You can use conditional statements like if
or null-aware operators
to handle the null case.
if (myVariable != null) {
// Access properties or call methods on myVariable
}
or using null-aware operators:
// Access properties or call methods on myVariable if not null
myVariable?.propertyOrMethod();
By performing this null check, you ensure that the variable is not null before using the null check operator.
Solution 2: Properly Initialize Variables
Make sure that you initialize your variables properly before using them. Double-check your code to ensure that you assign an appropriate value to the variable before accessing its properties or methods.
In the code snippet you shared, the body
property of the Scaffold
widget is an empty container. If you intended to add child widgets to the body
, make sure to add them within the Container
or a different widget that suits your needs.
Solution 3: Check Widget Composition
If the error is related to widget composition, review your code to ensure that you are rendering the correct widget hierarchy. Check if you passed the correct widget to the home
property of MaterialApp
or any other widget that might be causing the issue.
Additional Tips
Debugging:
In Flutter, you can run in debug mode and use breakpoints to pause the execution at specific points in your code. This can help you identify the exact line of code that is causing the error and investigate the value of variables at that point.
Flutter Doctor:
The output you provided shows a warning regarding the Flutter extension not being installed in VS Code. Consider installing the Flutter extension to enhance your development experience and access helpful tools.
Conclusion
If you're new to Flutter and encountered the "Null check operator used on a null value" error, don't panic! By understanding the causes, following the provided solutions, and applying additional debugging techniques, you'll be able to resolve this issue and continue building your Flutter app.
Remember, debugging is an essential skill for any developer, so embrace these challenges as learning opportunities.🚀
Got any tips or tricks for dealing with this error? Share your experiences in the comments below! Let's help each other become better Flutter developers. 👇